masks3d.scad
This file defines 3D masks for applying chamfers, roundovers, and teardrop roundovers to straight edges and circular edges in three dimensions.
To use, add the following lines to the beginning of your file:
include <BOSL2/std.scad>
-
-
chamfer_edge_mask()
– Creates a shape to chamfer a 90° edge. [Geom] -
chamfer_corner_mask()
– Creates a shape to chamfer a 90° corner. [Geom] -
chamfer_cylinder_mask()
– Creates a shape to chamfer the end of a cylinder. [Geom]
-
-
-
rounding_edge_mask()
– Creates a shape to round a 90° edge. [Geom] -
rounding_corner_mask()
– Creates a shape to round 90° corners. [Geom] -
rounding_angled_edge_mask()
– Creates a shape to round edges of any angle. [Geom] -
rounding_angled_corner_mask()
– Creates a shape to round the corner of an arbitrary angle. [Geom] -
rounding_cylinder_mask()
– Creates a shape to round the end of a cylinder. [Geom] -
rounding_hole_mask()
– Creates a shape to round the edge of a round hole. [Geom]
-
-
-
teardrop_edge_mask()
– Creates a shape to round a 90° edge but limit the angle of overhang. [Geom] -
teardrop_corner_mask()
– Creates a shape to round a 90° corner but limit the angle of overhang. [Geom]
-
Synopsis: Creates a shape to chamfer a 90° edge. [Geom]
Topics: Masking, Chamfers, Shapes (3D)
See Also: chamfer_corner_mask(), chamfer_cylinder_mask(), default_tag(), diff()
Usage:
- chamfer_edge_mask(l|h=|length=|height=, chamfer, [excess]) [ATTACHMENTS];
Description:
Creates a shape that can be used to chamfer a 90° edge. Difference it from the object to be chamfered. The center of the mask object should align exactly with the edge to be chamfered.
Arguments:
By Position | What it does |
---|---|
l / h / length / height
|
Length of mask. |
chamfer |
Size of chamfer. |
excess |
The extra amount to add to the length of the mask so that it differences away from other shapes cleanly. Default: 0.1
|
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Side Effects:
- Tags the children with "remove" (and hence sets
$tag
) if no tag is already set.
Example 1:
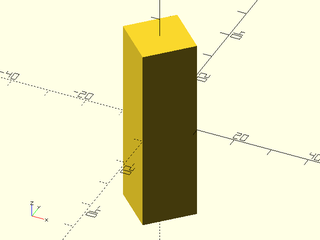
include <BOSL2/std.scad>
chamfer_edge_mask(l=50, chamfer=10);
Example 2:
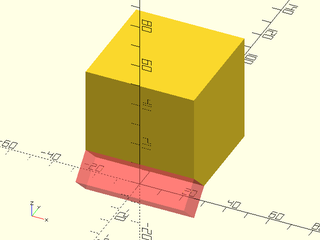
include <BOSL2/std.scad>
difference() {
cube(50, anchor=BOTTOM+FRONT);
#chamfer_edge_mask(l=50, chamfer=10, orient=RIGHT);
}
Example 3: Masking by Attachment
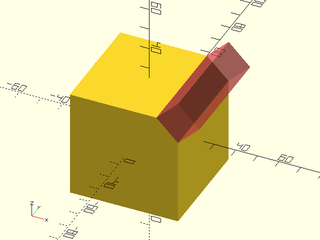
include <BOSL2/std.scad>
diff()
cube(50, center=true) {
edge_mask(TOP+RIGHT)
#chamfer_edge_mask(l=50, chamfer=10);
}
Synopsis: Creates a shape to chamfer a 90° corner. [Geom]
Topics: Masking, Chamfers, Shapes (3D)
See Also: chamfer_cylinder_mask(), chamfer_edge_mask(), default_tag(), diff()
Usage:
- chamfer_corner_mask(chamfer) [ATTACHMENTS];
Description:
Creates a shape that can be used to chamfer a 90° corner. Difference it from the object to be chamfered. The center of the mask object should align exactly with the corner to be chamfered.
Arguments:
By Position | What it does |
---|---|
chamfer |
Size of chamfer. |
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Side Effects:
- Tags the children with "remove" (and hence sets
$tag
) if no tag is already set.
Example 1:
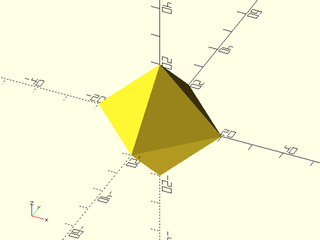
include <BOSL2/std.scad>
chamfer_corner_mask(chamfer=10);
Example 2:
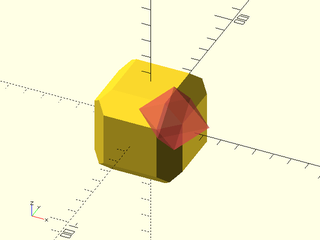
include <BOSL2/std.scad>
difference() {
cuboid(50, chamfer=10, trimcorners=false);
move(25*[1,-1,1]) #chamfer_corner_mask(chamfer=10);
}
Example 3: Masking by Attachment
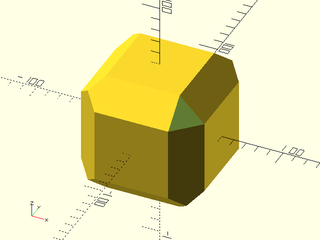
include <BOSL2/std.scad>
diff()
cuboid(100, chamfer=20, trimcorners=false) {
corner_mask(TOP+FWD+RIGHT)
chamfer_corner_mask(chamfer=20);
}
Example 4: Anchors
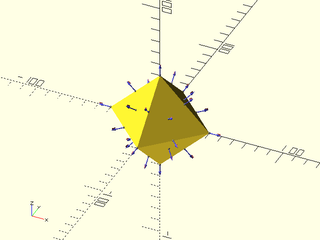
include <BOSL2/std.scad>
chamfer_corner_mask(chamfer=20)
show_anchors();
Synopsis: Creates a shape to chamfer the end of a cylinder. [Geom]
Topics: Masking, Chamfers, Cylinders
See Also: chamfer_corner_mask(), chamfer_edge_mask(), default_tag(), diff()
Usage:
- chamfer_cylinder_mask(r|d=, chamfer, [ang], [from_end]) [ATTACHMENTS];
Description:
Create a mask that can be used to bevel/chamfer the end of a cylindrical region. Difference it from the end of the region to be chamfered. The center of the mask object should align exactly with the center of the end of the cylindrical region to be chamfered.
Arguments:
By Position | What it does |
---|---|
r |
Radius of cylinder to chamfer. |
chamfer |
Size of the edge chamfered, inset from edge. |
By Name | What it does |
---|---|
d |
Diameter of cylinder to chamfer. Use instead of r. |
ang |
Angle of chamfer in degrees from the horizontal. (Default: 45) |
from_end |
If true, chamfer size is measured from end of cylinder. If false, chamfer is measured outset from the radius of the cylinder. (Default: false) |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Side Effects:
- Tags the children with "remove" (and hence sets
$tag
) if no tag is already set.
Example 1:
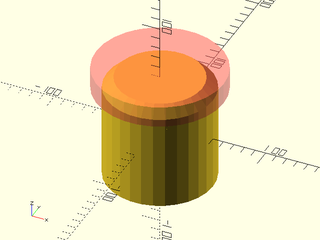
include <BOSL2/std.scad>
difference() {
cylinder(r=50, h=100, center=true);
up(50) #chamfer_cylinder_mask(r=50, chamfer=10);
}
Example 2:
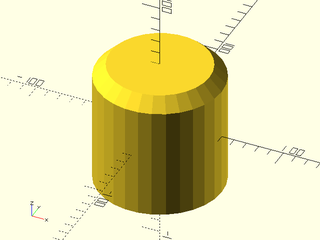
include <BOSL2/std.scad>
difference() {
cylinder(r=50, h=100, center=true);
up(50) chamfer_cylinder_mask(r=50, chamfer=10);
}
Example 3: Changing the chamfer angle
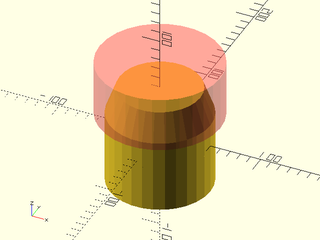
include <BOSL2/std.scad>
difference() {
cylinder(r=50, h=100, center=true);
up(50) #chamfer_cylinder_mask(r=50, chamfer=10, ang=70);
}
Example 4:
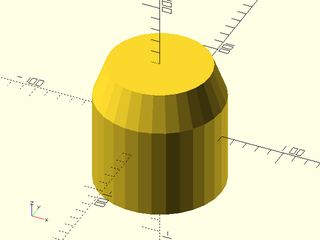
include <BOSL2/std.scad>
difference() {
cylinder(r=50, h=100, center=true);
up(50) chamfer_cylinder_mask(r=50, chamfer=10, ang=70);
}
Example 5: Masking by Attachment
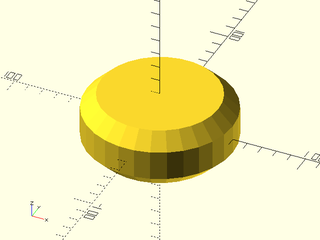
include <BOSL2/std.scad>
diff()
cyl(d=100,h=40)
attach([TOP,BOT])
tag("remove")chamfer_cylinder_mask(d=100, chamfer=10);
Synopsis: Creates a shape to round a 90° edge. [Geom]
Topics: Masks, Rounding, Shapes (3D)
See Also: rounding_angled_edge_mask(), rounding_corner_mask(), rounding_angled_corner_mask(), default_tag(), diff()
Usage:
- rounding_edge_mask(l|h=|length=|height=, r|d=, [excess=]) [ATTACHMENTS];
- rounding_edge_mask(l|h=|length=|height=, r1=|d1=, r2=|d2=, [excess=]) [ATTACHMENTS];
Description:
Creates a shape that can be used to round a vertical 90° edge. Difference it from the object to be rounded. The center of the mask object should align exactly with the edge to be rounded.
Arguments:
By Position | What it does |
---|---|
l / h / length / height
|
Length of mask. |
r |
Radius of the rounding. |
By Name | What it does |
---|---|
r1 |
Bottom radius of rounding. |
r2 |
Top radius of rounding. |
d |
Diameter of the rounding. |
d1 |
Bottom diameter of rounding. |
d2 |
Top diameter of rounding. |
excess |
Extra size for the mask. Defaults: 0.1 |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Side Effects:
- Tags the children with "remove" (and hence sets
$tag
) if no tag is already set.
Example 1:
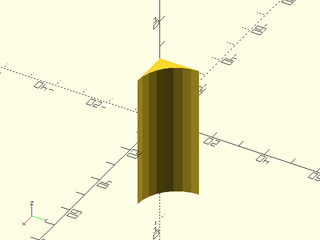
include <BOSL2/std.scad>
rounding_edge_mask(l=50, r1=10, r2=25);
Example 2:
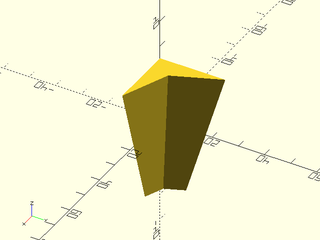
include <BOSL2/std.scad>
difference() {
cube(size=100, center=false);
#rounding_edge_mask(l=100, r=25, orient=UP, anchor=BOTTOM);
}
Example 3: Varying Rounding Radius
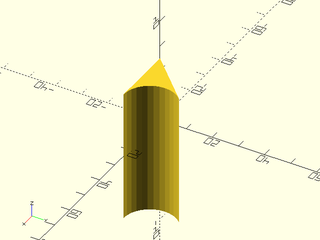
include <BOSL2/std.scad>
difference() {
cube(size=50, center=false);
#rounding_edge_mask(l=50, r1=25, r2=10, orient=UP, anchor=BOTTOM);
}
Example 4: Masking by Attachment
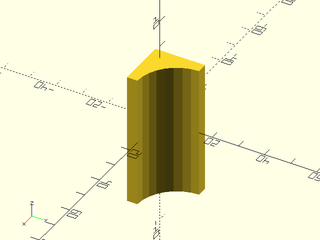
include <BOSL2/std.scad>
diff()
cube(100, center=true)
edge_mask(FRONT+RIGHT)
#rounding_edge_mask(l=$parent_size.z+0.01, r=25);
Example 5: Multiple Masking by Attachment
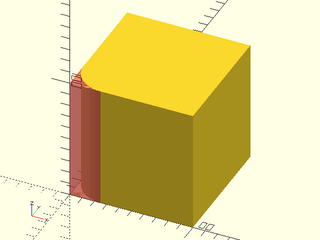
include <BOSL2/std.scad>
diff()
cube([80,90,100], center=true) {
let(p = $parent_size*1.01) {
edge_mask(TOP)
rounding_edge_mask(l=p.z, r=25);
}
}
Synopsis: Creates a shape to round 90° corners. [Geom]
Topics: Masking, Rounding, Shapes (3D)
See Also: rounding_angled_corner_mask(), rounding_edge_mask(), rounding_angled_edge_mask(), default_tag(), diff()
Usage:
- rounding_corner_mask(r|d, [excess=], [style=]) [ATTACHMENTS];
Description:
Creates a shape that you can use to round 90° corners. Difference it from the object to be rounded. The center of the mask object should align exactly with the corner to be rounded.
Arguments:
By Position | What it does |
---|---|
r |
Radius of corner rounding. |
By Name | What it does |
---|---|
d |
Diameter of corner rounding. |
excess |
Extra size for the mask. Defaults: 0.1 |
style |
The style of the sphere cutout's construction. One of "orig", "aligned", "stagger", "octa", or "icosa". Default: "octa" |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Side Effects:
- Tags the children with "remove" (and hence sets
$tag
) if no tag is already set.
Example 1:
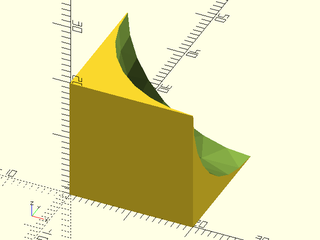
include <BOSL2/std.scad>
rounding_corner_mask(r=20.0);
Example 2:
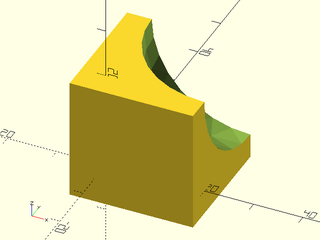
include <BOSL2/std.scad>
difference() {
cube(size=[50, 60, 70], center=true);
translate([-25, -30, 35])
#rounding_corner_mask(r=20, spin=90, orient=DOWN);
translate([25, -30, 35])
#rounding_corner_mask(r=20, orient=DOWN);
translate([25, -30, -35])
#rounding_corner_mask(r=20, spin=90);
}
Example 3: Masking by Attachment
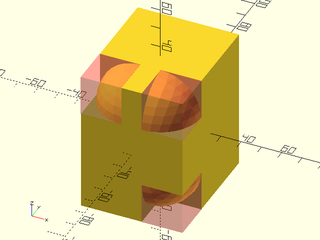
include <BOSL2/std.scad>
diff()
cube(size=[50, 60, 70]) {
corner_mask(TOP)
#rounding_corner_mask(r=20);
}
Synopsis: Creates a shape to round edges of any angle. [Geom]
See Also: rounding_angled_corner_mask(), rounding_edge_mask(), rounding_corner_mask(), default_tag(), diff()
Usage:
- rounding_angled_edge_mask(h|l=|length=|height=, r|d=, [ang=]) [ATTACHMENTS];
- rounding_angled_edge_mask(h|l=|length=|height=, r1=|d1=, r2=|d2=, [ang=]) [ATTACHMENTS];
Description:
Creates a vertical mask that can be used to round the edge where two face meet, at any arbitrary angle. Difference it from the object to be rounded. The center of the mask should align exactly with the edge to be rounded.
Arguments:
By Position | What it does |
---|---|
h / l / height / length
|
Height of vertical mask. |
r |
Radius of the rounding. |
By Name | What it does |
---|---|
r1 |
Bottom radius of rounding. |
r2 |
Top radius of rounding. |
d |
Diameter of the rounding. |
d1 |
Bottom diameter of rounding. |
d2 |
Top diameter of rounding. |
ang |
Angle that the planes meet at. Default: 90 |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Side Effects:
- Tags the children with "remove" (and hence sets
$tag
) if no tag is already set.
Example 1:
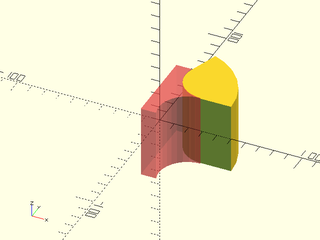
include <BOSL2/std.scad>
difference() {
pie_slice(ang=70, h=50, d=100, center=true);
#rounding_angled_edge_mask(h=51, r=20.0, ang=70, $fn=32);
}
Example 2: Varying Rounding Radius
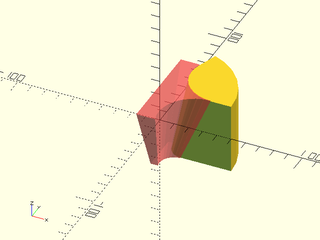
include <BOSL2/std.scad>
difference() {
pie_slice(ang=70, h=50, d=100, center=true);
#rounding_angled_edge_mask(h=51, r1=10, r2=25, ang=70, $fn=32);
}
Synopsis: Creates a shape to round the corner of an arbitrary angle. [Geom]
Topics: Masks, Rounding, Shapes (3D)
See Also: rounding_angled_edge_mask(), rounding_corner_mask(), rounding_edge_mask(), default_tag(), diff()
Usage:
- rounding_angled_corner_mask(r|d=, [ang]) [ATTACHMENTS];
Description:
Creates a shape that can be used to round the corner of an angle. Difference it from the object to be rounded. The center of the mask object should align exactly with the point of the corner to be rounded.
Arguments:
By Position | What it does |
---|---|
r |
Radius of the rounding. |
By Name | What it does |
---|---|
d |
Diameter of the rounding. |
ang |
Angle between planes that you need to round the corner of. Default: 90 |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Side Effects:
- Tags the children with "remove" (and hence sets
$tag
) if no tag is already set.
Example 1:
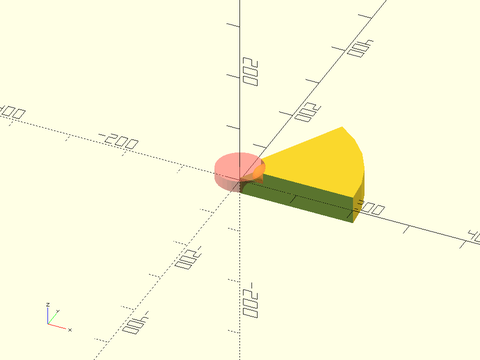
include <BOSL2/std.scad>
ang=60;
difference() {
pie_slice(ang=ang, h=50, r=200, center=true);
up(50/2) #rounding_angled_corner_mask(r=20, ang=ang);
}
Synopsis: Creates a shape to round the end of a cylinder. [Geom]
Topics: Masking, Rounding, Cylinders
See Also: rounding_hole_mask(), rounding_angled_edge_mask(), rounding_corner_mask(), rounding_angled_corner_mask(), default_tag(), diff()
Usage:
- rounding_cylinder_mask(r|d=, rounding);
Description:
Create a mask that can be used to round the end of a cylinder. Difference it from the cylinder to be rounded. The center of the mask object should align exactly with the center of the end of the cylinder to be rounded.
Arguments:
By Position | What it does |
---|---|
r |
Radius of cylinder. |
rounding |
Radius of the edge rounding. |
By Name | What it does |
---|---|
d |
Diameter of cylinder. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Side Effects:
- Tags the children with "remove" (and hence sets
$tag
) if no tag is already set.
Example 1:
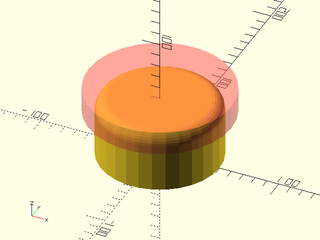
include <BOSL2/std.scad>
difference() {
cylinder(r=50, h=50, center=false);
up(50) #rounding_cylinder_mask(r=50, rounding=10);
}
Example 2:
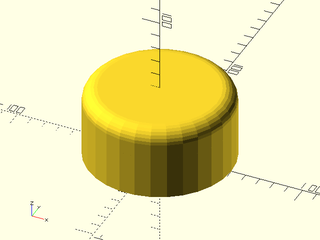
include <BOSL2/std.scad>
difference() {
cylinder(r=50, h=50, center=false);
up(50) rounding_cylinder_mask(r=50, rounding=10);
}
Example 3: Masking by Attachment
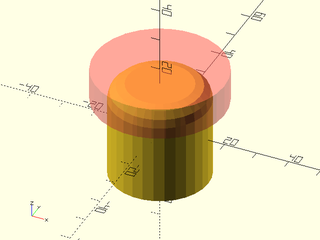
include <BOSL2/std.scad>
diff()
cyl(h=30, d=30) {
attach(TOP)
#tag("remove")
rounding_cylinder_mask(d=30, rounding=5);
}
Synopsis: Creates a shape to round the edge of a round hole. [Geom]
See Also: rounding_cylinder_mask(), rounding_angled_edge_mask(), rounding_corner_mask(), rounding_angled_corner_mask(), default_tag(), diff()
Usage:
- rounding_hole_mask(r|d, rounding, [excess]) [ATTACHMENTS];
Description:
Create a mask that can be used to round the edge of a circular hole. Difference it from the hole to be rounded. The center of the mask object should align exactly with the center of the end of the hole to be rounded.
Arguments:
By Position | What it does |
---|---|
r |
Radius of hole. |
rounding |
Radius of the rounding. |
excess |
The extra thickness of the mask. Default: 0.1 . |
By Name | What it does |
---|---|
d |
Diameter of hole to rounding. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Side Effects:
- Tags the children with "remove" (and hence sets
$tag
) if no tag is already set.
Example 1:
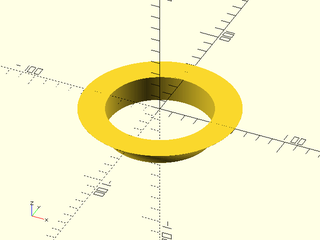
include <BOSL2/std.scad>
rounding_hole_mask(r=40, rounding=20, $fa=2, $fs=2);
Example 2:
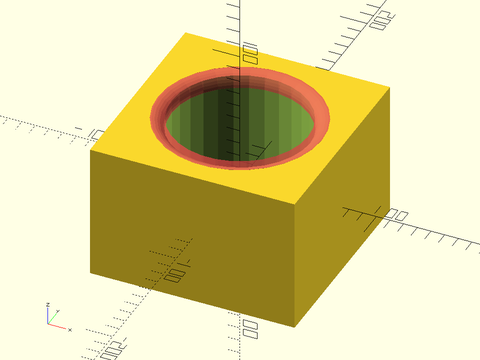
include <BOSL2/std.scad>
difference() {
cube([150,150,100], center=true);
cylinder(r=50, h=100.1, center=true);
up(50) #rounding_hole_mask(r=50, rounding=10);
}
Example 3:
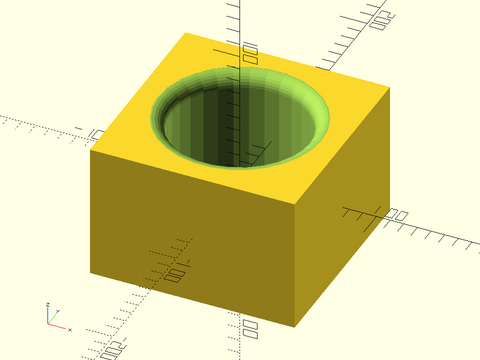
include <BOSL2/std.scad>
difference() {
cube([150,150,100], center=true);
cylinder(r=50, h=100.1, center=true);
up(50) rounding_hole_mask(r=50, rounding=10);
}
Synopsis: Creates a shape to round a 90° edge but limit the angle of overhang. [Geom]
Topics: Masking, Rounding, Shapes (3D), FDM Optimized
See Also: teardrop_corner_mask(), default_tag(), diff()
Usage:
- teardrop_edge_mask(l|h=|length=|height=, r|d=, [angle], [excess], [anchor], [spin], [orient]) [ATTACHMENTS];
Description:
Makes an apropriate 3D edge rounding mask that keeps within angle
degrees of vertical.
Arguments:
By Position | What it does |
---|---|
l / h / length / height
|
length of mask |
r |
Radius of the mask rounding. |
angle |
Maximum angle from vertical. Default: 45 |
excess |
Excess mask size. Default: 0.1 |
By Name | What it does |
---|---|
d |
Diameter of the mask rounding. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Side Effects:
- Tags the children with "remove" (and hence sets
$tag
) if no tag is already set.
Example 1:
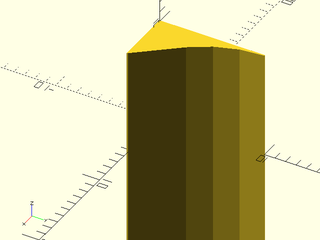
include <BOSL2/std.scad>
teardrop_edge_mask(l=20, r=10, angle=40);
Example 2:
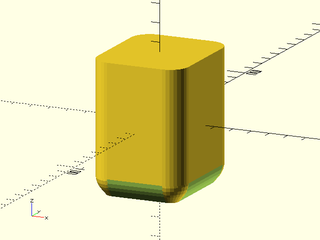
include <BOSL2/std.scad>
diff()
cuboid([50,60,70],rounding=10,edges="Z",anchor=CENTER) {
edge_mask(BOT)
teardrop_edge_mask(l=max($parent_size)+1, r=10, angle=40);
corner_mask(BOT)
teardrop_corner_mask(r=10, angle=40);
}
Synopsis: Creates a shape to round a 90° corner but limit the angle of overhang. [Geom]
Topics: Masking, Rounding, Shapes (3D), FDM Optimized
See Also: teardrop_edge_mask(), default_tag(), diff()
Usage:
- teardrop_corner_mask(r|d=, [angle], [excess], [anchor], [spin], [orient]) [ATTACHMENTS];
Description:
Makes an apropriate 3D corner rounding mask that keeps within angle
degrees of vertical.
Arguments:
By Position | What it does |
---|---|
r |
Radius of the mask rounding. |
angle |
Maximum angle from vertical. Default: 45 |
excess |
Excess mask size. Default: 0.1 |
By Name | What it does |
---|---|
d |
Diameter of the mask rounding. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Side Effects:
- Tags the children with "remove" (and hence sets
$tag
) if no tag is already set.
Example 1:
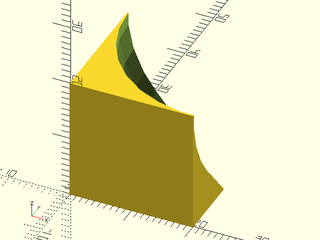
include <BOSL2/std.scad>
teardrop_corner_mask(r=20, angle=40);
Example 2:
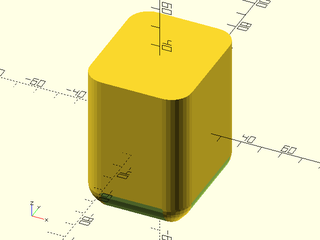
include <BOSL2/std.scad>
diff()
cuboid([50,60,70],rounding=10,edges="Z",anchor=CENTER) {
edge_profile(BOT)
mask2d_teardrop(r=10, angle=40);
corner_mask(BOT)
teardrop_corner_mask(r=10, angle=40);
}
Table of Contents
Function Index
Topics Index
Cheat Sheet
Tutorials
Basic Modeling:
- constants.scad STD
- transforms.scad STD
- attachments.scad STD
- shapes2d.scad STD
- shapes3d.scad STD
- drawing.scad STD
- masks2d.scad STD
- masks3d.scad STD
- distributors.scad STD
- color.scad STD
- partitions.scad STD
- mutators.scad STD
Advanced Modeling:
Math:
- math.scad STD
- linalg.scad STD
- vectors.scad STD
- coords.scad STD
- geometry.scad STD
- trigonometry.scad STD
Data Management:
- version.scad STD
- comparisons.scad STD
- lists.scad STD
- utility.scad STD
- strings.scad STD
- structs.scad
- fnliterals.scad
Threaded Parts:
Parts:
- ball_bearings.scad
- cubetruss.scad
- gears.scad
- hinges.scad
- joiners.scad
- linear_bearings.scad
- modular_hose.scad
- nema_steppers.scad
- polyhedra.scad
- sliders.scad
- tripod_mounts.scad
- walls.scad
- wiring.scad
STD = Included in std.scad