color.scad
HSV and HSL conversion, rainbow() module for coloring multiple objects. The recolor() and color_this() modules allow you to change the color of previously colored attachable objects.
To use, add the following lines to the beginning of your file:
include <BOSL2/std.scad>
-
-
recolor()
– Sets the color for attachable children and their descendants. [Trans] -
color_this()
– Sets the color for children at the current level only. [Trans] -
rainbow()
– Iterates through a list, displaying children in different colors. [Trans] -
color_overlaps()
– Shows ghostly children, with overlaps highlighted in color. [Trans]
-
Synopsis: Sets the color for attachable children and their descendants. [Trans]
Topics: Attachments
See Also: color_this(), hsl(), hsv()
Usage:
- recolor([c]) CHILDREN;
Description:
Sets the color for attachable children and their descendants, down until another recolor()
or color_this()
. This only works with attachables and you cannot have any color() modules
above it in any parents, only other recolor()
or color_this()
modules. This works by
setting the special $color
variable, which attachable objects make use of to set the color.
Arguments:
By Position | What it does |
---|---|
c |
Color name or RGBA vector. Default: The default color in your color scheme. |
Side Effects:
- Changes the value of
$color
. - Sets the color of child attachments.
Example 1:
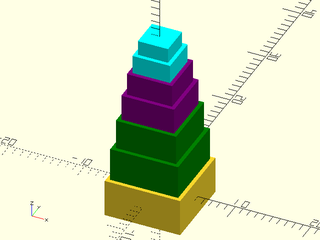
include <BOSL2/std.scad>
cuboid([10,10,5])
recolor("green")attach(TOP,BOT) cuboid([9,9,4.5])
attach(TOP,BOT) cuboid([8,8,4])
recolor("purple") attach(TOP,BOT) cuboid([7,7,3.5])
attach(TOP,BOT) cuboid([6,6,3])
recolor("cyan")attach(TOP,BOT) cuboid([5,5,2.5])
attach(TOP,BOT) cuboid([4,4,2]);
Synopsis: Sets the color for children at the current level only. [Trans]
Topics: Attachments
See Also: recolor(), hsl(), hsv()
Usage:
- color_this([c]) CHILDREN;
Description:
Sets the color for children at one level, reverting to the previous color for further descendants.
This works only with attachables and you cannot have any color() modules above it in any parents,
only recolor() or other color_this() modules. This works using the $color
and $save_color
variables,
which attachable objects make use of to set the color.
Arguments:
By Position | What it does |
---|---|
c |
Color name or RGBA vector. Default: the default color in your color scheme |
Side Effects:
- Changes the value of
$color
and$save_color
. - Sets the color of child attachments.
Example 1:
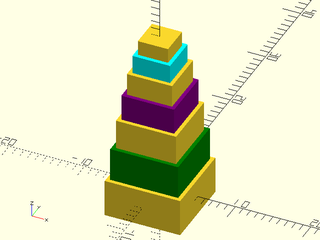
include <BOSL2/std.scad>
cuboid([10,10,5])
color_this("green")attach(TOP,BOT) cuboid([9,9,4.5])
attach(TOP,BOT) cuboid([8,8,4])
color_this("purple") attach(TOP,BOT) cuboid([7,7,3.5])
attach(TOP,BOT) cuboid([6,6,3])
color_this("cyan")attach(TOP,BOT) cuboid([5,5,2.5])
attach(TOP,BOT) cuboid([4,4,2]);
Synopsis: Iterates through a list, displaying children in different colors. [Trans]
Topics: List Handling
Usage:
- rainbow(list,[stride],[maxhues],[shuffle],[seed]) CHILDREN;
Description:
Iterates the list, displaying children in different colors for each list item. The color
is set using the color() module, so this module is not compatible with recolor()
or
color_this()
. This is useful for debugging regions or lists of paths.
Arguments:
By Position | What it does |
---|---|
list |
The list of items to iterate through. |
stride |
Consecutive colors stride around the color wheel divided into this many parts. |
maxhues |
max number of hues to use (to prevent lots of indistinguishable hues) |
shuffle |
if true then shuffle the hues in a random order. Default: false |
seed |
seed to use for shuffle |
Side Effects:
- Sets the color to progressive values along the ROYGBIV spectrum for each item.
- Sets
$idx
to the index of the current item inlist
that we want to show. - Sets
$item
to the current item inlist
that we want to show.
Example 1:
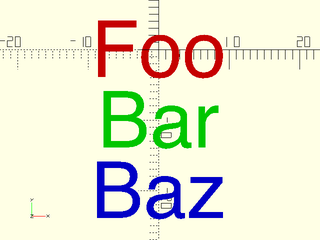
include <BOSL2/std.scad>
rainbow(["Foo","Bar","Baz"]) fwd($idx*10) text(text=$item,size=8,halign="center",valign="center");
Example 2:
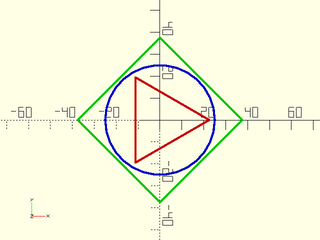
include <BOSL2/std.scad>
rgn = [circle(d=45,$fn=3), circle(d=75,$fn=4), circle(d=50)];
rainbow(rgn) stroke($item, closed=true);
Synopsis: Shows ghostly children, with overlaps highlighted in color. [Trans]
Topics: Debugging
See Also: rainbow(), debug_vnf()
Usage:
- color_overlaps([color]) CHILDREN;
Description:
Displays the given children in ghostly transparent gray, while the places where the they overlap are highlighted with the given color.
Arguments:
By Position | What it does |
---|---|
color |
The color to highlight overlaps with. Default: "red" |
Example 1: 2D Overlaps
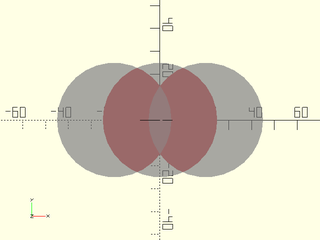
include <BOSL2/std.scad>
color_overlaps() {
circle(d=50);
left(20) circle(d=50);
right(20) circle(d=50);
}
Example 2: 3D Overlaps
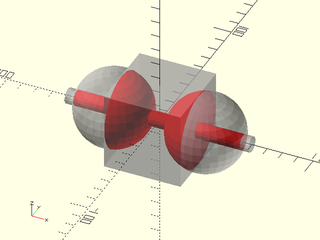
include <BOSL2/std.scad>
color_overlaps() {
cuboid(50);
left(30) sphere(d=50);
right(30) sphere(d=50);
xcyl(d=10,l=120);
}
Synopsis: Sets the color of children to a specified hue, saturation, lightness and optional alpha channel value. [Trans]
Topics: Colors, Colorspace
See Also: hsv(), recolor(), color_this()
Usage:
- hsl(h,[s],[l],[a]) CHILDREN;
- rgb = hsl(h,[s],[l],[a]);
Description:
When called as a function, returns the [R,G,B]
color for the given hue h
, saturation s
, and
lightness l
from the HSL colorspace. If you supply the a
value then you'll get a length 4
list [R,G,B,A]
. When called as a module, sets the color using the color() module to the given
hue h
, saturation s
, and lightness l
from the HSL colorspace.
Arguments:
By Position | What it does |
---|---|
h |
The hue, given as a value between 0 and 360. 0=red, 60=yellow, 120=green, 180=cyan, 240=blue, 300=magenta. |
s |
The saturation, given as a value between 0 and 1. 0 = grayscale, 1 = vivid colors. Default: 1 |
l |
The lightness, between 0 and 1. 0 = black, 0.5 = bright colors, 1 = white. Default: 0.5 |
a |
Specifies the alpha channel as a value between 0 and 1. 0 = fully transparent, 1=opaque. Default: 1 |
Side Effects:
- When called as a module, sets the color of all children.
Example 1:
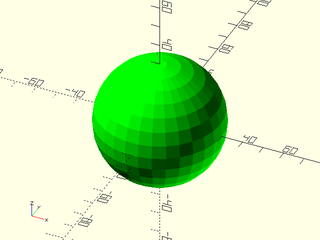
include <BOSL2/std.scad>
hsl(h=120,s=1,l=0.5) sphere(d=60);
Example 2:
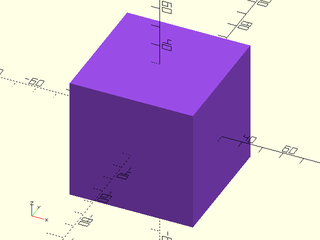
include <BOSL2/std.scad>
rgb = hsl(h=270,s=0.75,l=0.6);
color(rgb) cube(60, center=true);
Synopsis: Sets the color of children to a hue, saturation, value and optional alpha channel value. [Trans]
Topics: Colors, Colorspace
See Also: hsl(), recolor(), color_this()
Usage:
- hsv(h,[s],[v],[a]) CHILDREN;
- rgb = hsv(h,[s],[v],[a]);
Description:
When called as a function, returns the [R,G,B]
color for the given hue h
, saturation s
, and
value v
from the HSV colorspace. If you supply the a
value then you'll get a length 4 list
[R,G,B,A]
. When called as a module, sets the color using the color() module to the given hue
h
, saturation s
, and value v
from the HSV colorspace.
Arguments:
By Position | What it does |
---|---|
h |
The hue, given as a value between 0 and 360. 0=red, 60=yellow, 120=green, 180=cyan, 240=blue, 300=magenta. |
s |
The saturation, given as a value between 0 and 1. 0 = grayscale, 1 = vivid colors. Default: 1 |
v |
The value, between 0 and 1. 0 = darkest black, 1 = bright. Default: 1 |
a |
Specifies the alpha channel as a value between 0 and 1. 0 = fully transparent, 1=opaque. Default: 1 |
Side Effects:
- When called as a module, sets the color of all children.
Example 1:
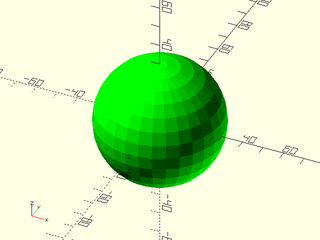
include <BOSL2/std.scad>
hsv(h=120,s=1,v=1) sphere(d=60);
Example 2:
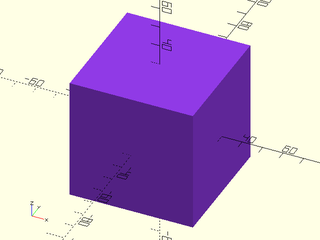
include <BOSL2/std.scad>
rgb = hsv(h=270,s=0.75,v=0.9);
color(rgb) cube(60, center=true);
Table of Contents
Function Index
Topics Index
Cheat Sheet
Tutorials
Basic Modeling:
- constants.scad STD
- transforms.scad STD
- attachments.scad STD
- shapes2d.scad STD
- shapes3d.scad STD
- drawing.scad STD
- masks2d.scad STD
- masks3d.scad STD
- distributors.scad STD
- color.scad STD
- partitions.scad STD
- mutators.scad STD
Advanced Modeling:
Math:
- math.scad STD
- linalg.scad STD
- vectors.scad STD
- coords.scad STD
- geometry.scad STD
- trigonometry.scad STD
Data Management:
- version.scad STD
- comparisons.scad STD
- lists.scad STD
- utility.scad STD
- strings.scad STD
- structs.scad
- fnliterals.scad
Threaded Parts:
Parts:
- ball_bearings.scad
- cubetruss.scad
- gears.scad
- hinges.scad
- joiners.scad
- linear_bearings.scad
- modular_hose.scad
- nema_steppers.scad
- polyhedra.scad
- sliders.scad
- tripod_mounts.scad
- walls.scad
- wiring.scad
STD = Included in std.scad