Home - poliklinikvildan/expressBookReviews GitHub Wiki
Welcome to the expressBookReviews wiki!
is a web application built using Node.js and Express.js. It allows users to browse, search, and manage a collection of books. The main file, index.js, serves as the backbone of the application, handling incoming requests, routing them to the appropriate endpoints, and executing the corresponding actions.
The index.js file enables users to retrieve the complete book list, search for books by titles or authors, and access detailed information about individual books. Users can register and log in to the system, which facilitates personalized experiences and book recommendations.
Users can also add and modify book reviews, contributing to a community of book enthusiasts. The system allows users to delete their own reviews, giving them control over their contributions.
To enhance performance, the index.js file uses asynchronous operations and promises for efficient data retrieval and external API calls. This ensures smooth and responsive interactions, even with large book databases and complex search operations.
The project includes the booksdb.js file, which serves as the data source for book information, and the userdb.json file for managing user-related information. These files can be expanded and updated to accommodate more books and additional user attributes or preferences.
Overall, the Coursera Books Project showcases the capabilities of Node.js, Express.js, and JSON data management in creating a feature-rich web application for book enthusiasts. It provides an intuitive and efficient way for users to explore, review, and engage with a vast library of books.
Let's explore and explain the different sections and functionalities of this file:
Importing Dependencies:
javascript code
const express = require('express');
const bodyParser = require('body-parser');
The project relies on the Express.js framework for building the server, and the body-parser middleware for parsing JSON data. These dependencies are imported at the beginning of the file.
Creating the Express Application:
javascript code
const app = express();
app.use(bodyParser.json());
An instance of the Express application is created, and the bodyParser.json() middleware is used to parse incoming JSON data.
Importing the Book Database:
javascript code
const books = require('./booksdb');
The booksdb.js file, which contains an array of book objects, is imported. This file serves as the database for book-related information in the project.
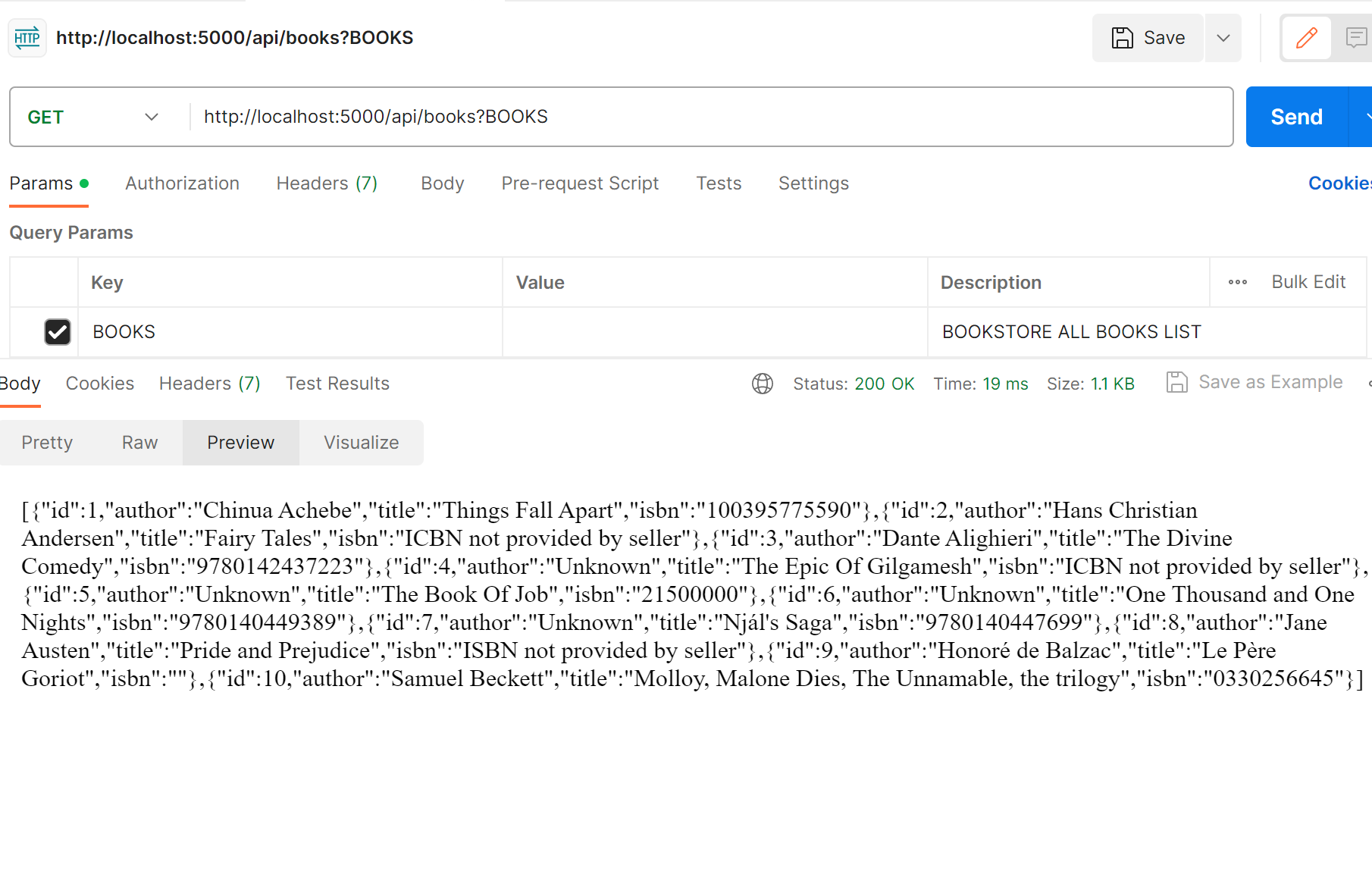
Javascript code for Task1:
// Task1: Get the book list available in the shop
app.get('/api/books', (req, res) => {
// Exclude the review property from the response
const booksWithoutReview = books.map(({ review, ...rest }) => rest);
// Return the list of books without the review property
res.json(booksWithoutReview);
});
When a GET request is made to /api/books, the server executes the callback function (req, res) => {...}. In this function, the books array is mapped using the map() met-hod. The callback function provided to map() uses object destructuring to exclude the review property from each book object. The resulting array, booksWithoutReview, contains the book objects without the review property. Finally, the server responds with a JSON object containing the booksWithoutReview array using the res.json() method. By accessing http.//localhost:5000/api/books, you will receive a JSON response with the list of books, including the book descriptions provided by the seller, but exclu-ding client reviews.
javascript code
app.get('/api/books/:isbn', (req, res) => {
// Code to retrieve a specific book based on ISBN
});
This endpoint handles the request to get a book based on its ISBN. It retrieves the book from the database by matching the ISBN parameter and returns the book if found, or an error message if not found.
javascript code
app.get('/api/books/author/:author', (req, res) => {
// Code to retrieve all books written by a specific author
});
This endpoint handles the request to get all books written by a specific author. It retrieves the books from the database by matching the author parameter (case-insensitive) and returns the list of books or an error message if no books are found.
javascript code
app.get('/api/books/title/:title', (req, res) => {
// Code to retrieve all books with a specific title
});
This endpoint handles the request to get all books with a specific title. It retrieves the books from the database by matching the title parameter (case-insensitive) and returns the list of books or an error message if no books are found.
javascript code
app.get('/api/books/:isbn/review', (req, res) => {
// Code to retrieve the review of a specific book based on ISBN
});
This endpoint handles the request to get the review of a specific book based on its ISBN. It retrieves the book from the database by matching the ISBN parameter and returns the review if found, or an error message if not found.
javascript code
app.post("/api/users/register", (req, res) => {
// Code to register a new user
});
This endpoint handles the request to register a new user. It expects the email and password in the request body and saves the user information. In the example provided, it logs the user details to the console and sends a success message as the response.
javascript code
app.get("/api/users/login", (req, res) => {
// Code to perform user login
});
This endpoint handles the request for user login. It expects the email and password in the request body, checks the provided credentials against the registered users' information, and sends a success message or an error message as the response.
javascript code
app.post('/api/books/:isbn/review', (req, res) => {
// Code to add or modify a book review based on ISBN
});
This endpoint handles the request to add or modify a book review based on the book's ISBN. It expects the review data in the request body, finds the book by ISBN, and adds or modifies the review property of the book accordingly. In the provided example, it logs the review to the console and sends a success message as the response.
javascript code
app.delete('/api/books/:isbn/review', (req, res) => {
// Code to delete a book review based on ISBN
});
This endpoint handles the request to delete a book review based on the book's ISBN. It finds the book by ISBN and removes the review property. In the provided example, it logs the review deletion to the console and sends a success message as the response.
The task programs how an endpoint is defined to retrieve all books from the server with a 1-second delay introduced using setTimeout to simulate an asynchronous operation. After the delay, the complete list of books is sent as a JSON response using the res.json method. The purpose of the task is to demonstrate handling asynchronous operations in Express using callback functions. The provided code snippet accurately represents the implementation of the task. The endpoint "/api/books" is defined with the HTTP GET method using app.get. Inside the route handler function, setTimeout is used to introduce a delay of 1 second (1000 milliseconds) before sending the response. After the delay, the res.json method is called to send the complete books array as a JSON response. The delay introduced by setTimeout simulates an asynchronous operation, as if there was a time-consuming task involved in retrieving the books. The purpose of this task is to demonstrate how to handle asynchronous operations in Express using callback functions.
javascript code
// Task10: Get all books - Using async callback function app.get('/api/books', (req, res) => {
// Retrieve all books using async callback function setTimeout(() => { res.json(books); }, 1000); });
The GET request to retrieve all books with a delay of 1 second will be initiated by the tool. Postman or any other tool that allows HTTP requests can be opened.
A new request can be created by selecting the appropriate options in the tool's interface. The HTTP method of the request should be set to GET. The request URL should be entered as 'http.//localhost:5000/api/books', assuming the server is running locally on port 5000. If the server is running on a different port or host, the URL should be adjusted accordingly. The request can be sent by clicking the "Send" button or using the corresponding command in the tool. The GET request to the server will be initiated by the tool.
The server, upon receiving the request, will start executing the corresponding route handler function for the /api/books endpoint.
Starting the Server:
javascript code
app.listen(5000, () => {
console.log('Server started on port 5000');
});
Finally, the server is started on port 5000, and a message is logged to the console indicating that the server is running.
These are the main sections and functionalities of the index.js file in the Coursera Books Project. It serves as the central controller for handling HTTP requests, interacting with the book and user databases, and providing appropriate responses to the client.