coords.scad
Coordinate transformations and coordinate system conversions.
To use, add the following lines to the beginning of your file:
include <BOSL2/std.scad>
-
-
polar_to_xy()
– Convert 2D polar coordinates to cartesian coordinates. [Path] -
xy_to_polar()
– Convert 2D cartesian coordinates to polar coordinates (radius and angle) -
project_plane()
– Project a set of points onto a specified plane, returning 2D points. [Path] -
lift_plane()
– Map a list of 2D points onto a plane in 3D. [Path] -
cylindrical_to_xyz()
– Convert cylindrical coordinates to cartesian coordinates. [Path] -
xyz_to_cylindrical()
– Convert 3D cartesian coordinates to cylindrical coordinates. -
spherical_to_xyz()
– Convert spherical coordinates to 3D cartesian coordinates. [Path] -
xyz_to_spherical()
– Convert 3D cartesian coordinates to spherical coordinates. -
altaz_to_xyz()
– Convert altitude/azimuth/range to 3D cartesian coordinates. [Path] -
xyz_to_altaz()
– Convert 3D cartesian coordinates to [altitude,azimuth,range].
-
Synopsis: Convert a vector to 2D.
Topics: Coordinates, Points
See Also: path2d(), point3d(), path3d()
Usage:
- pt = point2d(p, [fill]);
Description:
Returns a 2D vector/point from a 2D or 3D vector. If given a 3D point, removes the Z coordinate.
Arguments:
By Position | What it does |
---|---|
p |
The coordinates to force into a 2D vector/point. |
fill |
Value to fill missing values in vector with. Default: 0 |
Synopsis: Convert a path to 2D. [Path]
Topics: Coordinates, Points, Paths
See Also: point2d(), point3d(), path3d()
Usage:
- pts = path2d(points);
Description:
Returns a list of 2D vectors/points from a list of 2D, 3D or higher dimensional vectors/points. Removes the extra coordinates from higher dimensional points. The input must be a path, where every vector has the same length.
Arguments:
By Position | What it does |
---|---|
points |
A list of 2D or 3D points/vectors. |
Synopsis: Convert a vector to 3D.
Topics: Coordinates, Points
See Also: path2d(), point2d(), path3d()
Usage:
- pt = point3d(p, [fill]);
Description:
Returns a 3D vector/point from a 2D or 3D vector.
Arguments:
By Position | What it does |
---|---|
p |
The coordinates to force into a 3D vector/point. |
fill |
Value to fill missing values in vector with. Default: 0 |
Synopsis: Convert a path to 3D. [Path]
Topics: Coordinates, Points, Paths
See Also: point2d(), path2d(), point3d()
Usage:
- pts = path3d(points, [fill]);
Description:
Returns a list of 3D vectors/points from a list of 2D or higher dimensional vectors/points by removing extra coordinates or adding the z coordinate.
Arguments:
By Position | What it does |
---|---|
points |
A list of 2D, 3D or higher dimensional points/vectors. |
fill |
Value to fill missing values in vectors with (in the 2D case). Default: 0 |
Synopsis: Convert a vector to 4d.
Topics: Coordinates, Points
See Also: point2d(), path2d(), point3d(), path3d(), path4d()
Usage:
- pt = point4d(p, [fill]);
Description:
Returns a 4D vector/point from a 2D or 3D vector.
Arguments:
By Position | What it does |
---|---|
p |
The coordinates to force into a 4D vector/point. |
fill |
Value to fill missing values in vector with. Default: 0 |
Synopsis: Convert a path to 4d. [Path]
Topics: Coordinates, Points, Paths
See Also: point2d(), path2d(), point3d(), path3d(), point4d()
Usage:
- pt = path4d(points, [fill]);
Description:
Returns a list of 4D vectors/points from a list of 2D or 3D vectors/points.
Arguments:
By Position | What it does |
---|---|
points |
A list of 2D or 3D points/vectors. |
fill |
Value to fill missing values in vectors with. Default: 0 |
Synopsis: Convert 2D polar coordinates to cartesian coordinates. [Path]
Topics: Coordinates, Points, Paths
See Also: xy_to_polar(), xyz_to_cylindrical(), cylindrical_to_xyz(), xyz_to_spherical(), spherical_to_xyz()
Usage:
- pt = polar_to_xy(r, theta);
- pt = polar_to_xy([R, THETA]);
- pts = polar_to_xy([[R,THETA], [R,THETA], ...]);
Description:
Called with two arguments, converts the r
and theta
2D polar coordinate into an [X,Y]
cartesian coordinate.
Called with one [R,THETA]
vector argument, converts the 2D polar coordinate into an [X,Y]
cartesian coordinate.
Called with a list of [R,THETA]
vector arguments, converts each 2D polar coordinate into [X,Y]
cartesian coordinates.
Theta is the angle counter-clockwise of X+ on the XY plane.
Arguments:
By Position | What it does |
---|---|
r |
distance from the origin. |
theta |
angle in degrees, counter-clockwise of X+. |
Example 1:
include <BOSL2/std.scad>
xy = polar_to_xy(20,45); // Returns: ~[14.1421365, 14.1421365]
xy = polar_to_xy(40,30); // Returns: ~[34.6410162, 15]
xy = polar_to_xy([40,30]); // Returns: ~[34.6410162, 15]
xy = polar_to_xy([[40,30],[20,120]]); // Returns: ~[[34.6410162, 15], [-10, 17.3205]]
Example 2:
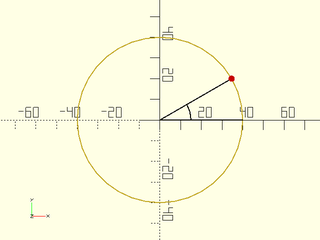
include <BOSL2/std.scad>
r=40; ang=30; $fn=36;
pt = polar_to_xy(r,ang);
stroke(circle(r=r), closed=true, width=0.5);
color("black") stroke([[r,0], [0,0], pt], width=0.5);
color("black") stroke(arc(r=15, angle=ang), width=0.5);
color("red") move(pt) circle(d=3);
Synopsis: Convert 2D cartesian coordinates to polar coordinates (radius and angle)
Topics: Coordinates, Points, Paths
See Also: polar_to_xy(), xyz_to_cylindrical(), cylindrical_to_xyz(), xyz_to_spherical(), spherical_to_xyz()
Usage:
- r_theta = xy_to_polar(x,y);
- r_theta = xy_to_polar([X,Y]);
- r_thetas = xy_to_polar([[X,Y], [X,Y], ...]);
Description:
Called with two arguments, converts the x
and y
2D cartesian coordinate into a [RADIUS,THETA]
polar coordinate.
Called with one [X,Y]
vector argument, converts the 2D cartesian coordinate into a [RADIUS,THETA]
polar coordinate.
Called with a list of [X,Y]
vector arguments, converts each 2D cartesian coordinate into [RADIUS,THETA]
polar coordinates.
Theta is the angle counter-clockwise of X+ on the XY plane.
Arguments:
By Position | What it does |
---|---|
x |
X coordinate. |
y |
Y coordinate. |
Example 1:
include <BOSL2/std.scad>
plr = xy_to_polar(20,30);
plr = xy_to_polar([40,60]);
plrs = xy_to_polar([[40,60],[-10,20]]);
Example 2:
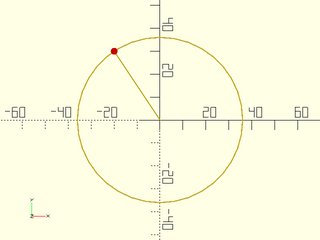
include <BOSL2/std.scad>
pt = [-20,30]; $fn = 36;
rt = xy_to_polar(pt);
r = rt[0]; ang = rt[1];
stroke(circle(r=r), closed=true, width=0.5);
zrot(ang) stroke([[0,0],[r,0]],width=0.5);
color("red") move(pt) circle(d=3);
Synopsis: Project a set of points onto a specified plane, returning 2D points. [Path]
Topics: Coordinates, Points, Paths
See Also: lift_plane()
Usage:
- xy = project_plane(plane, p);
Usage: To get a transform matrix
- M = project_plane(plane)
Description:
Maps the provided 3D point(s) from 3D coordinates to a 2D coordinate system defined by plane
. Points that are not
on the specified plane will be projected orthogonally onto the plane. This coordinate system is useful if you need
to perform 2D operations on a coplanar set of data. After those operations are done you can return the data
to 3D with lift_plane()
. You could also use this to force approximately coplanar data to be exactly coplanar.
The parameter p can be a point, path, region, bezier patch or VNF.
The plane can be specified as
- A list of three points. The planar coordinate system will have [0,0] at plane[0], and plane[1] will lie on the Y+ axis.
- A list of coplanar points that define a plane (not-collinear)
- A plane definition
[A,B,C,D]
whereAx+By+CZ=D
. The closest point on that plane to the origin will map to the origin in the new coordinate system.
If you omit the point specification then project_plane()
returns a rotation matrix that maps the specified plane to the XY plane.
Note that if you apply this transformation to data lying on the plane it will produce 3D points with the Z coordinate of zero.
Arguments:
By Position | What it does |
---|---|
plane |
plane specification or point list defining the plane |
p |
3D point, path, region, VNF or bezier patch to project |
Example 1:
include <BOSL2/std.scad>
pt = [5,-5,5];
a=[0,0,0]; b=[10,-10,0]; c=[10,0,10];
xy = project_plane([a,b,c],pt);
Example 2: The yellow points in 3D project onto the red points in 2D
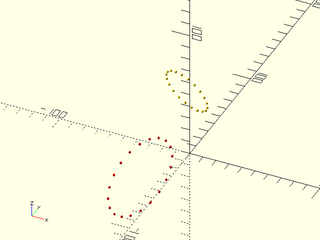
include <BOSL2/std.scad>
M = [[-1, 2, -1, -2], [-1, -3, 2, -1], [2, 3, 4, 53], [0, 0, 0, 1]];
data = apply(M,path3d(circle(r=10, $fn=20)));
move_copies(data) sphere(r=1);
color("red") move_copies(project_plane(data, data)) sphere(r=1);
Example 3:
include <BOSL2/std.scad>
xyzpath = move([10,20,30], p=yrot(25, p=path3d(circle(d=100))));
mat = project_plane(xyzpath);
xypath = path2d(apply(mat, xyzpath));
#stroke(xyzpath,closed=true);
stroke(xypath,closed=true);
Synopsis: Map a list of 2D points onto a plane in 3D. [Path]
Topics: Coordinates, Points, Paths
See Also: project_plane()
Usage:
- xyz = lift_plane(plane, p);
Usage: to get transform matrix
- M = lift_plane(plane);
Description:
Converts the given 2D point on the plane to 3D coordinates of the specified plane. The parameter p can be a point, path, region, bezier patch or VNF. The plane can be specified as
- A list of three points. The planar coordinate system will have [0,0] at plane[0], and plane[1] will lie on the Y+ axis.
- A list of coplanar points that define a plane (not-collinear)
- A plane definition
[A,B,C,D]
whereAx+By+CZ=D
. The closest point on that plane to the origin will map to the origin in the new coordinate system.
If you do not supply p
then you get a transformation matrix which operates in 3D, assuming that the Z coordinate of the points is zero.
This matrix is a rotation, the inverse of the one produced by project_plane.
Arguments:
By Position | What it does |
---|---|
plane |
Plane specification or list of points to define a plane |
p |
points, path, region, VNF, or bezier patch to transform. |
Synopsis: Convert cylindrical coordinates to cartesian coordinates. [Path]
Topics: Coordinates, Points, Paths
See Also: xyz_to_cylindrical(), xy_to_polar(), polar_to_xy(), xyz_to_spherical(), spherical_to_xyz()
Usage:
- pt = cylindrical_to_xyz(r, theta, z);
- pt = cylindrical_to_xyz([RADIUS,THETA,Z]);
- pts = cylindrical_to_xyz([[RADIUS,THETA,Z], [RADIUS,THETA,Z], ...]);
Description:
Called with three arguments, converts the r
, theta
, and 'z' 3D cylindrical coordinate into an [X,Y,Z]
cartesian coordinate.
Called with one [RADIUS,THETA,Z]
vector argument, converts the 3D cylindrical coordinate into an [X,Y,Z]
cartesian coordinate.
Called with a list of [RADIUS,THETA,Z]
vector arguments, converts each 3D cylindrical coordinate into [X,Y,Z]
cartesian coordinates.
Theta is the angle counter-clockwise of X+ on the XY plane. Z is height above the XY plane.
Arguments:
By Position | What it does |
---|---|
r |
distance from the Z axis. |
theta |
angle in degrees, counter-clockwise of X+ on the XY plane. |
z |
Height above XY plane. |
Example 1:
include <BOSL2/std.scad>
xyz = cylindrical_to_xyz(20,30,40);
xyz = cylindrical_to_xyz([40,60,50]);
Synopsis: Convert 3D cartesian coordinates to cylindrical coordinates.
Topics: Coordinates, Points, Paths
See Also: cylindrical_to_xyz(), xy_to_polar(), polar_to_xy(), xyz_to_spherical(), spherical_to_xyz()
Usage:
- rtz = xyz_to_cylindrical(x,y,z);
- rtz = xyz_to_cylindrical([X,Y,Z]);
- rtzs = xyz_to_cylindrical([[X,Y,Z], [X,Y,Z], ...]);
Description:
Called with three arguments, converts the x
, y
, and z
3D cartesian coordinate into a [RADIUS,THETA,Z]
cylindrical coordinate.
Called with one [X,Y,Z]
vector argument, converts the 3D cartesian coordinate into a [RADIUS,THETA,Z]
cylindrical coordinate.
Called with a list of [X,Y,Z]
vector arguments, converts each 3D cartesian coordinate into [RADIUS,THETA,Z]
cylindrical coordinates.
Theta is the angle counter-clockwise of X+ on the XY plane. Z is height above the XY plane.
Arguments:
By Position | What it does |
---|---|
x |
X coordinate. |
y |
Y coordinate. |
z |
Z coordinate. |
Example 1:
include <BOSL2/std.scad>
cyl = xyz_to_cylindrical(20,30,40);
cyl = xyz_to_cylindrical([40,50,70]);
cyls = xyz_to_cylindrical([[40,50,70], [-10,15,-30]]);
Synopsis: Convert spherical coordinates to 3D cartesian coordinates. [Path]
Topics: Coordinates, Points, Paths
See Also: cylindrical_to_xyz(), xyz_to_spherical(), xyz_to_cylindrical(), altaz_to_xyz(), xyz_to_altaz()
Usage:
- pt = spherical_to_xyz(r, theta, phi);
- pt = spherical_to_xyz([RADIUS,THETA,PHI]);
- pts = spherical_to_xyz([[RADIUS,THETA,PHI], [RADIUS,THETA,PHI], ...]);
Description:
Called with three arguments, converts the r
, theta
, and 'phi' 3D spherical coordinate into an [X,Y,Z]
cartesian coordinate.
Called with one [RADIUS,THETA,PHI]
vector argument, converts the 3D spherical coordinate into an [X,Y,Z]
cartesian coordinate.
Called with a list of [RADIUS,THETA,PHI]
vector arguments, converts each 3D spherical coordinate into [X,Y,Z]
cartesian coordinates.
Theta is the angle counter-clockwise of X+ on the XY plane. Phi is the angle down from the Z+ pole.
Arguments:
By Position | What it does |
---|---|
r |
distance from origin. |
theta |
angle in degrees, counter-clockwise of X+ on the XY plane. |
phi |
angle in degrees from the vertical Z+ axis. |
Example 1:
include <BOSL2/std.scad>
xyz = spherical_to_xyz(20,30,40);
xyz = spherical_to_xyz([40,60,50]);
xyzs = spherical_to_xyz([[40,60,50], [50,120,100]]);
Synopsis: Convert 3D cartesian coordinates to spherical coordinates.
Topics: Coordinates, Points, Paths
See Also: cylindrical_to_xyz(), spherical_to_xyz(), xyz_to_cylindrical(), altaz_to_xyz(), xyz_to_altaz()
Usage:
- r_theta_phi = xyz_to_spherical(x,y,z)
- r_theta_phi = xyz_to_spherical([X,Y,Z])
- r_theta_phis = xyz_to_spherical([[X,Y,Z], [X,Y,Z], ...])
Description:
Called with three arguments, converts the x
, y
, and z
3D cartesian coordinate into a [RADIUS,THETA,PHI]
spherical coordinate.
Called with one [X,Y,Z]
vector argument, converts the 3D cartesian coordinate into a [RADIUS,THETA,PHI]
spherical coordinate.
Called with a list of [X,Y,Z]
vector arguments, converts each 3D cartesian coordinate into [RADIUS,THETA,PHI]
spherical coordinates.
Theta is the angle counter-clockwise of X+ on the XY plane. Phi is the angle down from the Z+ pole.
Arguments:
By Position | What it does |
---|---|
x |
X coordinate. |
y |
Y coordinate. |
z |
Z coordinate. |
Example 1:
include <BOSL2/std.scad>
sph = xyz_to_spherical(20,30,40);
sph = xyz_to_spherical([40,50,70]);
sphs = xyz_to_spherical([[40,50,70], [25,-14,27]]);
Synopsis: Convert altitude/azimuth/range to 3D cartesian coordinates. [Path]
Topics: Coordinates, Points, Paths
See Also: cylindrical_to_xyz(), xyz_to_spherical(), spherical_to_xyz(), xyz_to_cylindrical(), xyz_to_altaz()
Usage:
- pt = altaz_to_xyz(alt, az, r);
- pt = altaz_to_xyz([ALT,AZ,R]);
- pts = altaz_to_xyz([[ALT,AZ,R], [ALT,AZ,R], ...]);
Description:
Convert altitude/azimuth/range coordinates to 3D cartesian coordinates.
Called with three arguments, converts the alt
, az
, and 'r' 3D altitude-azimuth coordinate into an [X,Y,Z]
cartesian coordinate.
Called with one [ALTITUDE,AZIMUTH,RANGE]
vector argument, converts the 3D alt-az coordinate into an [X,Y,Z]
cartesian coordinate.
Called with a list of [ALTITUDE,AZIMUTH,RANGE]
vector arguments, converts each 3D alt-az coordinate into [X,Y,Z]
cartesian coordinates.
Altitude is the angle above the XY plane, Azimuth is degrees clockwise of Y+ on the XY plane, and Range is the distance from the origin.
Arguments:
By Position | What it does |
---|---|
alt |
altitude angle in degrees above the XY plane. |
az |
azimuth angle in degrees clockwise of Y+ on the XY plane. |
r |
distance from origin. |
Example 1:
include <BOSL2/std.scad>
xyz = altaz_to_xyz(20,30,40);
xyz = altaz_to_xyz([40,60,50]);
Synopsis: Convert 3D cartesian coordinates to [altitude,azimuth,range].
Topics: Coordinates, Points, Paths
See Also: cylindrical_to_xyz(), xyz_to_spherical(), spherical_to_xyz(), xyz_to_cylindrical(), altaz_to_xyz()
Usage:
- alt_az_r = xyz_to_altaz(x,y,z);
- alt_az_r = xyz_to_altaz([X,Y,Z]);
- alt_az_rs = xyz_to_altaz([[X,Y,Z], [X,Y,Z], ...]);
Description:
Converts 3D cartesian coordinates to altitude/azimuth/range coordinates.
Called with three arguments, converts the x
, y
, and z
3D cartesian coordinate into an [ALTITUDE,AZIMUTH,RANGE]
coordinate.
Called with one [X,Y,Z]
vector argument, converts the 3D cartesian coordinate into a [ALTITUDE,AZIMUTH,RANGE]
coordinate.
Called with a list of [X,Y,Z]
vector arguments, converts each 3D cartesian coordinate into [ALTITUDE,AZIMUTH,RANGE]
coordinates.
Altitude is the angle above the XY plane, Azimuth is degrees clockwise of Y+ on the XY plane, and Range is the distance from the origin.
Arguments:
By Position | What it does |
---|---|
x |
X coordinate. |
y |
Y coordinate. |
z |
Z coordinate. |
Example 1:
include <BOSL2/std.scad>
aa = xyz_to_altaz(20,30,40);
aa = xyz_to_altaz([40,50,70]);
Table of Contents
Function Index
Topics Index
Cheat Sheet
Tutorials
Basic Modeling:
- constants.scad STD
- transforms.scad STD
- attachments.scad STD
- shapes2d.scad STD
- shapes3d.scad STD
- drawing.scad STD
- masks2d.scad STD
- masks3d.scad STD
- distributors.scad STD
- color.scad STD
- partitions.scad STD
- mutators.scad STD
Advanced Modeling:
Math:
- math.scad STD
- linalg.scad STD
- vectors.scad STD
- coords.scad STD
- geometry.scad STD
- trigonometry.scad STD
Data Management:
- version.scad STD
- comparisons.scad STD
- lists.scad STD
- utility.scad STD
- strings.scad STD
- structs.scad
- fnliterals.scad
Threaded Parts:
Parts:
- ball_bearings.scad
- cubetruss.scad
- gears.scad
- hinges.scad
- joiners.scad
- linear_bearings.scad
- modular_hose.scad
- nema_steppers.scad
- polyhedra.scad
- sliders.scad
- tripod_mounts.scad
- walls.scad
- wiring.scad
STD = Included in std.scad