Binary/Hexadecimal |
 |
Binary is a number system that can represent any number with two digits (0 and 1). Each position in the binary number is two times more than the previous position. Hexadecimal is a number system that can represent any number with 0-9 digits and the letters A-F. After 9 is used the digit in the next position would be A. |
Bit |
 |
A bit is a unit of information for the computer which is usually portrayed as 0 or 1. |
Nibble |
refer to example image for Bit |
1 Nibble is equal to 4 bits. |
Byte |
refer to example image for Bit |
1 Byte is equal to 8 bits. A Byte can represent various things such as a string or a number. |
Data |
x=10 |
Data is information which is stored in a computer. Data is communicated or interpreted by a computer. |
Data Abstraction |
background: var(--clr-switch); |
Simplified version of data and only providing the necessary details. The logical properties of the data is separated from the representation of the data. |
Lossless/Lossy Compression |
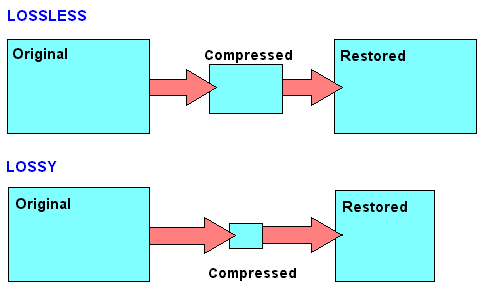 |
Lossy compression is making the files more compressed by discarding data that is considered not noticeable. Lossless compression is valuing the quality of the data rather than the size, and the data is kept identical when compressed. |
Metadata |
<img src="/static/assets/alicewithmask.jpg" style="height: 300px; width: 300px;"/> |
Data that gives information about more data. |
Computer Network |
(183).jpg) |
Connected devices that exchange data with each other. |
Parallel/Distributed computing |
 |
Parallel computing is where several processers preform tasks at the same time. Distributed computing is where the tasks are distributed between the processers to reach the same goal. |
Protocol |
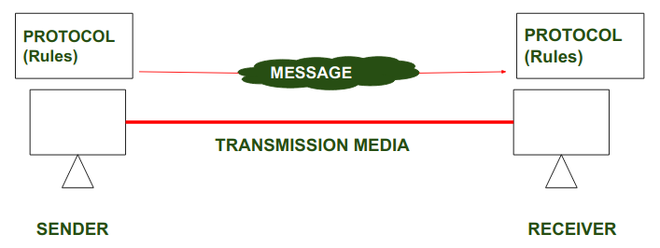 |
Protocol is a set of rules for communicating data between devices. |
TCP/IP |
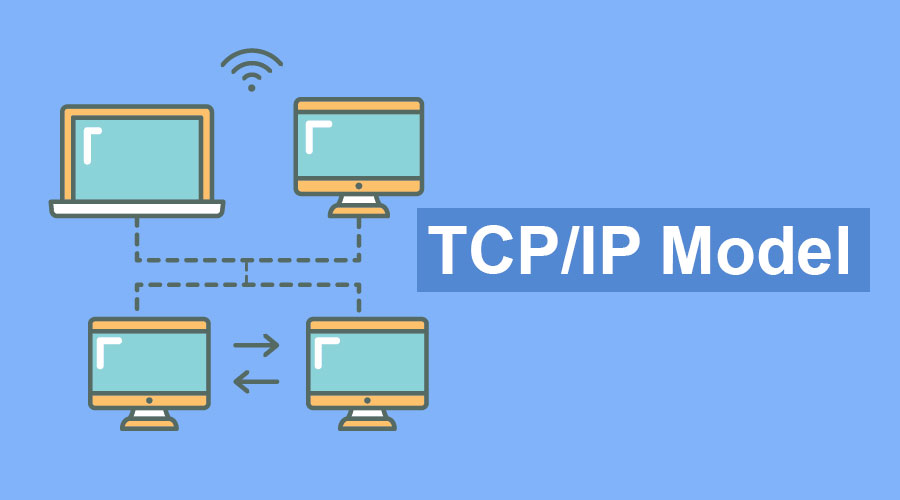 |
This stands for Transmission Control Protocol/Internet Protocol. This allows computers to communicate over long distance networks. The TCP part is for checking that the data has been transmitted and the IP part is the moving of the data. |
HTTP |
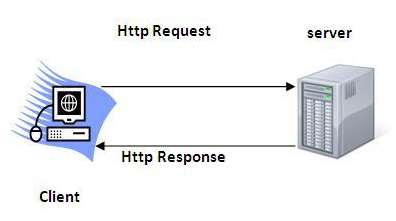 |
This stands for Hypertext Transfer Protocol. It is used to transfer data over the internet. It defines the commands used for transmitting data for webpages. |
GET |
"method": "GET", |
Recieves and requests data from a server. |
POST |
@app.route('/search/term/', methods=["POST"]) |
Sends data over to the server. |
Library |
import requests |
A library is parts of a program that are stored to do a common or specified thing that assist the programmer when they are building code. |
Dependencies |
Dependencies |
A dependency is an essential piece of code, functionality or library that's necessary for a different part of the code to work. |
Import |
Import |
Import is a command which is used for importing parts of files, settings and plug-ins. |
Web API |
Web API |
API stands for Application Program Interface. It is commands, functions and protocols used to create code which will interact with other systems. |
REST |
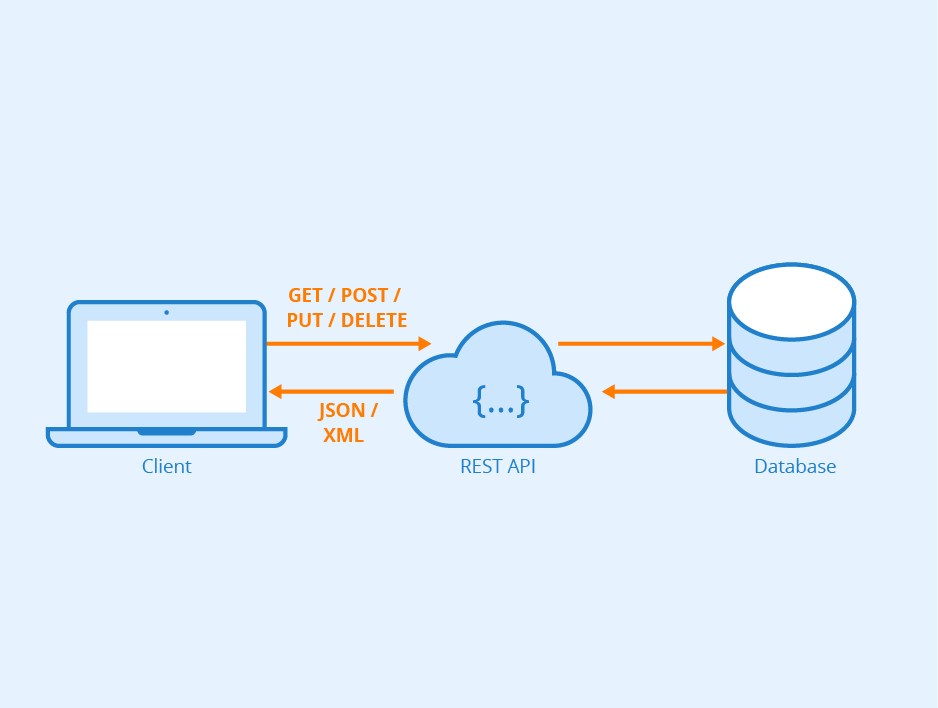 |
It is an API which uses HTTP requests to use and access data. A RESTful API interacts with other RESTful services. |
FETCH |
FETCH |
It is an API which makes HTTP requests to servers. |
Async |
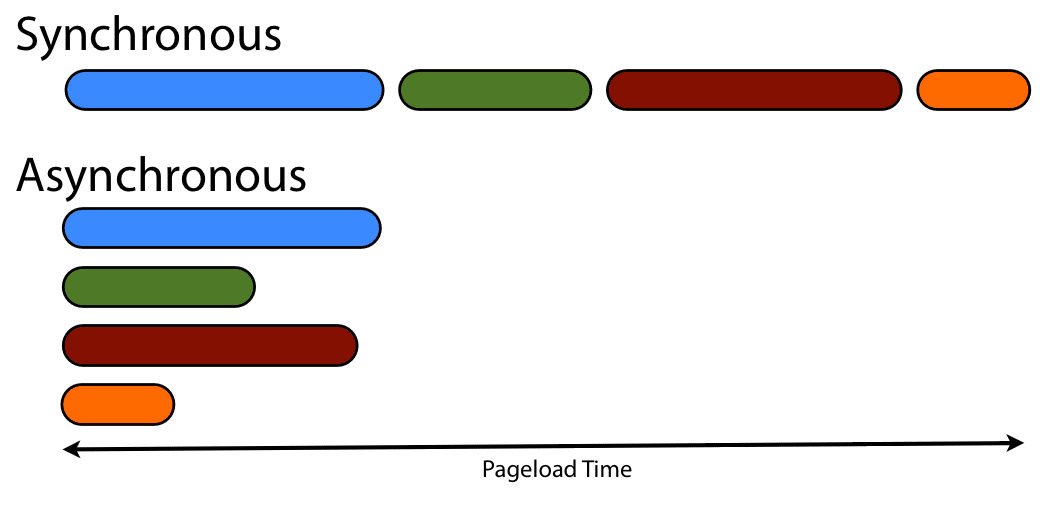 |
Asynchronous (async) programming allows a block of code to be executed without stopping the entire thread where the it is being run because async means that multiple events can be executed at the same time, and it is not necessary for one event to finish in order to move on to the next one. |
Request |
response = requests.request("GET", url, headers=headers) |
The program requests for data |
Response |
response = requests.request("GET", url, headers=headers) |
The program responds to the request for data |
Blueprints |
Blueprints |
Blueprints record operations to execute. It defines the views for routes to access program files. Blueprints also include the templates and static files that will be served on these routes. |
MVC |
 |
MVC stands for Model - View - Controller. It is a pattern that is used to develop new interfaces. Model = data. View = user interface. Controller = process handling input. It provides the fundamental pieces needed for designing a program. |
Code Sequence |
Code Sequence |
Specific steps carried out in order. |
Procedures/Functions |
Procedures/Functions |
Functions are the relationship between a defined value and a variable. Functions have both inputs and outputs as well as parameters which are like guidelines for the function. A procedure is a set of instructions which tells the computer how to run the program. |
Procedural Abstraction |
Procedural Abstraction |
When the logical properties of an procedure/function/action is separated from the details of how the procedure is implemented. For example, naming a function and calling it elsewhere in the code is procedural abstraction. It can be called multiple times and the program becomes a more manageable set of functions, instead of a flat sequence of thousands of statements. |
OOP |
  |
OOP programming stands for object-oriented programming. It is programming that organizes software design around objects rather than logic and functions. |
Class |
<div class="maincontainer"> |
In OOP, a class is a blue print for creating an object, it is needed before an object can be created. It defines the methods and variables in a particular object. Example: If you want to use a person in your program, a class called "person" would describe the person and what it would do. |
Attribute |
Attribute |
In OOP, an attribute is data representing the different properties/characteristics of an object and is stored inside the class or object. Example: The name of the person object or its age would be considered the attributes. |
Method |
Method |
In OOP, a method is a function that is part of a class and included in any object of that class. Example: The person should also be able to perform something such as walking or jumping, this would be considered the method of the person. |
Object |
Object |
In OOP the basic units are objects. An object is a self-contained component consisting of methods and attributes. Example: To actually use a person in your program and implement your class "person", you need to create an object of the type "person" |
CRUD |
CRUD |
CRUD is an acronym that stands for create, read, update, and delete. These are the four most basic operations necessary to implement a database system. They are also necessary for interacting with databases. |
Sort |
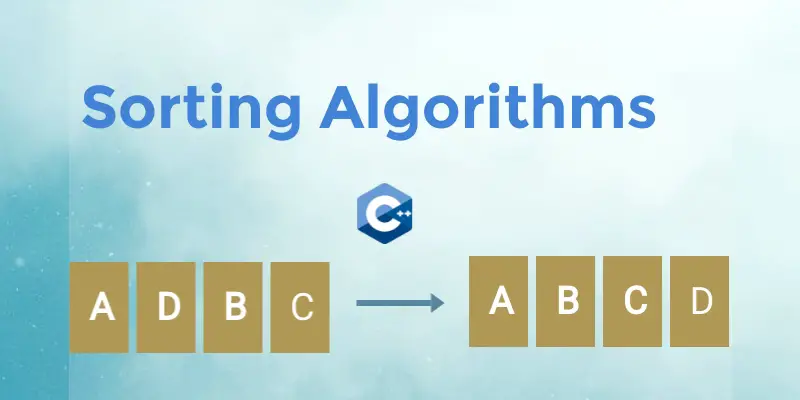 |
The process of organizing data in a particular order that makes it easier to find information. |
Search-Linear/Binary |
 |
Linear search finds an element in a list by searching for the element consecutively through the list until it is found. A binary search finds the middle element in the list and then another middle element in the half of the list coming before or after the initial middle element. The search does this until a middle element is matched with the searched element. |