utility.scad
Functions for type checking, handling undefs, processing function arguments, and testing.
To use, add the following lines to the beginning of your file:
include <BOSL2/std.scad>
-
-
typeof()
– Returns a string representing the type of the value. -
is_type()
– Returns true if the type of 'x' is one of those in the listtypes
. -
is_def()
– Returns true ifx
is notundef
. -
is_str()
– Returns true if the argument is a string. -
is_int()
– Returns true if the argument is an integer. -
all_integer()
– Returns true if all of the numbers in the argument are integers. -
is_nan()
– Return true if the argument is "not a number". -
is_finite()
– Returns true if the argument is a finite number. -
is_range()
– Returns true if the argument is a range. -
valid_range()
– Returns true if the argument is a valid range. -
is_func()
– Returns true if the argument is a function literal. -
is_consistent()
– Returns true if the argument is a list with consistent structure and finite numerical data. -
same_shape()
– Returns true if the argument lists are numeric and of the same shape. -
is_bool_list()
– Returns true if the argument list contains only booleans.
-
-
-
any()
– Returns true if any item in the argument list is true. -
all()
– Returns true if all items in the argument list are true. -
num_true()
– Returns the number of true entries in the arguemnt list.
-
-
-
default()
– Returns a default value if the argument is 'undef', else returns the argument. -
first_defined()
– Returns the first value in the argument list that is not 'undef'. -
one_defined()
– Returns the defined value in the argument list if only a single value is defined. -
num_defined()
– Returns the number of defined values in the the argument list. -
any_defined()
– Returns true if any item in the argument list is notundef
. -
all_defined()
– Returns true if all items in the given array are defined.
-
-
Section: Undef Safe Arithmetic
-
u_add()
– Returns the sum of 2 numbers if both are defined, otherwise returns undef. -
u_sub()
– Returns the difference of 2 numbers if both are defined, otherwise returns undef. -
u_mul()
– Returns the product of 2 numbers if both are defined, otherwise returns undef. -
u_div()
– Returns the quotient of 2 numbers if both are defined, otherwise returns undef.
-
-
Section: Processing Arguments to Functions and Modules
-
get_anchor()
– Returns the correct anchor fromanchor
andcenter
. -
get_radius()
– Given various radii and diameters, returns the most specific radius. -
scalar_vec3()
– Expands a scalar or a list with length less than 3 to a length 3 vector. -
segs()
– Returns the number of sides for a circle given$fn
,$fa
, and$fs
. -
no_children()
– Assert that the calling module does not support children. -
req_children()
– Assert that the calling module requires children. -
no_function()
– Assert that the argument exists only as a module and not as a function. -
no_module()
– Assert that the argument exists only as a function and not as a module. -
deprecate()
– Display a console note that a module is deprecated and suggest a replacement.
-
-
-
assert_approx()
– Assert that a value is approximately what was expected. -
assert_equal()
– Assert that a value is expected. -
shape_compare()
– Compares two child shapes. [Geom]
-
-
Section: C-Style For Loop Helpers
-
looping()
– Returns true if the argument indicates the current C-style loop should continue. -
loop_while()
– Returns true if both arguments indicate the current C-style loop should continue. -
loop_done()
– Returns true if the argument indicates the current C-style loop is finishing.
-
Synopsis: Returns a string representing the type of the value.
Topics: Type Checking
See Also: is_type()
Usage:
- typ = typeof(x);
Description:
Returns a string representing the type of the value. One of "undef", "boolean", "number", "nan", "string", "list", "range", "function" or "invalid". Some malformed "ranges", like '[0:NAN:INF]' and '[0:"a":INF]', may be classified as "undef" or "invalid".
Arguments:
By Position | What it does |
---|---|
x |
value whose type to check |
Example 1:
include <BOSL2/std.scad>
typ = typeof(undef); // Returns: "undef"
typ = typeof(true); // Returns: "boolean"
typ = typeof(42); // Returns: "number"
typ = typeof(NAN); // Returns: "nan"
typ = typeof("foo"); // Returns: "string"
typ = typeof([3,4,5]); // Returns: "list"
typ = typeof([3:1:8]); // Returns: "range"
typ = typeof(function (x,y) x+y); // Returns: "function"
Synopsis: Returns true if the type of 'x' is one of those in the list types
.
Topics: Type Checking
See Also: typeof()
Usage:
- bool = is_type(x, types);
Description:
Returns true if the type of the value x
is one of those given as strings in the list types
.
Valid types are "undef", "boolean", "number", "nan", "string", "list", "range", or "function".
Arguments:
By Position | What it does |
---|---|
x |
The value to check the type of. |
types |
A list of types to check |
Example 1:
include <BOSL2/std.scad>
is_str_or_list = is_type("foo", ["string","list"]); // Returns: true
is_str_or_list2 = is_type([1,2,3], ["string","list"]); // Returns: true
is_str_or_list3 = is_type(2, ["string","list"]); // Returns: false
is_str = is_type("foo", "string"); // Returns: true
is_str2 = is_type([3,4], "string"); // Returns: false
is_str3 = is_type(["foo"], "string"); // Returns: false
is_str4 = is_type(3, "string"); // Returns: false
Synopsis: Returns true if x
is not undef
.
Topics: Type Checking
See Also: typeof(), is_type(), is_str()
Usage:
- bool = is_def(x);
Description:
Returns true if x
is not undef
. False if x==undef
.
Arguments:
By Position | What it does |
---|---|
x |
value to check |
Example 1:
include <BOSL2/std.scad>
bool = is_def(undef); // Returns: false
bool = is_def(false); // Returns: true
bool = is_def(42); // Returns: true
bool = is_def("foo"); // Returns: true
Synopsis: Returns true if the argument is a string.
Topics: Type Checking
See Also: typeof(), is_type(), is_int(), is_def(), is_int()
Usage:
- bool = is_str(x);
Description:
Returns true if x
is a string. A shortcut for is_string()
.
Arguments:
By Position | What it does |
---|---|
x |
value to check |
Example 1:
include <BOSL2/std.scad>
bool = is_str(undef); // Returns: false
bool = is_str(false); // Returns: false
bool = is_str(42); // Returns: false
bool = is_str("foo"); // Returns: true
Alias: is_integer()
Synopsis: Returns true if the argument is an integer.
Topics: Type Checking
See Also: typeof(), is_type(), is_str(), is_def()
Usage:
- bool = is_int(n);
- bool = is_integer(n);
Description:
Returns true if the given value is an integer (it is a number and it rounds to itself).
Arguments:
By Position | What it does |
---|---|
n |
value to check |
Example 1:
include <BOSL2/std.scad>
bool = is_int(undef); // Returns: false
bool = is_int(false); // Returns: false
bool = is_int(42); // Returns: true
bool = is_int("foo"); // Returns: false
Synopsis: Returns true if all of the numbers in the argument are integers.
Topics: Type Checking
Usage:
- bool = all_integer(x);
Description:
If given a number, returns true if the number is a finite integer. If given an empty list, returns false. If given a non-empty list, returns true if every item of the list is an integer. Otherwise, returns false.
Arguments:
By Position | What it does |
---|---|
x |
The value to check. |
Example 1:
include <BOSL2/std.scad>
b = all_integer(true); // Returns: false
b = all_integer("foo"); // Returns: false
b = all_integer(4); // Returns: true
b = all_integer(4.5); // Returns: false
b = all_integer([]); // Returns: false
b = all_integer([3,4,5]); // Returns: true
b = all_integer([3,4.2,5]); // Returns: false
b = all_integer([3,[4,7],5]); // Returns: false
Synopsis: Return true if the argument is "not a number".
Topics: Type Checking
See Also: typeof(), is_type(), is_str(), is_def(), is_int(), is_finite()
Usage:
- bool = is_nan(x);
Description:
Returns true if a given value x
is nan, a floating point value representing "not a number".
Arguments:
By Position | What it does |
---|---|
x |
value to check |
Example 1:
include <BOSL2/std.scad>
bool = is_nan(undef); // Returns: false
bool = is_nan(false); // Returns: false
bool = is_nan(42); // Returns: false
bool = is_nan("foo"); // Returns: false
bool = is_nan(NAN); // Returns: true
Synopsis: Returns true if the argument is a finite number.
Topics: Type Checking
See Also: typeof(), is_type(), is_str(), is_def(), is_int(), is_nan()
Usage:
- bool = is_finite(x);
Description:
Returns true if a given value x
is a finite number.
Arguments:
By Position | What it does |
---|---|
x |
value to check |
Example 1:
include <BOSL2/std.scad>
bool = is_finite(undef); // Returns: false
bool = is_finite(false); // Returns: false
bool = is_finite(42); // Returns: true
bool = is_finite("foo"); // Returns: false
bool = is_finite(NAN); // Returns: false
bool = is_finite(INF); // Returns: false
bool = is_finite(-INF); // Returns: false
Synopsis: Returns true if the argument is a range.
Topics: Type Checking
See Also: typeof(), is_type(), is_str(), is_def(), is_int()
Usage:
- bool = is_range(x);
Description:
Returns true if its argument is a range
Arguments:
By Position | What it does |
---|---|
x |
value to check |
Example 1:
include <BOSL2/std.scad>
bool = is_range(undef); // Returns: false
bool = is_range(false); // Returns: false
bool = is_range(42); // Returns: false
bool = is_range([3,4,5]); // Returns: false
bool = is_range("foo"); // Returns: false
bool = is_range([3:5]); // Returns: true
Synopsis: Returns true if the argument is a valid range.
Topics: Type Checking
See Also: typeof(), is_type(), is_str(), is_def(), is_int(), is_range()
Usage:
- bool = valid_range(x);
Description:
Returns true if its argument is a valid range (deprecated ranges excluded).
Arguments:
By Position | What it does |
---|---|
x |
value to check |
Example 1:
include <BOSL2/std.scad>
bool = is_range(undef); // Returns: false
bool = is_range(false); // Returns: false
bool = is_range(42); // Returns: false
bool = is_range([3,4,5]); // Returns: false
bool = is_range("foo"); // Returns: false
bool = is_range([3:5]); // Returns: true
bool = is_range([3:1]); // Returns: false
Synopsis: Returns true if the argument is a function literal.
Topics: Type Checking, Function Literals
Usage:
- bool = is_func(x);
Description:
Returns true if OpenSCAD supports function literals, and the given item is one.
Arguments:
By Position | What it does |
---|---|
x |
The value to check |
Example 1:
include <BOSL2/std.scad>
f = function (a) a==2;
bool = is_func(f); // Returns: true
Synopsis: Returns true if the argument is a list with consistent structure and finite numerical data.
Topics: Type Checking, Testing
See Also: typeof(), is_type(), is_str(), is_def(), is_int(), is_range(), is_homogeneous()
Usage:
- bool = is_consistent(list, [pattern]);
Description:
Tests whether input is a list of entries which all have the same list structure
and are filled with finite numerical data. You can optionally specify a required
list structure with the pattern argument.
It returns true
for the empty list regardless the value of the pattern
.
Arguments:
By Position | What it does |
---|---|
list |
list to check |
pattern |
optional pattern required to match |
Example 1:
include <BOSL2/std.scad>
is_consistent([3,4,5]); // Returns true
is_consistent([[3,4],[4,5],[6,7]]); // Returns true
is_consistent([[3,4,5],[3,4]]); // Returns false
is_consistent([[3,[3,4,[5]]], [5,[2,9,[9]]]]); // Returns true
is_consistent([[3,[3,4,[5]]], [5,[2,9,9]]]); // Returns false
is_consistent([3,4,5], 0); // Returns true
is_consistent([3,4,undef], 0); // Returns false
is_consistent([[3,4],[4,5]], [1,1]); // Returns true
is_consistent([[3,"a"],[4,true]], [1,undef]); // Returns true
is_consistent([[3,4], 6, [4,5]], [1,1]); // Returns false
is_consistent([[1,[3,4]], [4,[5,6]]], [1,[2,3]]); // Returns true
is_consistent([[1,[3,INF]], [4,[5,6]]], [1,[2,3]]); // Returns false
is_consistent([], [1,[2,3]]); // Returns true
Synopsis: Returns true if the argument lists are numeric and of the same shape.
Topics: Type Checking, Testing
See Also: is_homogeneous(), is_consistent()
Usage:
- bool = same_shape(a,b);
Description:
Tests whether the inputs a
and b
are both numeric and are the same shaped list.
Example 1:
include <BOSL2/std.scad>
same_shape([3,[4,5]],[7,[3,4]]); // Returns true
same_shape([3,4,5], [7,[3,4]]); // Returns false
Synopsis: Returns true if the argument list contains only booleans.
Topics: Boolean Testing
See Also: is_homogeneous(), is_consistent()
Usage:
- check = is_bool_list(list,[length])
Description:
Tests whether input is a list containing only booleans, and optionally checks its length.
Arguments:
By Position | What it does |
---|---|
list |
list to test |
length |
if given, list must be this length |
Synopsis: Returns true if any item in the argument list is true.
Topics: Type Checking
See Also: all(), num_true()
Usage:
- bool = any(l);
- bool = any(l, func); // Requires OpenSCAD 2021.01 or later.
Requirements:
- Requires OpenSCAD 2021.01 or later to use the
func
argument.
Description:
Returns true if any item in list l
evaluates as true.
If func
is given then returns true if the function evaluates as true on any list entry.
Items that evaluate as true include nonempty lists, nonempty strings, and nonzero numbers.
Arguments:
By Position | What it does |
---|---|
l |
The list to test for true items. |
func |
An optional function literal of signature (x), returning bool, to test each list item with. |
Example 1:
include <BOSL2/std.scad>
any([0,false,undef]); // Returns false.
any([1,false,undef]); // Returns true.
any([1,5,true]); // Returns true.
any([[0,0], [0,0]]); // Returns true.
any([[0,0], [1,0]]); // Returns true.
Synopsis: Returns true if all items in the argument list are true.
Topics: Type Checking
See Also: any(), num_true()
Usage:
- bool = all(l);
- bool = all(l, func); // Requires OpenSCAD 2021.01 or later.
Requirements:
- Requires OpenSCAD 2021.01 or later to use the
func
argument.
Description:
Returns true if all items in list l
evaluate as true.
If func
is given then returns true if the function evaluates as true on all list etnries.
Items that evaluate as true include nonempty lists, nonempty strings, and nonzero numbers.
Arguments:
By Position | What it does |
---|---|
l |
The list to test for true items. |
func |
An optional function literal of signature (x), returning bool, to test each list item with. |
Example 1:
include <BOSL2/std.scad>
test1 = all([0,false,undef]); // Returns false.
test2 = all([1,false,undef]); // Returns false.
test3 = all([1,5,true]); // Returns true.
test4 = all([[0,0], [0,0]]); // Returns true.
test5 = all([[0,0], [1,0]]); // Returns true.
test6 = all([[1,1], [1,1]]); // Returns true.
Synopsis: Returns the number of true entries in the arguemnt list.
Topics: Boolean Testing
Usage:
- seq = num_true(l);
- seq = num_true(l, func); // Requires OpenSCAD 2021.01 or later.
Requirements:
- Requires OpenSCAD 2021.01 or later to use the
func=
argument.
Description:
Returns the number of items in l
that evaluate as true. If func
is given then counts
list entries where the function evaluates as true.
Items that evaluate as true include nonempty lists, nonempty strings, and nonzero numbers.
Arguments:
By Position | What it does |
---|---|
l |
The list to test for true items. |
func |
An optional function literal of signature (x), returning bool, to test each list item with. |
Example 1:
include <BOSL2/std.scad>
num1 = num_true([0,false,undef]); // Returns 0.
num2 = num_true([1,false,undef]); // Returns 1.
num3 = num_true([1,5,false]); // Returns 2.
num4 = num_true([1,5,true]); // Returns 3.
num5 = num_true([[0,0], [0,0]]); // Returns 2.
num6 = num_true([[], [1,0]]); // Returns 1.
Synopsis: Returns a default value if the argument is 'undef', else returns the argument.
Topics: Undef Handling
See Also: first_defined(), one_defined(), num_defined()
Usage:
- val = default(val, dflt);
Description:
Returns the value given as v
if it is not undef
.
Otherwise, returns the value of dflt
.
Arguments:
By Position | What it does |
---|---|
v |
Value to pass through if not undef . |
dflt |
Value to return if v is undef . Default: undef |
Synopsis: Returns the first value in the argument list that is not 'undef'.
Topics: Undef Handling
See Also: default(), one_defined(), num_defined(), any_defined(), all_defined()
Usage:
- val = first_defined(v, [recursive]);
Description:
Returns the first item in the list that is not undef
.
If all items are undef
, or list is empty, returns undef
.
Arguments:
By Position | What it does |
---|---|
v |
The list whose items are being checked. |
recursive |
If true, sublists are checked recursively for defined values. The first sublist that has a defined item is returned. Default: false |
Example 1:
include <BOSL2/std.scad>
val = first_defined([undef,7,undef,true]); // Returns: 7
Synopsis: Returns the defined value in the argument list if only a single value is defined.
Topics: Undef Handling
See Also: default(), first_defined(), num_defined(), any_defined(), all_defined()
Usage:
- val = one_defined(vals, names, [dflt])
Description:
Examines the input list vals
and returns the entry which is not undef
.
If more than one entry is not undef
then an error is asserted, specifying
"Must define exactly one of" followed by the names in the names
parameter.
If dflt
is given, and all vals
are undef
, then the value in dflt
is returned.
If dflt
is not given, and all vals
are undef
, then an error is asserted.
Arguments:
By Position | What it does |
---|---|
vals |
The values to return the first one which is not undef . |
names |
A string with comma-separated names for the arguments whose values are passed in vals . |
dflt |
If given, the value returned if all vals are undef . |
Example 1:
include <BOSL2/std.scad>
length1 = one_defined([length,L,l], ["length","L","l"]);
length2 = one_defined([length,L,l], "length,L,l", dflt=1);
Synopsis: Returns the number of defined values in the the argument list.
Topics: Undef Handling
See Also: default(), first_defined(), one_defined(), any_defined(), all_defined()
Usage:
- cnt = num_defined(v);
Description:
Counts how many items in list v
are not undef
.
Example 1:
include <BOSL2/std.scad>
cnt = num_defined([3,7,undef,2,undef,undef,1]); // Returns: 4
Synopsis: Returns true if any item in the argument list is not undef
.
Topics: Undef Handling
See Also: default(), first_defined(), one_defined(), num_defined(), all_defined()
Usage:
- bool = any_defined(v, [recursive]);
Description:
Returns true if any item in the given array is not undef
.
Arguments:
By Position | What it does |
---|---|
v |
The list whose items are being checked. |
recursive |
If true, any sublists are evaluated recursively. Default: false |
Example 1:
include <BOSL2/std.scad>
bool = any_defined([undef,undef,undef]); // Returns: false
bool = any_defined([undef,42,undef]); // Returns: true
bool = any_defined([34,42,87]); // Returns: true
bool = any_defined([undef,undef,[undef]]); // Returns: true
bool = any_defined([undef,undef,[undef]],recursive=true); // Returns: false
bool = any_defined([undef,undef,[42]],recursive=true); // Returns: true
Synopsis: Returns true if all items in the given array are defined.
Topics: Undef Handling
See Also: default(), first_defined(), one_defined(), num_defined()
Usage:
- bool = all_defined(v, [recursive]);
Description:
Returns true if all items in the given array are not undef
.
Arguments:
By Position | What it does |
---|---|
v |
The list whose items are being checked. |
recursive |
If true, any sublists are evaluated recursively. Default: false |
Example 1:
include <BOSL2/std.scad>
bool = all_defined([undef,undef,undef]); // Returns: false
bool = all_defined([undef,42,undef]); // Returns: false
bool = all_defined([34,42,87]); // Returns: true
bool = all_defined([23,34,[undef]]); // Returns: true
bool = all_defined([23,34,[undef]],recursive=true); // Returns: false
bool = all_defined([23,34,[42]],recursive=true); // Returns: true
Synopsis: Returns the sum of 2 numbers if both are defined, otherwise returns undef.
Topics: Undef Handling
See Also: u_sub(), u_mul(), u_div()
Usage:
- x = u_add(a, b);
Description:
Adds a
to b
, returning the result, or undef if either value is undef
.
This emulates the way undefs used to be handled in versions of OpenSCAD before 2020.
Arguments:
By Position | What it does |
---|---|
a |
First value. |
b |
Second value. |
Synopsis: Returns the difference of 2 numbers if both are defined, otherwise returns undef.
Topics: Undef Handling
See Also: u_add(), u_mul(), u_div()
Usage:
- x = u_sub(a, b);
Description:
Subtracts b
from a
, returning the result, or undef if either value is undef
.
This emulates the way undefs used to be handled in versions of OpenSCAD before 2020.
Arguments:
By Position | What it does |
---|---|
a |
First value. |
b |
Second value. |
Synopsis: Returns the product of 2 numbers if both are defined, otherwise returns undef.
Topics: Undef Handling
See Also: u_add(), u_sub(), u_div()
Usage:
- x = u_mul(a, b);
Description:
Multiplies a
by b
, returning the result, or undef if either value is undef
.
This emulates the way undefs used to be handled in versions of OpenSCAD before 2020.
Arguments:
By Position | What it does |
---|---|
a |
First value. |
b |
Second value. |
Synopsis: Returns the quotient of 2 numbers if both are defined, otherwise returns undef.
Topics: Undef Handling
See Also: u_add(), u_sub(), u_mul()
Usage:
- x = u_div(a, b);
Description:
Divides a
by b
, returning the result, or undef if either value is undef
.
This emulates the way undefs used to be handled in versions of OpenSCAD before 2020.
Arguments:
By Position | What it does |
---|---|
a |
First value. |
b |
Second value. |
Synopsis: Returns the correct anchor from anchor
and center
.
Topics: Argument Handling
See Also: get_radius()
Usage:
- anchr = get_anchor(anchor,center,[uncentered],[dflt]);
Description:
Calculated the correct anchor from anchor
and center
. In order:
- If
center
is notundef
andcenter
evaluates as true, thenCENTER
([0,0,0]
) is returned. - Otherwise, if
center
is notundef
andcenter
evaluates as false, then the value ofuncentered
is returned. - Otherwise, if
anchor
is notundef
, then the value ofanchor
is returned. - Otherwise, the value of
dflt
is returned.
This ordering ensures that center
will override anchor
.
Arguments:
By Position | What it does |
---|---|
anchor |
The anchor name or vector. |
center |
If not undef , this overrides the value of anchor . |
uncentered |
The value to return if center is not undef and evaluates as false. Default: BOTTOM |
dflt |
The default value to return if both anchor and center are undef . Default: CENTER
|
Example 1:
include <BOSL2/std.scad>
anchr1 = get_anchor(undef, undef, BOTTOM, TOP); // Returns: [0, 0, 1] (TOP)
anchr2 = get_anchor(RIGHT, undef, BOTTOM, TOP); // Returns: [1, 0, 0] (RIGHT)
anchr3 = get_anchor(undef, false, BOTTOM, TOP); // Returns: [0, 0,-1] (BOTTOM)
anchr4 = get_anchor(RIGHT, false, BOTTOM, TOP); // Returns: [0, 0,-1] (BOTTOM)
anchr5 = get_anchor(undef, true, BOTTOM, TOP); // Returns: [0, 0, 0] (CENTER)
anchr6 = get_anchor(RIGHT, true, BOTTOM, TOP); // Returns: [0, 0, 0] (CENTER)
Synopsis: Given various radii and diameters, returns the most specific radius.
Topics: Argument Handling
See Also: get_anchor()
Usage:
- r = get_radius([r1=], [r2=], [r=], [d1=], [d2=], [d=], [dflt=]);
Description:
Given various radii and diameters, returns the most specific radius. If a diameter is most
specific, returns half its value, giving the radius. If no radii or diameters are defined,
returns the value of dflt
. Value specificity order is r1
, r2
, d1
, d2
, r
, d
,
then dflt
. Only one of r1
, r2
, d1
, or d2
can be defined at once, or else it errors
out, complaining about conflicting radius/diameter values.
Arguments:
By Position | What it does |
---|---|
r1 |
Most specific radius. |
r2 |
Second most specific radius. |
r |
Most general radius. |
d1 |
Most specific diameter. |
d2 |
Second most specific diameter. |
d |
Most general diameter. |
dflt |
Value to return if all other values given are undef . |
Example 1:
include <BOSL2/std.scad>
r = get_radius(r1=undef, r=undef, dflt=undef); // Returns: undef
r = get_radius(r1=undef, r=undef, dflt=1); // Returns: 1
r = get_radius(r1=undef, r=6, dflt=1); // Returns: 6
r = get_radius(r1=7, r=6, dflt=1); // Returns: 7
r = get_radius(r1=undef, r2=8, r=6, dflt=1); // Returns: 8
r = get_radius(r1=undef, r2=8, d=6, dflt=1); // Returns: 8
r = get_radius(r1=undef, d=6, dflt=1); // Returns: 3
r = get_radius(d1=7, d=6, dflt=1); // Returns: 3.5
r = get_radius(d1=7, d2=8, d=6, dflt=1); // Returns: 3.5
r = get_radius(d1=undef, d2=8, d=6, dflt=1); // Returns: 4
r = get_radius(r1=8, d=6, dflt=1); // Returns: 8
Synopsis: Expands a scalar or a list with length less than 3 to a length 3 vector.
Topics: Argument Handling
See Also: get_anchor(), get_radius(), force_list()
Usage:
- vec = scalar_vec3(v, [dflt]);
Description:
This is expands a scalar or a list with length less than 3 to a length 3 vector in the
same way that OpenSCAD expands short vectors in some contexts, e.g. cube(10) or rotate([45,90]).
If v
is a scalar, and dflt==undef
, returns [v, v, v]
.
If v
is a scalar, and dflt!=undef
, returns [v, dflt, dflt]
.
If v
is a vector and dflt is defined, returns the first 3 items, with any missing values replaced by dflt
.
If v
is a vector and dflt is undef, returns the first 3 items, with any missing values replaced by 0.
If v
is undef
, returns undef
.
Arguments:
By Position | What it does |
---|---|
v |
Value to return vector from. |
dflt |
Default value to set empty vector parts from. |
Example 1:
include <BOSL2/std.scad>
vec = scalar_vec3(undef); // Returns: undef
vec = scalar_vec3(10); // Returns: [10,10,10]
vec = scalar_vec3(10,1); // Returns: [10,1,1]
vec = scalar_vec3([10,10],1); // Returns: [10,10,1]
vec = scalar_vec3([10,10]); // Returns: [10,10,0]
vec = scalar_vec3([10]); // Returns: [10,0,0]
Synopsis: Returns the number of sides for a circle given $fn
, $fa
, and $fs
.
Topics: Geometry
Usage:
- sides = segs(r);
Description:
Calculate the standard number of sides OpenSCAD would give a circle based on $fn
, $fa
, and $fs
.
Arguments:
By Position | What it does |
---|---|
r |
Radius of circle to get the number of segments for. |
Example 1:
include <BOSL2/std.scad>
$fn=12; sides=segs(10); // Returns: 12
$fa=2; $fs=3; sides=segs(10); // Returns: 21
Synopsis: Assert that the calling module does not support children.
Topics: Error Checking
See Also: no_function(), no_module(), req_children()
Usage:
- no_children($children);
Description:
Assert that the calling module does not support children. Prints an error message to this effect and fails if children are present, as indicated by its argument.
Arguments:
By Position | What it does |
---|---|
$children |
number of children the module has. |
Example 1:
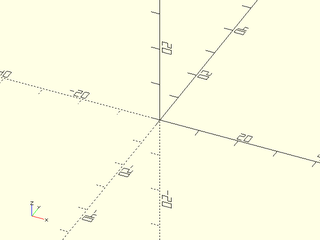
include <BOSL2/std.scad>
module foo() {
no_children($children);
}
Synopsis: Assert that the calling module requires children.
Topics: Error Checking
See Also: no_function(), no_module()
Usage:
- req_children($children);
Description:
Assert that the calling module requires children. Prints an error message and fails if no children are present as indicated by its argument.
Arguments:
By Position | What it does |
---|---|
$children |
number of children the module has. |
Example 1:
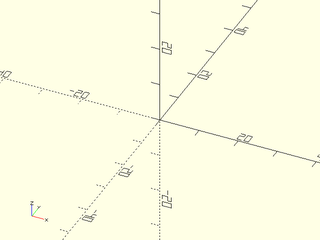
include <BOSL2/std.scad>
module foo() {
req_children($children);
}
Synopsis: Assert that the argument exists only as a module and not as a function.
Topics: Error Checking
See Also: no_children(), no_module()
Usage:
- dummy = no_function(name)
Description:
Asserts that the function, "name", only exists as a module.
Example 1:
include <BOSL2/std.scad>
x = no_function("foo");
Synopsis: Assert that the argument exists only as a function and not as a module.
Topics: Error Checking
See Also: no_children(), no_function()
Usage:
- no_module();
Description:
Asserts that the called module exists only as a function.
Example 1:
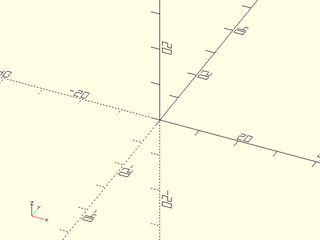
include <BOSL2/std.scad>
module foo() { no_module(); }
Synopsis: Display a console note that a module is deprecated and suggest a replacement.
Topics: Error Checking
See Also: no_function(), no_module()
Usage:
- deprecate(new_name);
Description:
Display info that the current module is deprecated and you should switch to a new name
Arguments:
By Position | What it does |
---|---|
new_name |
name of the new module that replaces the old one |
Synopsis: Assert that a value is approximately what was expected.
Topics: Error Checking, Debugging
See Also: no_children(), no_function(), no_module(), assert_equal()
Usage:
- assert_approx(got, expected, [info]);
Description:
Tests if the value gotten is what was expected, plus or minus 1e-9. If not, then the expected and received values are printed to the console and an assertion is thrown to stop execution. Returns false if both 'got' and 'expected' are 'nan'.
Arguments:
By Position | What it does |
---|---|
got |
The value actually received. |
expected |
The value that was expected. |
info |
Extra info to print out to make the error clearer. |
Example 1:
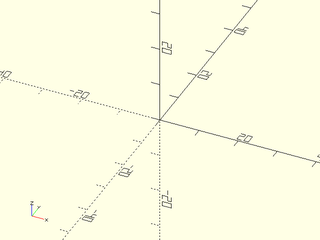
include <BOSL2/std.scad>
assert_approx(1/3, 0.333333333333333, str("number=",1,", denom=",3));
Synopsis: Assert that a value is expected.
Topics: Error Checking, Debugging
See Also: no_children(), no_function(), no_module(), assert_approx()
Usage:
- assert_equal(got, expected, [info]);
Description:
Tests if the value gotten is what was expected. If not, then the expected and received values are printed to the console and an assertion is thrown to stop execution. Returns true if both 'got' and 'expected' are 'nan'.
Arguments:
By Position | What it does |
---|---|
got |
The value actually received. |
expected |
The value that was expected. |
info |
Extra info to print out to make the error clearer. |
Example 1:
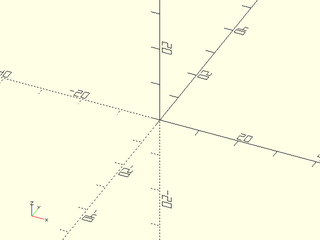
include <BOSL2/std.scad>
assert_approx(3*9, 27, str("a=",3,", b=",9));
Synopsis: Compares two child shapes. [Geom]
Topics: Error Checking, Debugging, Testing
See Also: assert_approx(), assert_equal()
Usage:
- shape_compare([eps]) {TEST_SHAPE; EXPECTED_SHAPE;}
Description:
Compares two child shapes, returning empty geometry if they are very nearly the same shape and size. Returns the differential geometry if they are not quite the same shape and size.
Arguments:
By Position | What it does |
---|---|
eps |
The surface of the two shapes must be within this size of each other. Default: 1/1024 |
Example 1:
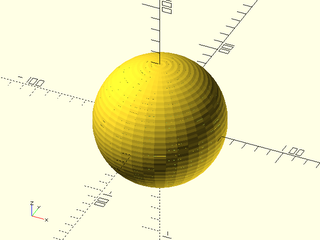
include <BOSL2/std.scad>
$fn=36;
shape_compare() {
sphere(d=100);
rotate_extrude() right_half(planar=true) circle(d=100);
}
You can use a list comprehension with a C-style for loop to iteratively make a calculation.
The syntax is: [for (INIT; CONDITION; NEXT) RETVAL]
where:
- INIT is zero or more
let()
style assignments that are evaluated exactly one time, before the first loop. - CONDITION is an expression evaluated at the start of each loop. If true, continues with the loop.
- RETVAL is an expression that returns a list item for each loop.
- NEXT is one or more
let()
style assignments that is evaluated at the end of each loop.
Since the INIT phase is only run once, and the CONDITION and RETVAL expressions cannot update
variables, that means that only the NEXT phase can be used for iterative calculations.
Unfortunately, the NEXT phase runs after the RETVAL expression, which means that you need
to run the loop one extra time to return the final value. This tends to make the loop code
look rather ugly. The looping()
, loop_while()
and loop_done()
functions
can make this somewhat more legible.
function flat_sum(l) = [
for (
i = 0,
total = 0,
state = 0;
looping(state);
state = loop_while(state, i < len(l)),
total = total +
loop_done(state) ? 0 :
let( x = l[i] )
is_list(x) ? flat_sum(x) : x,
i = i + 1
) if (loop_done(state)) total;
].x;
Synopsis: Returns true if the argument indicates the current C-style loop should continue.
Topics: Iteration
See Also: loop_while(), loop_done()
Usage:
- bool = looping(state);
Description:
Returns true if the state
value indicates the current loop should continue. This is useful
when using C-style for loops to iteratively calculate a value. Used with loop_while()
and
loop_done()
. See Looping Helpers for an example.
Arguments:
By Position | What it does |
---|---|
state |
The loop state value. |
Synopsis: Returns true if both arguments indicate the current C-style loop should continue.
Topics: Iteration
See Also: looping(), loop_done()
Usage:
- state = loop_while(state, continue);
Description:
Given the current state
, and a boolean continue
that indicates if the loop should still be
continuing, returns the updated state value for the the next loop. This is useful when using
C-style for loops to iteratively calculate a value. Used with looping()
and loop_done()
.
See Looping Helpers for an example.
Arguments:
By Position | What it does |
---|---|
state |
The loop state value. |
continue |
A boolean value indicating whether the current loop should progress. |
Synopsis: Returns true if the argument indicates the current C-style loop is finishing.
Topics: Iteration
See Also: looping(), loop_while()
Usage:
- bool = loop_done(state);
Description:
Returns true if the state
value indicates the loop is finishing. This is useful when using
C-style for loops to iteratively calculate a value. Used with looping()
and loop_while()
.
See Looping Helpers for an example.
Arguments:
By Position | What it does |
---|---|
state |
The loop state value. |
Table of Contents
Function Index
Topics Index
Cheat Sheet
Tutorials
Basic Modeling:
- constants.scad STD
- transforms.scad STD
- attachments.scad STD
- shapes2d.scad STD
- shapes3d.scad STD
- drawing.scad STD
- masks2d.scad STD
- masks3d.scad STD
- distributors.scad STD
- color.scad STD
- partitions.scad STD
- mutators.scad STD
Advanced Modeling:
Math:
- math.scad STD
- linalg.scad STD
- vectors.scad STD
- coords.scad STD
- geometry.scad STD
- trigonometry.scad STD
Data Management:
- version.scad STD
- comparisons.scad STD
- lists.scad STD
- utility.scad STD
- strings.scad STD
- structs.scad
- fnliterals.scad
Threaded Parts:
Parts:
- ball_bearings.scad
- cubetruss.scad
- gears.scad
- hinges.scad
- joiners.scad
- linear_bearings.scad
- modular_hose.scad
- nema_steppers.scad
- polyhedra.scad
- sliders.scad
- tripod_mounts.scad
- walls.scad
- wiring.scad
STD = Included in std.scad