cubetruss.scad
Parts for making modular open-frame cross-braced trusses and connectors.
To use, add the following lines to the beginning of your file:
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
-
-
cubetruss()
– Creates a multi-cube straight cubetruss shape. [Geom] -
cubetruss_corner()
– Creates a multi-cube corner cubetruss shape. [Geom] -
cubetruss_support()
– Creates a cubetruss support structure shape. [Geom]
-
-
-
cubetruss_foot()
– Creates a foot that can connect two cubetrusses. [Geom] -
cubetruss_joiner()
– Creates a joiner that can connect two cubetrusses end-to-end. [Geom] -
cubetruss_uclip()
– Creates a joiner that can connect two cubetrusses end-to-end. [Geom]
-
-
-
cubetruss_segment()
– Creates a single cubetruss cube. [Geom] -
cubetruss_clip()
– Creates a clip for the end of a cubetruss to snap-lock it to another cubetruss. [Geom] -
cubetruss_dist()
– Returns the length of a cubetruss truss.
-
Synopsis: Creates a multi-cube straight cubetruss shape. [Geom]
Topics: Trusses, CubeTruss, FDM Optimized, Parts
See Also: cubetruss_segment(), cubetruss_support(), cubetruss_corner()
Usage:
- cubetruss(extents, [clips=], [bracing=], [size=], [strut=], [clipthick=], ...) [ATTACHMENTS];
Description:
Creates a cubetruss truss, assembled out of one or more cubical segments.
Arguments:
By Position | What it does |
---|---|
extents |
The number of cubes in length to make the truss. If given as a [X,Y,Z] vector, specifies the number of cubes in each dimension. |
clips |
List of vectors pointing towards the sides to add clips to. |
bracing |
If true, adds internal cross-braces. Default: $cubetruss_bracing (usually true) |
size |
The length of each side of the cubetruss cubes. Default: $cubetruss_size (usually 30) |
strut |
The width of the struts on the cubetruss cubes. Default: $cubetruss_strut_size (usually 3) |
clipthick |
The thickness of the clips. Default: $cubetruss_clip_thickness (usually 1.6) |
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis. See spin. Default: 0
|
orient |
Vector to rotate top towards. See orient. Default: UP
|
Example 1:
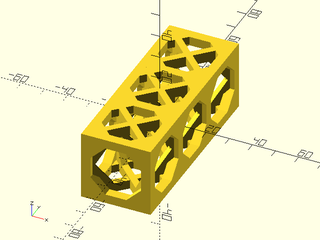
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss(extents=3);
Example 2:
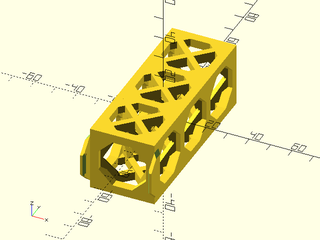
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss(extents=3, clips=FRONT);
Example 3:
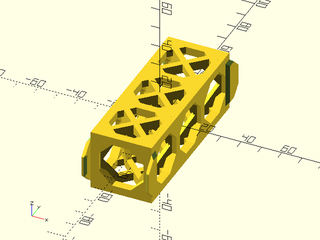
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss(extents=3, clips=[FRONT,BACK]);
Example 4:
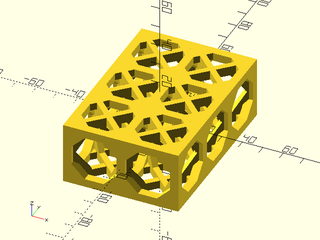
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss(extents=[2,3]);
Example 5:
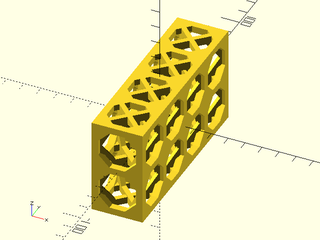
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss(extents=[1,4,2]);
Example 6:
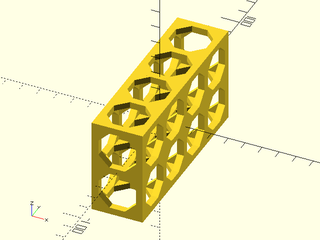
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss(extents=[1,4,2], bracing=false);
Synopsis: Creates a multi-cube corner cubetruss shape. [Geom]
Topics: Trusses, CubeTruss, FDM Optimized, Parts
See Also: cubetruss_segment(), cubetruss_support(), cubetruss()
Usage:
- cubetruss_corner(h, extents, [bracing=], [size=], [strut=], [clipthick=]);
Description:
Creates a corner cubetruss with extents jutting out in one or more directions.
Arguments:
By Position | What it does |
---|---|
h |
The number of cubes high to make the base and horizontal extents. |
extents |
The number of cubes to extend beyond the corner. If given as a vector of cube counts, gives the number of cubes to extend right, back, left, front, and up in order. If the vector is shorter than length 5 the extra cube counts are taken to be zero. |
bracing |
If true, adds internal cross-braces. Default: $cubetruss_bracing (usually true) |
size |
The length of each side of the cubetruss cubes. Default: $cubetruss_size (usually 30) |
strut |
The width of the struts on the cubetruss cubes. Default: $cubetruss_strut_size (usually 3) |
clipthick |
The thickness of the clips. Default: $cubetruss_clip_thickness (usually 1.6) |
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis. See spin. Default: 0
|
orient |
Vector to rotate top towards. See orient. Default: UP
|
Example 1:
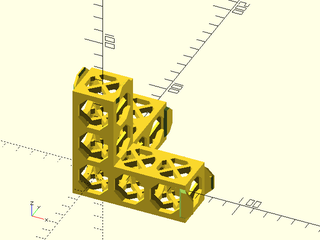
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_corner(extents=2);
Example 2:
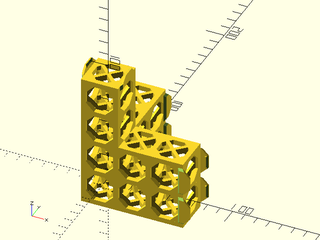
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_corner(extents=2, h=2);
Example 3:
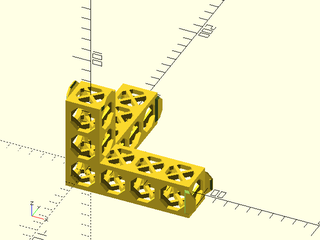
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_corner(extents=[3,3,0,0,2]);
Example 4:
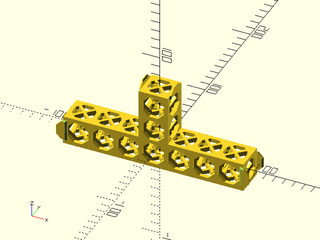
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_corner(extents=[3,0,3,0,2]);
Example 5:
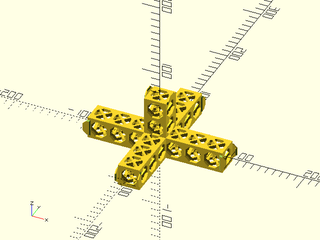
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_corner(extents=[3,3,3,3,2]);
Synopsis: Creates a cubetruss support structure shape. [Geom]
Topics: Trusses, CubeTruss, FDM Optimized, Parts
See Also: cubetruss_segment(), cubetruss(), cubetruss_corner()
Usage:
- cubetruss_support([size=], [strut=], [extents=]) [ATTACHMENTS];
Description:
Creates a single cubetruss support.
Arguments:
By Position | What it does |
---|---|
size |
The length of each side of the cubetruss cubes. Default: $cubetruss_size (usually 30) |
strut |
The width of the struts on the cubetruss cubes. Default: $cubetruss_strut_size (usually 3) |
extents |
If given as an integer, specifies the number of vertical segments for the support. If given as a list of 3 integers, specifies the number of segments in the X, Y, and Z directions. Default: 1. |
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis. See spin. Default: 0
|
orient |
Vector to rotate top towards. See orient. Default: UP
|
Example 1:
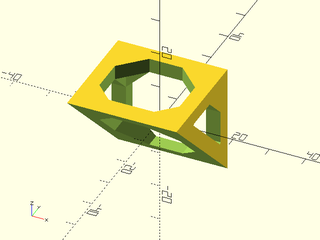
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_support();
Example 2:
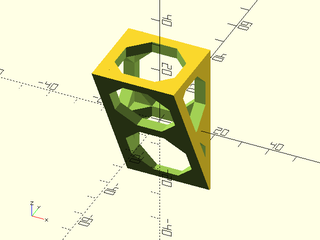
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_support(extents=2);
Example 3:
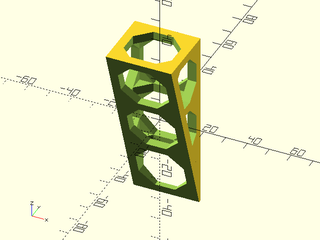
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_support(extents=3);
Example 4:
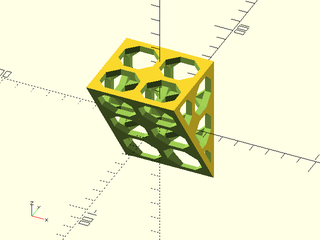
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_support(extents=[2,2,3]);
Example 5:
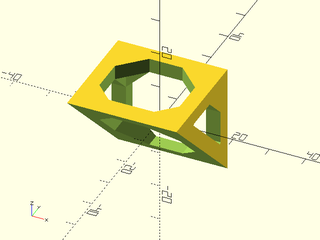
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_support(strut=4);
Example 6:
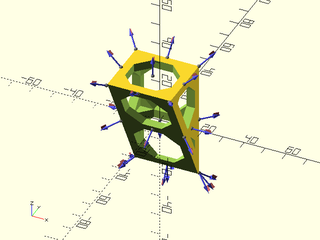
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_support(extents=2) show_anchors();
Synopsis: Creates a foot that can connect two cubetrusses. [Geom]
Topics: Trusses, CubeTruss, FDM Optimized, Parts
See Also: cubetruss_segment(), cubetruss_support(), cubetruss(), cubetruss_corner()
Usage:
- cubetruss_foot(w, [size=], [strut=], [clipthick=]) [ATTACHMENTS];
Description:
Creates a foot that can be clipped onto the bottom of a truss for support.
Arguments:
By Position | What it does |
---|---|
w |
The number of cube segments to span between the clips. Default: 1 |
size |
The length of each side of the cubetruss cubes. Default: $cubetruss_size (usually 30) |
strut |
The width of the struts on the cubetruss cubes. Default: $cubetruss_strut_size (usually 3) |
clipthick |
The thickness of the clips. Default: $cubetruss_clip_thickness (usually 1.6) |
By Name | What it does |
---|---|
$slop |
make fit looser to allow for printer overextrusion |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis. See spin. Default: 0
|
orient |
Vector to rotate top towards. See orient. Default: UP
|
Example 1:
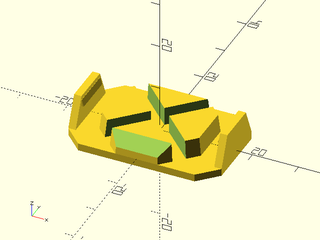
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_foot(w=1);
Example 2:
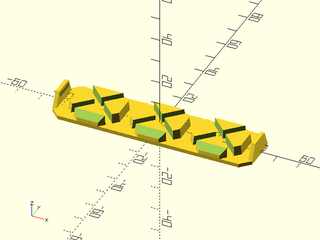
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_foot(w=3);
Synopsis: Creates a joiner that can connect two cubetrusses end-to-end. [Geom]
Topics: Trusses, CubeTruss, FDM Optimized, Parts
See Also: cubetruss_segment(), cubetruss_support(), cubetruss(), cubetruss_corner()
Usage:
- cubetruss_joiner([w=], [vert=], [size=], [strut=], [clipthick=]) [ATTACHMENTS];
Description:
Creates a part to join two cubetruss trusses end-to-end.
Arguments:
By Position | What it does |
---|---|
w |
The number of cube segments to span between the clips. Default: 1 |
vert |
If true, add vertical risers to clip to the ends of the cubetruss trusses. Default: true |
size |
The length of each side of the cubetruss cubes. Default: $cubetruss_size (usually 30) |
strut |
The width of the struts on the cubetruss cubes. Default: $cubetruss_strut_size (usually 3) |
clipthick |
The thickness of the clips. Default: $cubetruss_clip_thickness (usually 1.6) |
By Name | What it does |
---|---|
$slop |
Make fit looser by this amount to allow for printer overextrusion |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis. See spin. Default: 0
|
orient |
Vector to rotate top towards. See orient. Default: UP
|
Example 1:
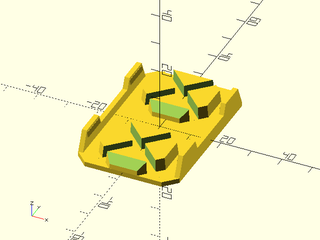
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_joiner(w=1, vert=false);
Example 2:
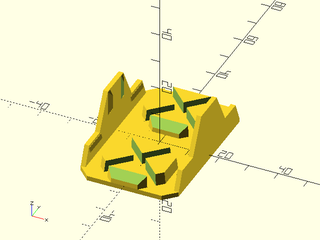
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_joiner(w=1, vert=true);
Example 3:
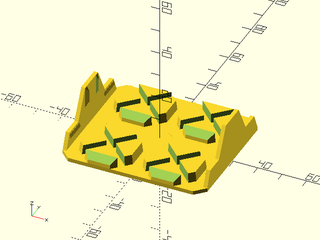
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_joiner(w=2, vert=true, anchor=BOT);
Synopsis: Creates a joiner that can connect two cubetrusses end-to-end. [Geom]
Topics: Trusses, CubeTruss, FDM Optimized, Parts
See Also: cubetruss_segment(), cubetruss_support(), cubetruss(), cubetruss_corner()
Usage:
- cubetruss_uclip(dual, [size=], [strut=], [clipthick=]) [ATTACHMENTS];
Description:
Creates a small clip that can snap around one or two adjacent struts.
Arguments:
By Position | What it does |
---|---|
dual |
If true, create a clip to clip around two adjacent struts. If false, just fit around one strut. Default: true |
size |
The length of each side of the cubetruss cubes. Default: $cubetruss_size (usually 30) |
strut |
The width of the struts on the cubetruss cubes. Default: $cubetruss_strut_size (usually 3) |
clipthick |
The thickness of the clips. Default: $cubetruss_clip_thickness (usually 1.6) |
By Name | What it does |
---|---|
$slop |
Make fit looser by this amount |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis. See spin. Default: 0
|
orient |
Vector to rotate top towards. See orient. Default: UP
|
Example 1:
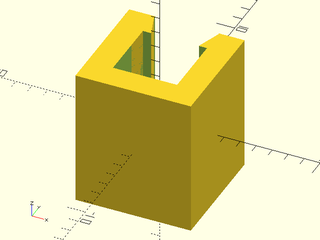
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_uclip(dual=false);
Example 2:
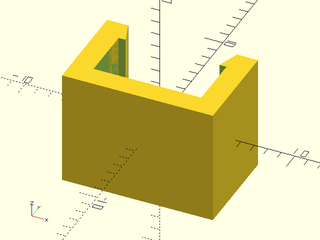
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_uclip(dual=true);
Synopsis: Creates a single cubetruss cube. [Geom]
Topics: Trusses, CubeTruss, FDM Optimized, Parts
See Also: cubetruss_support(), cubetruss(), cubetruss_corner()
Usage:
- cubetruss_segment([size=], [strut=], [bracing=]);
Description:
Creates a single cubetruss cube segment.
Arguments:
By Position | What it does |
---|---|
size |
The length of each side of the cubetruss cubes. Default: $cubetruss_size (usually 30) |
strut |
The width of the struts on the cubetruss cubes. Default: $cubetruss_strut_size (usually 3) |
bracing |
If true, adds internal cross-braces. Default: $cubetruss_bracing (usually true) |
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis. See spin. Default: 0
|
orient |
Vector to rotate top towards. See orient. Default: UP
|
Example 1:
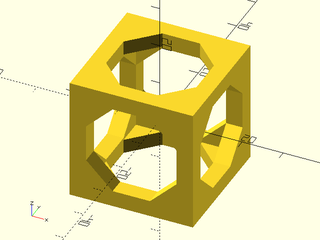
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_segment(bracing=false);
Example 2:
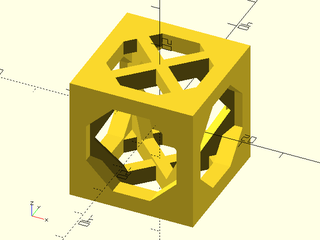
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_segment(bracing=true);
Example 3:
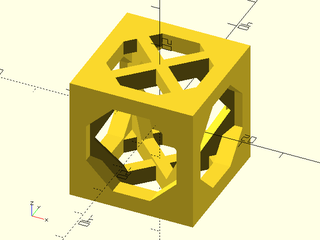
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_segment(strut=4);
Example 4:
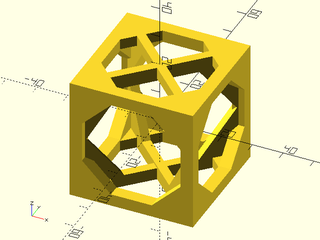
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_segment(size=40);
Synopsis: Creates a clip for the end of a cubetruss to snap-lock it to another cubetruss. [Geom]
Topics: Trusses, CubeTruss, FDM Optimized, Parts
See Also: cubetruss_segment(), cubetruss_support(), cubetruss(), cubetruss_corner()
Usage:
- cubetruss_clip(extents, [size=], [strut=], [clipthick=]) [ATTACHMENTS];
Description:
Creates a pair of clips to add onto the end of a truss.
Arguments:
By Position | What it does |
---|---|
extents |
How many cubes to separate the clips by. |
size |
The length of each side of the cubetruss cubes. Default: $cubetruss_size (usually 30) |
strut |
The width of the struts on the cubetruss cubes. Default: $cubetruss_strut_size (usually 3) |
clipthick |
The thickness of the clip. Default: $cubetruss_clip_thickness (usually 1.6) |
By Name | What it does |
---|---|
$slop |
allowance for printer overextrusion |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis. See spin. Default: 0
|
orient |
Vector to rotate top towards. See orient. Default: UP
|
Example 1:
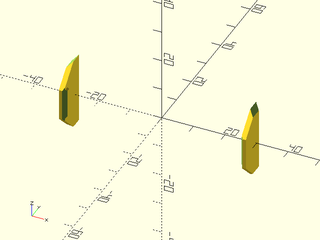
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_clip(extents=2);
Example 2:
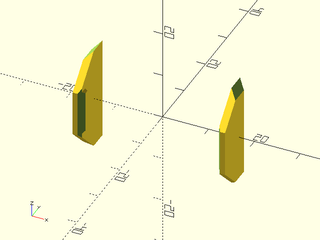
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_clip(extents=1);
Example 3:
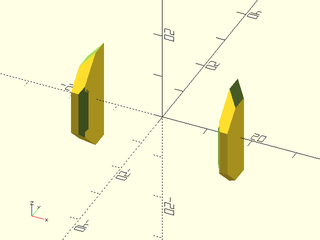
include <BOSL2/std.scad>
include <BOSL2/cubetruss.scad>
cubetruss_clip(clipthick=2.5);
Synopsis: Returns the length of a cubetruss truss.
Topics: Trusses, CubeTruss, FDM Optimized, Parts
See Also: cubetruss_segment(), cubetruss_support(), cubetruss(), cubetruss_corner()
Usage:
- length = cubetruss_dist(cubes, [gaps], [size=], [strut=]);
Description:
Function to calculate the length of a cubetruss truss.
Arguments:
By Position | What it does |
---|---|
cubes |
The number of cubes along the truss's length. |
gaps |
The number of extra strut widths to add in, corresponding to each time a truss butts up against another. |
size |
The length of each side of the cubetruss cubes. Default: $cubetruss_size (usually 30) |
strut |
The width of the struts on the cubetruss cubes. Default: $cubetruss_strut_size (usually 3) |
Table of Contents
Function Index
Topics Index
Cheat Sheet
Tutorials
Basic Modeling:
- constants.scad STD
- transforms.scad STD
- attachments.scad STD
- shapes2d.scad STD
- shapes3d.scad STD
- drawing.scad STD
- masks2d.scad STD
- masks3d.scad STD
- distributors.scad STD
- color.scad STD
- partitions.scad STD
- mutators.scad STD
Advanced Modeling:
Math:
- math.scad STD
- linalg.scad STD
- vectors.scad STD
- coords.scad STD
- geometry.scad STD
- trigonometry.scad STD
Data Management:
- version.scad STD
- comparisons.scad STD
- lists.scad STD
- utility.scad STD
- strings.scad STD
- structs.scad
- fnliterals.scad
Threaded Parts:
Parts:
- ball_bearings.scad
- cubetruss.scad
- gears.scad
- hinges.scad
- joiners.scad
- linear_bearings.scad
- modular_hose.scad
- nema_steppers.scad
- polyhedra.scad
- sliders.scad
- tripod_mounts.scad
- walls.scad
- wiring.scad
STD = Included in std.scad