bottlecaps.scad
Bottle caps and necks for PCO18XX standard plastic beverage bottles, and SPI standard bottle necks.
To use, add the following lines to the beginning of your file:
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
-
Section: PCO-1810 Bottle Threading
-
pco1810_neck()
– Creates a neck for a PCO1810 standard bottle. [Geom] -
pco1810_cap()
– Creates a cap for a PCO1810 standard bottle. [Geom]
-
-
Section: PCO-1881 Bottle Threading
-
pco1881_neck()
– Creates a neck for a PCO1881 standard bottle. [Geom] -
pco1881_cap()
– Creates a cap for a PCO1881 standard bottle. [Geom]
-
-
Section: Generic Bottle Connectors
-
generic_bottle_neck()
– Creates a generic neck for a bottle. [Geom] -
generic_bottle_cap()
– Creates a generic cap for a bottle. [Geom] -
bottle_adapter_neck_to_cap()
– Creates a generic adaptor between a neck and a cap. [Geom] -
bottle_adapter_cap_to_cap()
– Creates a generic adaptor between a cap and a cap. [Geom] -
bottle_adapter_neck_to_neck()
– Creates a generic adaptor between a neck and a neck. [Geom]
-
-
-
sp_neck()
– Creates an SPI threaded bottle neck. [Geom] -
sp_cap()
– Creates an SPI threaded bottle cap. [Geom] -
sp_diameter()
– Returns the base diameter of an SPI bottle neck from the nominal diameter and type number.
-
Synopsis: Creates a neck for a PCO1810 standard bottle. [Geom]
See Also: pco1810_cap()
Usage:
- pco1810_neck([wall]) [ATTACHMENTS];
Description:
Creates an approximation of a standard PCO-1810 threaded beverage bottle neck.
Arguments:
By Position | What it does |
---|---|
wall |
Wall thickness in mm. |
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Extra Anchors:
Anchor Name | Position |
---|---|
"tamper-ring" | Centered at the top of the anti-tamper ring channel. |
"support-ring" | Centered at the bottom of the support ring. |
Example 1:
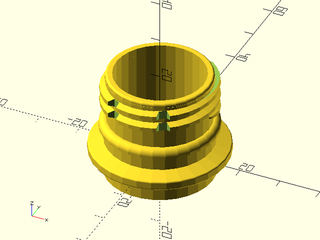
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
pco1810_neck();
Example 2: Standard Anchors
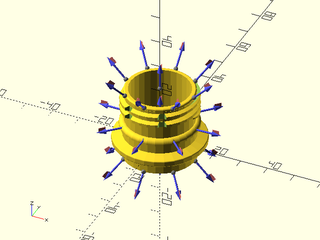
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
pco1810_neck() show_anchors(custom=false);
Example 3: Custom Named Anchors
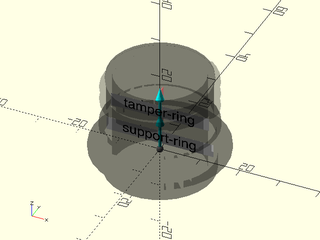
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
expose_anchors(0.3)
pco1810_neck()
show_anchors(std=false);
Synopsis: Creates a cap for a PCO1810 standard bottle. [Geom]
See Also: pco1810_neck()
Usage:
- pco1810_cap([h], [r|d], [wall], [texture]) [ATTACHMENTS];
Description:
Creates a basic cap for a PCO1810 threaded beverage bottle.
Arguments:
By Position | What it does |
---|---|
h |
The height of the cap. |
r |
Outer radius of the cap. |
d |
Outer diameter of the cap. |
wall |
Wall thickness in mm. |
texture |
The surface texture of the cap. Valid values are "none", "knurled", or "ribbed". Default: "none" |
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Extra Anchors:
Anchor Name | Position |
---|---|
"inside-top" | Centered on the inside top of the cap. |
Example 1:
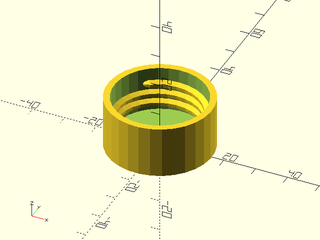
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
pco1810_cap();
Example 2:
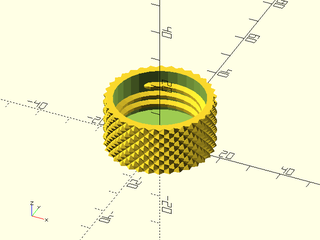
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
pco1810_cap(texture="knurled");
Example 3:
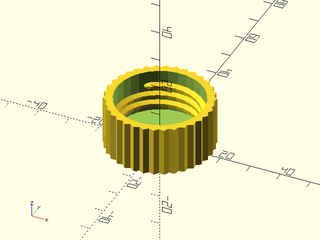
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
pco1810_cap(texture="ribbed");
Example 4: Standard Anchors
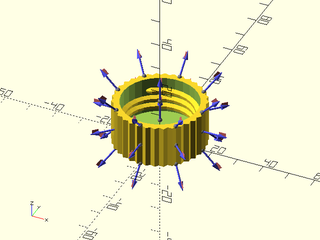
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
pco1810_cap(texture="ribbed") show_anchors(custom=false);
Example 5: Custom Named Anchors
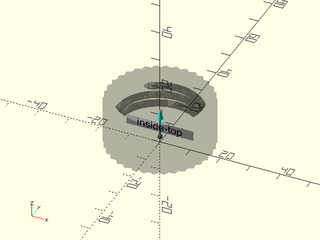
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
expose_anchors(0.3)
pco1810_cap(texture="ribbed")
show_anchors(std=false);
Synopsis: Creates a neck for a PCO1881 standard bottle. [Geom]
See Also: pco1881_cap()
Usage:
- pco1881_neck([wall]) [ATTACHMENTS];
Description:
Creates an approximation of a standard PCO-1881 threaded beverage bottle neck.
Arguments:
By Position | What it does |
---|---|
wall |
Wall thickness in mm. |
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Extra Anchors:
Anchor Name | Position |
---|---|
"tamper-ring" | Centered at the top of the anti-tamper ring channel. |
"support-ring" | Centered at the bottom of the support ring. |
Example 1:
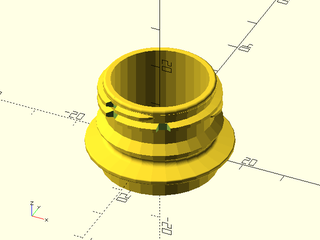
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
pco1881_neck();
Example 2:
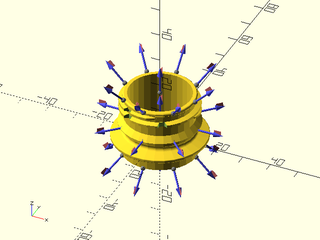
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
pco1881_neck() show_anchors(custom=false);
Example 3:
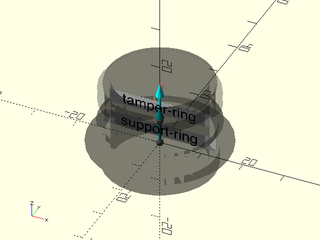
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
expose_anchors(0.3)
pco1881_neck()
show_anchors(std=false);
Synopsis: Creates a cap for a PCO1881 standard bottle. [Geom]
See Also: pco1881_neck()
Usage:
- pco1881_cap(wall, [texture]) [ATTACHMENTS];
Description:
Creates a basic cap for a PCO1881 threaded beverage bottle.
Arguments:
By Position | What it does |
---|---|
wall |
Wall thickness in mm. |
texture |
The surface texture of the cap. Valid values are "none", "knurled", or "ribbed". Default: "none" |
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Extra Anchors:
Anchor Name | Position |
---|---|
"inside-top" | Centered on the inside top of the cap. |
Example 1:
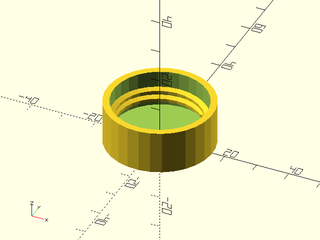
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
pco1881_cap();
Example 2:
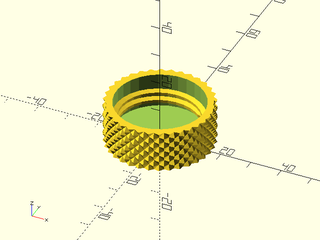
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
pco1881_cap(texture="knurled");
Example 3:
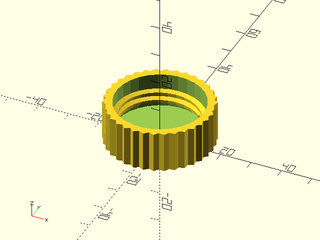
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
pco1881_cap(texture="ribbed");
Example 4: Standard Anchors
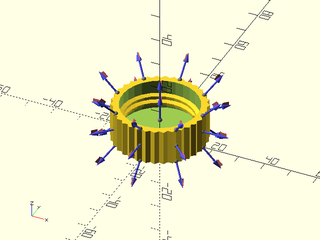
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
pco1881_cap(texture="ribbed") show_anchors(custom=false);
Example 5: Custom Named Anchors
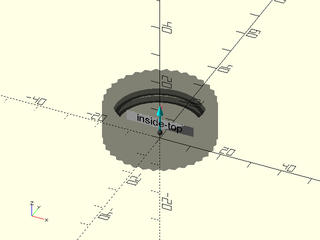
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
expose_anchors(0.5)
pco1881_cap(texture="ribbed")
show_anchors(std=false);
Synopsis: Creates a generic neck for a bottle. [Geom]
See Also: generic_bottle_cap()
Usage:
- generic_bottle_neck([wall], ...) [ATTACHMENTS];
Description:
Creates a bottle neck given specifications.
Arguments:
By Position | What it does |
---|---|
wall |
distance between ID and any wall that may be below the support |
By Name | What it does |
---|---|
neck_d |
Outer diameter of neck without threads |
id |
Inner diameter of neck |
thread_od |
Outer diameter of thread |
height |
Height of neck above support |
support_d |
Outer diameter of support ring. Set to 0 for no support. |
pitch |
Thread pitch |
round_supp |
True to round the lower edge of the support ring |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Extra Anchors:
Anchor Name | Position |
---|---|
"support-ring" | Centered at the bottom of the support ring. |
Example 1:
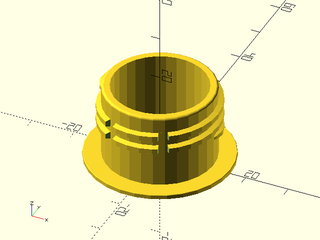
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
generic_bottle_neck();
Synopsis: Creates a generic cap for a bottle. [Geom]
See Also: generic_bottle_neck(), sp_cap()
Usage:
- generic_bottle_cap(wall, [texture], ...) [ATTACHMENTS];
Description:
Creates a basic threaded cap given specifications. You must give exactly two of thread_od
, neck_od
and thread_depth
to
specify the thread geometry. Note that most glass bottles conform to the SPI standard and caps for them may be more easily produced using sp_cap()
.
Arguments:
By Position | What it does |
---|---|
wall |
Wall thickness. Default: 2 |
texture |
The surface texture of the cap. Valid values are "none", "knurled", or "ribbed". Default: "none" |
By Name | What it does |
---|---|
height |
Interior height of the cap |
thread_od |
Outer diameter of the threads |
neck_od |
Outer diameter of neck |
thread_depth |
Depth of the threads |
tolerance |
Extra space to add to the outer diameter of threads and neck. Applied to radius. Default: 0.2 |
flank_angle |
Angle of taper on threads. Default: 15 |
pitch |
Thread pitch. Default: 4 |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Extra Anchors:
Anchor Name | Position |
---|---|
"inside-top" | Centered on the inside top of the cap. |
Example 1:
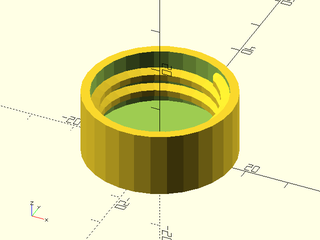
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
generic_bottle_cap(thread_depth=2,neck_od=INCH,height=INCH/2);
Example 2:
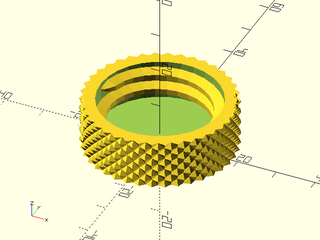
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
generic_bottle_cap(texture="knurled",neck_od=25,thread_od=30,height=10);
Example 3:
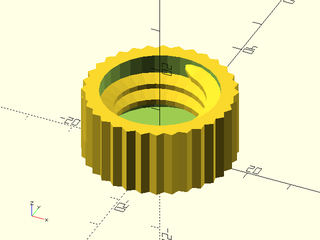
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
generic_bottle_cap(texture="ribbed",thread_depth=3,thread_od=25,height=13);
Synopsis: Creates a generic adaptor between a neck and a cap. [Geom]
See Also: bottle_adapter_neck_to_neck()
Usage:
- bottle_adapter_neck_to_cap(wall, [texture], ...) [ATTACHMENTS];
Description:
Creates a threaded neck to cap adapter
Arguments:
By Position | What it does |
---|---|
wall |
Thickness of wall between neck and cap when d=0. Leave undefined to have the outside of the tube go from the OD of the neck support ring to the OD of the cap. Default: undef |
texture |
The surface texture of the cap. Valid values are "none", "knurled", or "ribbed". Default: "none" |
cap_wall |
Wall thickness of the cap in mm. |
cap_h |
Interior height of the cap in mm. |
cap_thread_depth |
Cap thread depth. Default: 2.34 |
tolerance |
Extra space to add to the outer diameter of threads and neck in mm. Applied to radius. |
cap_neck_od |
Inner diameter of the cap threads. |
cap_neck_id |
Inner diameter of the hole through the cap. |
cap_thread_taper |
Angle of taper on threads. |
cap_thread_pitch |
Thread pitch in mm |
neck_d |
Outer diameter of neck w/o threads |
neck_id |
Inner diameter of neck |
neck_thread_od |
27.2 |
neck_h |
Height of neck down to support ring |
neck_thread_pitch |
Thread pitch in mm. |
neck_support_od |
Outer diameter of neck support ring. Leave undefined to set equal to OD of cap. Set to 0 for no ring. Default: undef |
d |
Distance between bottom of neck and top of cap |
taper_lead_in |
Length to leave straight before tapering on tube between neck and cap if exists. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Example 1:
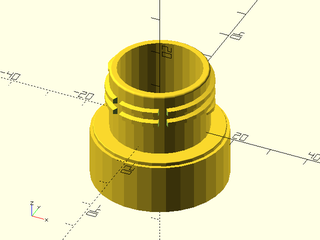
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
bottle_adapter_neck_to_cap();
Synopsis: Creates a generic adaptor between a cap and a cap. [Geom]
See Also: bottle_adapter_neck_to_cap(), bottle_adapter_neck_to_neck()
Usage:
- bottle_adapter_cap_to_cap(wall, [texture]) [ATTACHMENTS];
Description:
Creates a threaded cap to cap adapter.
Arguments:
By Position | What it does |
---|---|
wall |
Wall thickness in mm. |
texture |
The surface texture of the cap. Valid values are "none", "knurled", or "ribbed". Default: "none" |
cap_h1 |
Interior height of top cap. |
cap_thread_depth1 |
Thread depth on top cap. Default: 2.34 |
tolerance |
Extra space to add to the outer diameter of threads and neck in mm. Applied to radius. |
cap_neck_od1 |
Inner diameter of threads on top cap. |
cap_thread_pitch1 |
Thread pitch of top cap in mm. |
cap_h2 |
Interior height of bottom cap. Leave undefined to duplicate cap_h1. |
cap_thread_depth2 |
Thread depth on bottom cap. Default: same as cap_thread_depth1 |
cap_neck_od2 |
Inner diameter of threads on top cap. Leave undefined to duplicate cap_neck_od1. |
cap_thread_pitch2 |
Thread pitch of bottom cap in mm. Leave undefinced to duplicate cap_thread_pitch1. |
d |
Distance between caps. |
neck_id1 |
Inner diameter of cutout in top cap. |
neck_id2 |
Inner diameter of cutout in bottom cap. |
taper_lead_in |
Length to leave straight before tapering on tube between caps if exists. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Example 1:
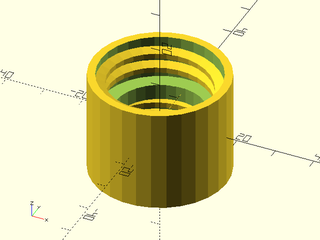
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
bottle_adapter_cap_to_cap();
Synopsis: Creates a generic adaptor between a neck and a neck. [Geom]
See Also: bottle_adapter_neck_to_cap(), bottle_adapter_cap_to_cap()
Usage:
- bottle_adapter_neck_to_neck(...) [ATTACHMENTS];
Description:
Creates a threaded neck to neck adapter.
Arguments:
By Position | What it does |
---|---|
d |
Distance between bottoms of necks |
neck_od1 |
Outer diameter of top neck w/o threads |
neck_id1 |
Inner diameter of top neck |
thread_od1 |
Outer diameter of threads on top neck |
height1 |
Height of top neck above support ring. |
support_od1 |
Outer diameter of the support ring on the top neck. Set to 0 for no ring. |
thread_pitch1 |
Thread pitch of top neck. |
neck_od2 |
Outer diameter of bottom neck w/o threads. Leave undefined to duplicate neck_od1 |
neck_id2 |
Inner diameter of bottom neck. Leave undefined to duplicate neck_id1 |
thread_od2 |
Outer diameter of threads on bottom neck. Leave undefined to duplicate thread_od1 |
height2 |
Height of bottom neck above support ring. Leave undefined to duplicate height1 |
support_od2 |
Outer diameter of the support ring on bottom neck. Set to 0 for no ring. Leave undefined to duplicate support_od1 |
pitch2 |
Thread pitch of bottom neck. Leave undefined to duplicate thread_pitch1 |
taper_lead_in |
Length to leave straight before tapering on tube between necks if exists. |
wall |
Thickness of tube wall between necks. Leave undefined to match outer diameters with the neckODs/supportODs. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Example 1:
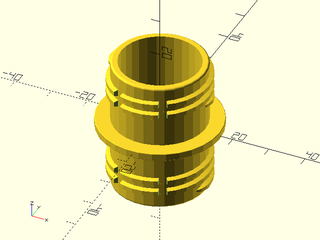
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
bottle_adapter_neck_to_neck();
Synopsis: Creates an SPI threaded bottle neck. [Geom]
See Also: sp_cap()
Usage:
- sp_neck(diam, type, wall|id=, [style=], [bead=]) [ATTACHMENTS];
Description:
Make a SPI (Society of Plastics Industry) threaded bottle neck. You must supply the nominal outer diameter of the threads and the thread type, one of 400, 410 and 415. The 400 type neck has 360 degrees of thread, the 410 neck has 540 degrees of thread, and the 415 neck has 720 degrees of thread. You can also choose between the L style thread, which is symmetric and the M style thread, which is an asymmetric buttress thread. The M style may be good for 3d printing if printed with the flat face up. You can specify the wall thickness (measured from the base of the threads) or the inner diameter, and you can specify an optional bead at the base of the threads.
Arguments:
By Position | What it does |
---|---|
diam |
nominal outer diameter of threads |
type |
thread type, one of 400, 410 and 415 |
wall |
wall thickness |
By Name | What it does |
---|---|
id |
inner diameter |
style |
Either "L" or "M" to specify the thread style. Default: "L" |
bead |
if true apply a bad to the neck. Default: false |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Example 1:
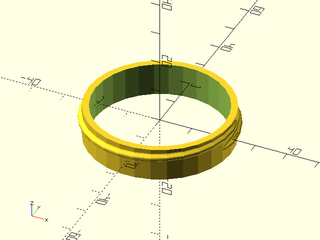
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
sp_neck(48,400,2);
Example 2:
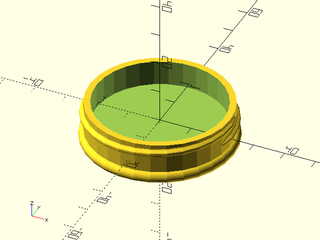
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
sp_neck(48,400,2,bead=true);
Example 3:
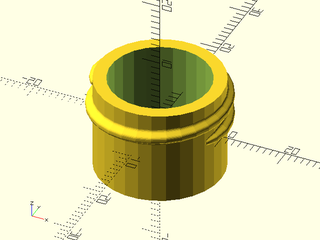
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
sp_neck(22,410,2);
Example 4:
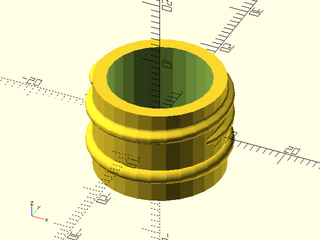
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
sp_neck(22,410,2,bead=true);
Example 5:
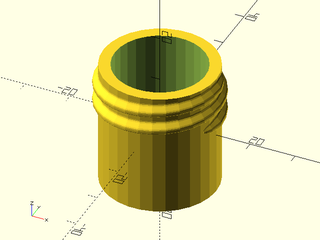
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
sp_neck(28,415,id=20,style="M");
Example 6:
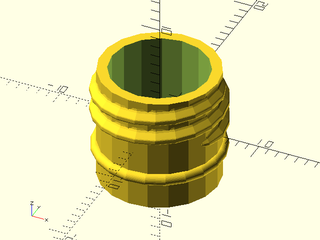
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
sp_neck(13,415,wall=1,style="M",bead=true);
Synopsis: Creates an SPI threaded bottle cap. [Geom]
See Also: sp_neck()
Usage:
- sp_cap(diam, type, wall, [style=], [top_adj=], [bot_adj=], [texture=], [$slop]) [ATTACHMENTS];
Description:
Make a SPI (Society of Plastics Industry) threaded bottle neck. You must supply the nominal outer diameter of the threads and the thread type, one of 400, 410 and 415. The 400 type neck has 360 degrees of thread, the 410 neck has 540 degrees of thread, and the 415 neck has 720 degrees of thread. You can also choose between the L style thread, which is symmetric and the M style thread, which is an asymmetric buttress thread. Note that it is OK to mix styles, so you can put an L-style cap onto an M-style neck.
The 410 and 415 caps have very long unthreaded sections at the bottom. The bot_adj parameter specifies an amount to reduce that bottom extension, which might be necessary if the cap bottoms out on the bead. Be careful that you don't shrink past the threads, especially if making adjustments to 400 caps which have a very small bottom extension. These caps often contain a cardboard or foam sealer disk, which can be as much as 1mm thick, and would cause the cap to stop in a higher position.
You can also adjust the space between the top of the cap and the threads using top_adj. This will change how the threads engage when the cap is fully seated.
The inner diameter of the cap is set to allow 10% of the thread depth in clearance. The diameter
is further increased by 2 * $slop
so you can increase clearance if necessary.
Note: there is a published SPI standard for necks, but absolutely nothing for caps. This cap module was designed based on the neck standard to mate reasonably well, but if you find ways that it does the wrong thing, file a report.
Arguments:
By Position | What it does |
---|---|
diam |
nominal outer diameter of threads |
type |
thread type, one of 400, 410 and 415 |
wall |
wall thickness |
By Name | What it does |
---|---|
style |
Either "L" or "M" to specify the thread style. Default: "L" |
top_adj |
Amount to reduce top space in the cap, which means it doesn't screw down as far. Default: 0 |
bot_adj |
Amount to reduce extension of cap at the bottom, which also means it doesn't screw down as far. Default: 0 |
texture |
texture for outside of cap, one of "knurled", "ribbed" or "none. Default: "none" |
$slop |
Increase inner diameter by 2 * $slop . |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0
|
orient |
Vector to rotate top towards, after spin. See orient. Default: UP
|
Example 1:
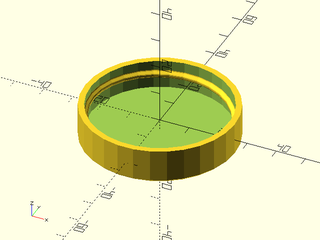
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
sp_cap(48,400,2);
Example 2:
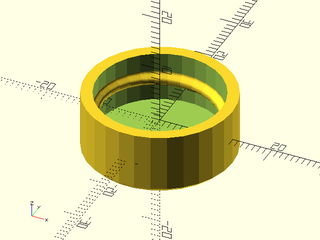
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
sp_cap(22,400,2);
Example 3:
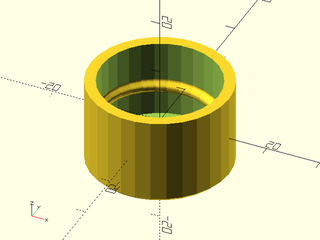
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
sp_cap(22,410,2);
Example 4:
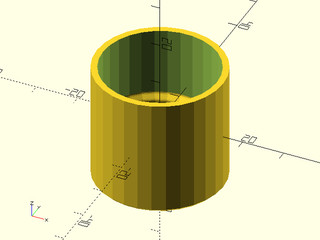
include <BOSL2/std.scad>
include <BOSL2/bottlecaps.scad>
sp_cap(28,415,1.5,style="M");
Synopsis: Returns the base diameter of an SPI bottle neck from the nominal diameter and type number.
Usage:
- true_diam = sp_diameter(diam,type)
Description:
Returns the actual base diameter (root of the threads) for a SPI plastic bottle neck given the nominal diameter and type number (400, 410, 415).
Arguments:
By Position | What it does |
---|---|
diam |
nominal diameter |
type |
closure type number (400, 410 or 415) |
Table of Contents
Function Index
Topics Index
Cheat Sheet
Tutorials
Basic Modeling:
- constants.scad STD
- transforms.scad STD
- attachments.scad STD
- shapes2d.scad STD
- shapes3d.scad STD
- drawing.scad STD
- masks2d.scad STD
- masks3d.scad STD
- distributors.scad STD
- color.scad STD
- partitions.scad STD
- mutators.scad STD
Advanced Modeling:
Math:
- math.scad STD
- linalg.scad STD
- vectors.scad STD
- coords.scad STD
- geometry.scad STD
- trigonometry.scad STD
Data Management:
- version.scad STD
- comparisons.scad STD
- lists.scad STD
- utility.scad STD
- strings.scad STD
- structs.scad
- fnliterals.scad
Threaded Parts:
Parts:
- ball_bearings.scad
- cubetruss.scad
- gears.scad
- hinges.scad
- joiners.scad
- linear_bearings.scad
- modular_hose.scad
- nema_steppers.scad
- polyhedra.scad
- sliders.scad
- tripod_mounts.scad
- walls.scad
- wiring.scad
STD = Included in std.scad