React Native ~ Code Push - rohit120582sharma/Documentation GitHub Wiki
A React Native app is composed of JavaScript files and any accompanying images, which are bundled together by the packager and distributed as part of a platform-specific binary (i.e. an .ipa or .apk file).
Once the app is released, updating either the JavaScript code (e.g. making bug fixes, adding new features) or image assets, requires you to recompile and redistribute the entire binary, which of course, includes any review time associated with the store(s) you are publishing to.
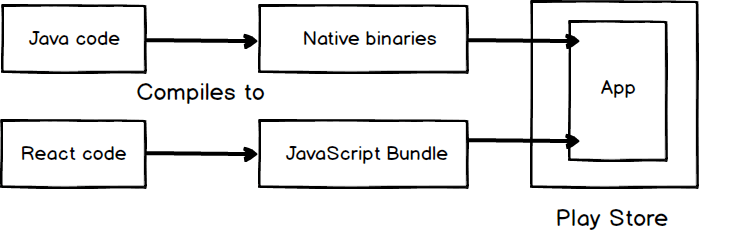
CodePush is a service from Microsoft that helps improve the deployment experience of your React Native apps.
Instead of embedding JavaScript bundle into the app, CodePush workflow stores the bundle in Microsoft servers or Appcenter. The user downloads the app in the device. When the app starts, the app downloads the JavaScript bundle from Appcenter. When we want to update the app, we push the update to Appcenter. And the app automatically downloads the update when it restarts the next time. In this way, developers bypass the App Store rollout for minor updates.

Note: Any product changes which touch native code (e.g. modifying your AppDelegate.m / MainActivity.java file, adding a new plugin) cannot be distributed via CodePush, and therefore, must be updated via the appropriate store(s).
- https://medium.com/@rajanmaharjan/get-started-with-wonderful-technology-d838aafdc2d3
- https://www.npmjs.com/package/code-push-cli
- https://www.npmjs.com/package/react-native-code-push
- https://www.youtube.com/watch?v=f6I9y7V-Ibk
npm install -g code-push-cli
Before you can release any updates, you first need to create a CodePush account. to do this, simply type the following command via the CLI and authenticate with either your GitHub or Microsoft account:
code-push register
Note: After registering, you are automatically logged-in with the CLI, so until you explicitly log out, you do not need to login again from the same machine.
Most commands within the CodePush CLI require authentication, and therefore, before you can begin managing your account, you need to login
code-push login
If at any time you want to determine if you are already logged in, you can run the following command to display the e-mail address associated with your current authentication session
code-push whoami
In order to end your session and delete this access key, simply run the following command:
code-push logout
Before you can deploy any updates, you need to register an app with the CodePush service using the following command:
code-push app add <appName> <os> <platform>
If your app targets both iOS and Android, please create separate apps for each platform with CodePush. This way, you can manage and release updates to them separately, which in the long run, also tends to make things simpler.
code-push app add CodepushApp-iOS ios react-native
code-push app add CodepushApp-Android android react-native
If you want to list all apps that you have registered with the CodePush server, you can run the following command:
code-push app ls
Whenever an app is registered using the CLI, the CodePush service creates two deployment keys by default: Staging and Production which is required for deployment.
If having a staging and production version of your app is enough to meet your needs, then you do not need to do anything else. However, if you want an alpha, dev, etc. deployment, you can easily create them using the following command:
code-push deployment add <appName> <deploymentName>
If at any time you'd like to view the list of deployments that a specific app includes, you can simply run the following command:
code-push deployment ls <appName> [--displayKeys|-k]
If you will be working with other developers on the same CodePush app, you can add them as collaborators using the following command:
code-push collaborator add <appName> <collaboratorEmail>
This expects the developer to have already registered with CodePush using the specified e-mail address
Over time, if someone is no longer working on an app with you, you can remove them as a collaborator using the following command:
code-push collaborator rm <appName> <collaboratorEmail>
If at any time you want to list all collaborators that have been added to an app, you can simply run the following command:
code-push collaborator ls <appName>
npm install --save react-native-code-push
Note: Do use a specific version of the CodePush plugin in order to support the exact version of React Native you are using.
React Native version(s) Supporting CodePush version(s)
v0.46-v0.53 v5.1+ (RN removed unused registration of JS modules)
v0.54-v0.55 v5.3+ (Android Gradle Plugin 3.x integration)
v0.56-v0.57 v5.4+ (RN upgraded versions for Android tools)
react-native link react-native-code-push
Note: If you use
react-native link
to automatically link the plugin, these steps have already been done for you so you may skip manual section for both iOS and Android.
With the CodePush plugin downloaded and linked, and your app asking CodePush where to get the right JS bundle from, the only thing left is to add the necessary code to your app to control the following policies:
- When to check for an update? (e.g. app start, in response to clicking a button in a settings page, periodically at some fixed interval)
- When an update is available, how to present it to the end user?
import codePush from "react-native-code-push";
let codePushOptions = { checkFrequency: codePush.CheckFrequency.IMMEDIATE };
...
class App extends Component {
componentDidMount(){
codePush.sync({
updateDialog: true,
installMode: codePush.InstallMode.IMMEDIATE
});
}
...
}
App = codePush(codePushOptions)(App);
Once your app has been configured and distributed to your users, and you've made some JS and/or asset changes, it's time to instantly release them! The simplest (and recommended) way to do this is to use the release-react command in the CodePush CLI, which will handle bundling your JavaScript and asset files and releasing the update to the CodePush server.
code-push release-react <appName> <platform>
[--bundleName <bundleName>]
[--deploymentName <deploymentName>]
[--description <description>]
[--development <development>]
[--disabled <disabled>]
[--entryFile <entryFile>]
[--gradleFile <gradleFile>]
[--mandatory]
[--noDuplicateReleaseError]
[--outputDir <outputDir>]
[--plistFile <plistFile>]
[--plistFilePrefix <plistFilePrefix>]
[--sourcemapOutput <sourcemapOutput>]
[--targetBinaryVersion <targetBinaryVersion>]
[--rollout <rolloutPercentage>]
[--privateKeyPath <pathToPrivateKey>]
[--config <config>]
code-push release-react MyApp-iOS ios -d "Production" --description "Modified the header color"
code-push release-react MyApp-Android android -d "Production" --description "Modified the header color"
Promote the app from Staging to Production:
code-push promote MyApp-Android Staging Production