walls.scad
Walls and structural elements that 3D print without support.
To use, add the following lines to the beginning of your file:
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
-
Section: Walls
-
sparse_wall()
– Makes an open cross-braced rectangular wall. [Geom] -
sparse_wall2d()
– Makes an open cross-braced rectangular wall. [Geom] -
corrugated_wall()
– Makes a corrugated rectangular wall. [Geom] -
thinning_wall()
– Makes a rectangular wall with a thin middle. [Geom] -
thinning_triangle()
– Makes a triangular wall with a thin middle. [Geom] -
narrowing_strut()
– Makes a strut like an extruded baseball home plate. [Geom]
-
Synopsis: Makes an open cross-braced rectangular wall. [Geom]
Topics: FDM Optimized, Walls
See Also: corrugated_wall(), thinning_wall(), thinning_triangle(), narrowing_strut()
See Also: corrugated_wall(), thinning_wall()
Usage:
- sparse_wall(h, l, thick, [maxang=], [strut=], [max_bridge=]) [ATTACHMENTS];
Description:
Makes an open rectangular strut with X-shaped cross-bracing, designed to reduce the need for support material in 3D printing.
Arguments:
By Position | What it does |
---|---|
h |
height of strut wall. |
l |
length of strut wall. |
thick |
thickness of strut wall. |
By Name | What it does |
---|---|
maxang |
maximum overhang angle of cross-braces. |
strut |
the width of the cross-braces. |
max_bridge |
maximum bridging distance between cross-braces. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Example 1: Typical Shape
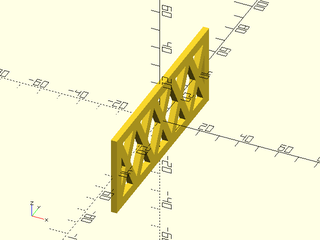
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
sparse_wall(h=40, l=100, thick=3);
Example 2: Thinner Strut
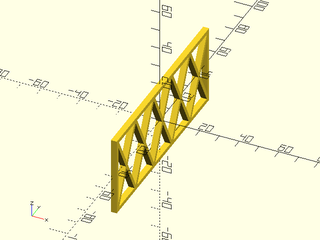
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
sparse_wall(h=40, l=100, thick=3, strut=2);
Example 3: Larger maxang
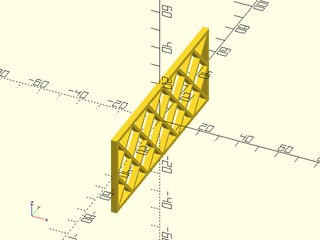
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
sparse_wall(h=40, l=100, thick=3, strut=2, maxang=45);
Example 4: Longer max_bridge
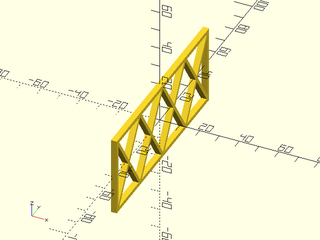
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
sparse_wall(h=40, l=100, thick=3, strut=2, maxang=45, max_bridge=30);
Synopsis: Makes an open cross-braced rectangular wall. [Geom]
Topics: FDM Optimized, Walls
See Also: sparse_wall(), corrugated_wall(), thinning_wall(), thinning_triangle(), narrowing_strut()
See Also: corrugated_wall(), thinning_wall()
Usage:
- sparse_wall2d(size, [maxang=], [strut=], [max_bridge=]) [ATTACHMENTS];
Description:
Makes a 2D open rectangular square with X-shaped cross-bracing, designed to be extruded, to make a strut that reduces the need for support material in 3D printing.
Arguments:
By Position | What it does |
---|---|
size |
The [X,Y] size of the outer rectangle. |
By Name | What it does |
---|---|
maxang |
maximum overhang angle of cross-braces. |
strut |
the width of the cross-braces. |
max_bridge |
maximum bridging distance between cross-braces. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
Example 1: Typical Shape
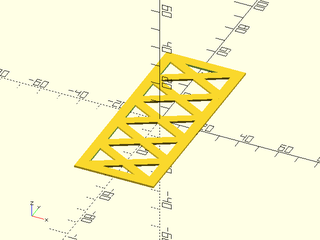
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
sparse_wall2d(size=[40,100]);
Example 2: Thinner Strut
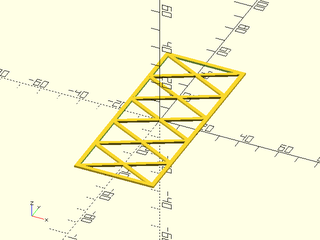
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
sparse_wall2d(size=[40,100], strut=2);
Example 3: Larger maxang
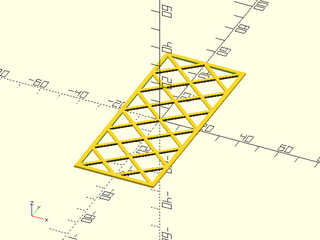
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
sparse_wall2d(size=[40,100], strut=2, maxang=45);
Example 4: Longer max_bridge
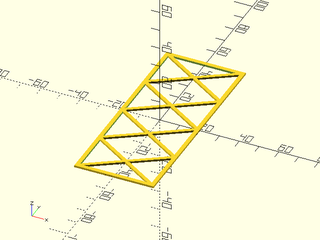
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
sparse_wall2d(size=[40,100], strut=2, maxang=45, max_bridge=30);
Synopsis: Makes a corrugated rectangular wall. [Geom]
Topics: FDM Optimized, Walls
See Also: sparse_wall(), thinning_wall(), thinning_triangle(), narrowing_strut()
See Also: sparse_wall(), thinning_wall()
Usage:
- corrugated_wall(h, l, thick, [strut=], [wall=]) [ATTACHMENTS];
Description:
Makes a corrugated wall which relieves contraction stress while still providing support strength. Designed with 3D printing in mind.
Arguments:
By Position | What it does |
---|---|
h |
height of strut wall. |
l |
length of strut wall. |
thick |
thickness of strut wall. |
By Name | What it does |
---|---|
strut |
the width of the cross-braces. |
wall |
thickness of corrugations. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Example 1: Typical Shape
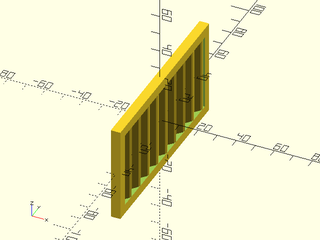
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
corrugated_wall(h=50, l=100);
Example 2: Wider Strut
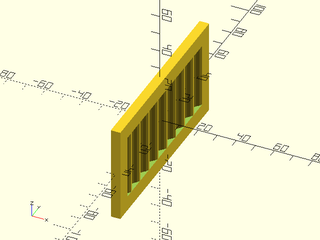
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
corrugated_wall(h=50, l=100, strut=8);
Example 3: Thicker Wall
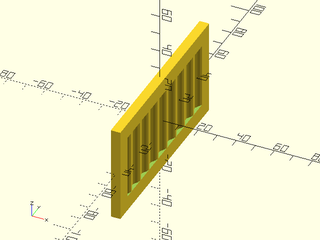
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
corrugated_wall(h=50, l=100, strut=8, wall=3);
Synopsis: Makes a rectangular wall with a thin middle. [Geom]
Topics: FDM Optimized, Walls
See Also: sparse_wall(), corrugated_wall(), thinning_triangle(), narrowing_strut()
See Also: sparse_wall(), corrugated_wall(), thinning_triangle()
Usage:
- thinning_wall(h, l, thick, [ang=], [braces=], [strut=], [wall=]) [ATTACHMENTS];
Description:
Makes a rectangular wall which thins to a smaller width in the center, with angled supports to prevent critical overhangs.
Arguments:
By Position | What it does |
---|---|
h |
Height of wall. |
l |
Length of wall. If given as a vector of two numbers, specifies bottom and top lengths, respectively. |
thick |
Thickness of wall. |
By Name | What it does |
---|---|
ang |
Maximum overhang angle of diagonal brace. |
braces |
If true, adds diagonal crossbraces for strength. |
strut |
The width of the borders and diagonal braces. Default: thick/2
|
wall |
The thickness of the thinned portion of the wall. Default: thick/2
|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Example 1: Typical Shape
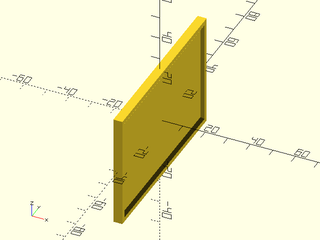
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
thinning_wall(h=50, l=80, thick=4);
Example 2: Trapezoidal
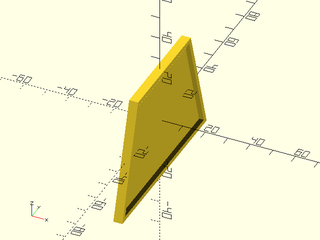
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
thinning_wall(h=50, l=[80,50], thick=4);
Example 3: Trapezoidal with Braces
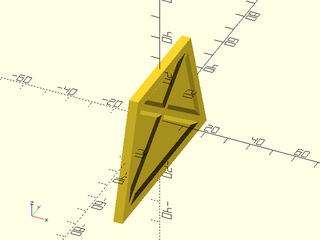
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
thinning_wall(h=50, l=[80,50], thick=4, strut=4, wall=2, braces=true);
Synopsis: Makes a triangular wall with a thin middle. [Geom]
Topics: FDM Optimized, Walls
See Also: sparse_wall(), corrugated_wall(), thinning_wall(), narrowing_strut()
See Also: thinning_wall()
Usage:
- thinning_triangle(h, l, thick, [ang=], [strut=], [wall=], [diagonly=], [center=]) [ATTACHMENTS];
Description:
Makes a triangular wall with thick edges, which thins to a smaller width in the center, with angled supports to prevent critical overhangs.
Arguments:
By Position | What it does |
---|---|
h |
height of wall. |
l |
length of wall. |
thick |
thickness of wall. |
By Name | What it does |
---|---|
ang |
maximum overhang angle of diagonal brace. |
strut |
the width of the diagonal brace. |
wall |
the thickness of the thinned portion of the wall. |
diagonly |
boolean, which denotes only the diagonal side (hypotenuse) should be thick. |
center |
If true, centers shape. If false, overrides anchor with UP+BACK . |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Example 1: Centered
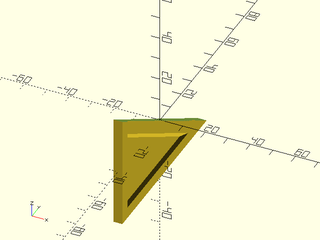
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
thinning_triangle(h=50, l=80, thick=4, ang=30, strut=5, wall=2, center=true);
Example 2: All Braces
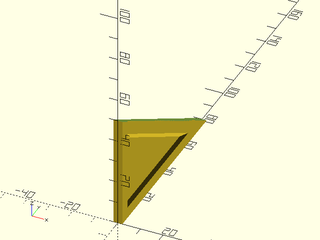
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
thinning_triangle(h=50, l=80, thick=4, ang=30, strut=5, wall=2, center=false);
Example 3: Diagonal Brace Only
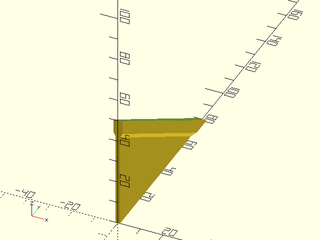
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
thinning_triangle(h=50, l=80, thick=4, ang=30, strut=5, wall=2, diagonly=true, center=false);
Synopsis: Makes a strut like an extruded baseball home plate. [Geom]
Topics: FDM Optimized
See Also: sparse_wall(), corrugated_wall(), thinning_wall(), thinning_triangle()
Usage:
- narrowing_strut(w, l, wall, [ang=]) [ATTACHMENTS];
Description:
Makes a rectangular strut with the top side narrowing in a triangle. The shape created may be likened to an extruded home plate from baseball. This is useful for constructing parts that minimize the need to support overhangs.
Arguments:
By Position | What it does |
---|---|
w |
Width (thickness) of the strut. |
l |
Length of the strut. |
wall |
height of rectangular portion of the strut. |
By Name | What it does |
---|---|
ang |
angle that the trianglar side will converge at. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Example 1:
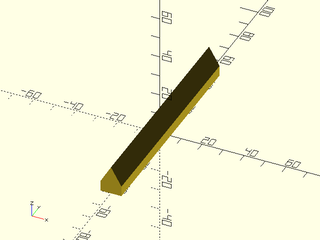
include <BOSL2/std.scad>
include <BOSL2/walls.scad>
narrowing_strut(w=10, l=100, wall=5, ang=30);
Table of Contents
Function Index
Topics Index
Cheat Sheet
Tutorials
Basic Modeling:
- constants.scad STD
- transforms.scad STD
- attachments.scad STD
- shapes2d.scad STD
- shapes3d.scad STD
- drawing.scad STD
- masks2d.scad STD
- masks3d.scad STD
- distributors.scad STD
- color.scad STD
- partitions.scad STD
- mutators.scad STD
Advanced Modeling:
Math:
- math.scad STD
- linalg.scad STD
- vectors.scad STD
- coords.scad STD
- geometry.scad STD
- trigonometry.scad STD
Data Management:
- version.scad STD
- comparisons.scad STD
- lists.scad STD
- utility.scad STD
- strings.scad STD
- structs.scad
- fnliterals.scad
Threaded Parts:
Parts:
- ball_bearings.scad
- cubetruss.scad
- gears.scad
- hinges.scad
- joiners.scad
- linear_bearings.scad
- modular_hose.scad
- nema_steppers.scad
- polyhedra.scad
- sliders.scad
- tripod_mounts.scad
- walls.scad
- wiring.scad
STD = Included in std.scad