Building the UserForm - ldco2016/microurb_web_framework GitHub Wiki
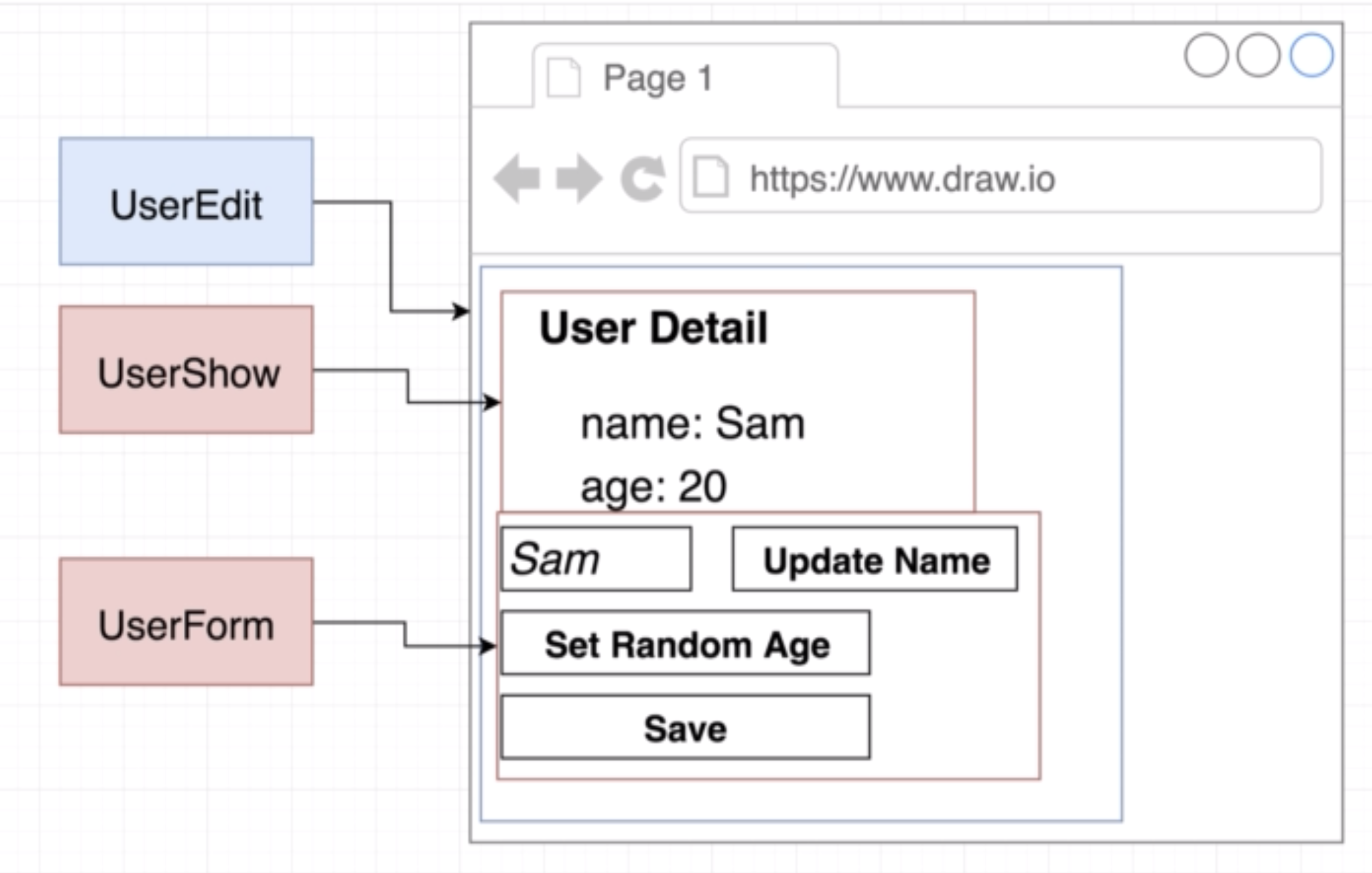
Below is my idea behind the UserForm class, its properties and how that can lead to reusable logic inside of it:
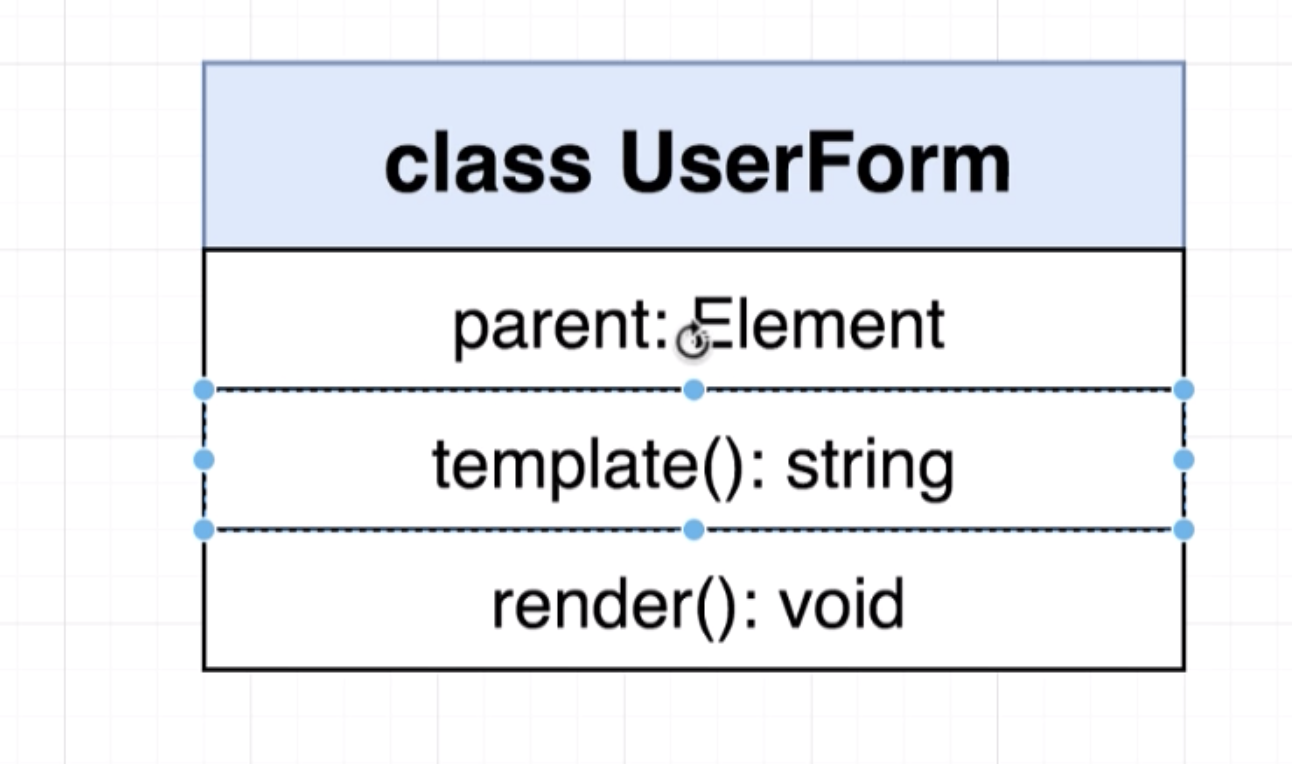
Inside of class UserForm, I am going to start off with one property called parent
and two methods. The first method is going to be called template()
and it will return a string. The idea behind the method is that it will return a string that will contain some amount of HTML to return to a user. Additionally, the render()
method will cause the HTML to be displayed to the DOM.
To ensure that this can be inserted into the DOM, thats where the parent
property comes in. parent
will be a reference to some HTML element that exists inside the DOM.
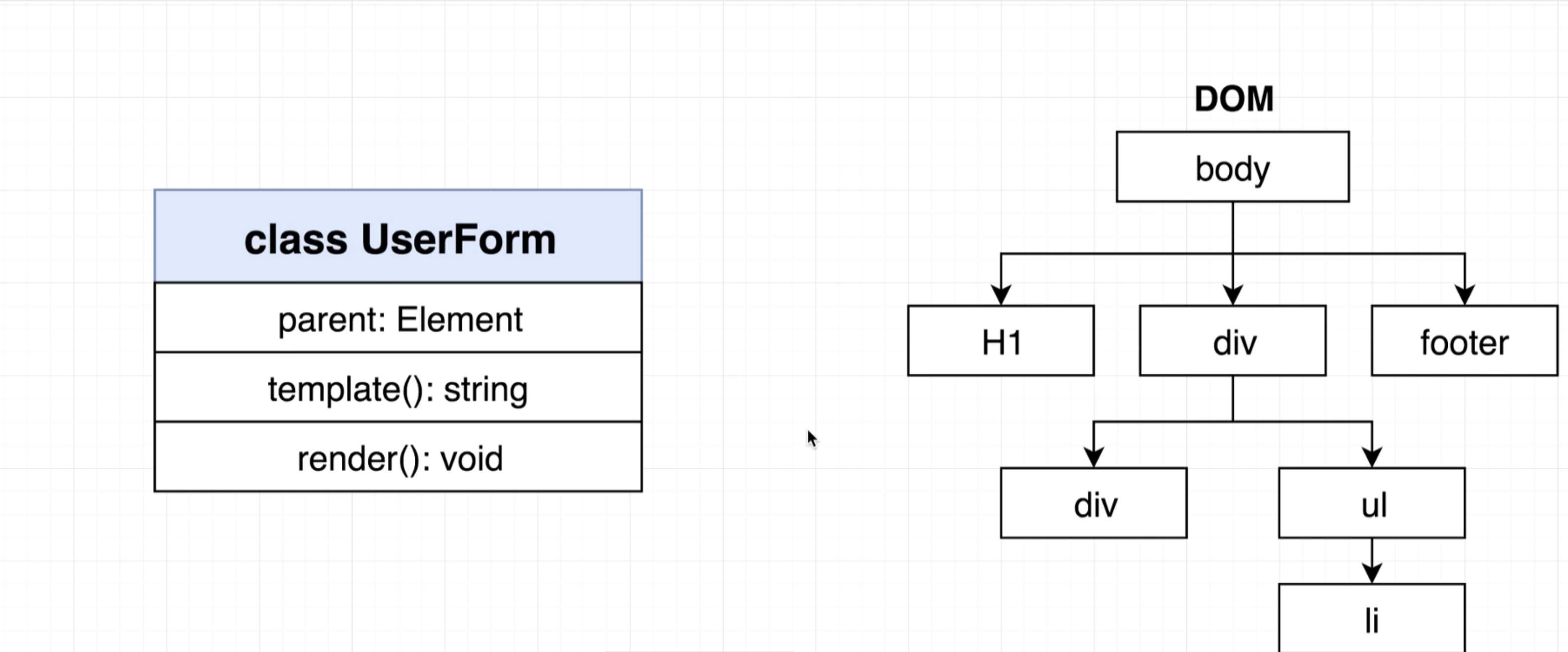
I am going to call render()
, internally, render()
is going to call template()
and template()
is going to contain a string that contains some amount of HTML.
So you can imagine that template()
is going to render a form, a label and an input like so:
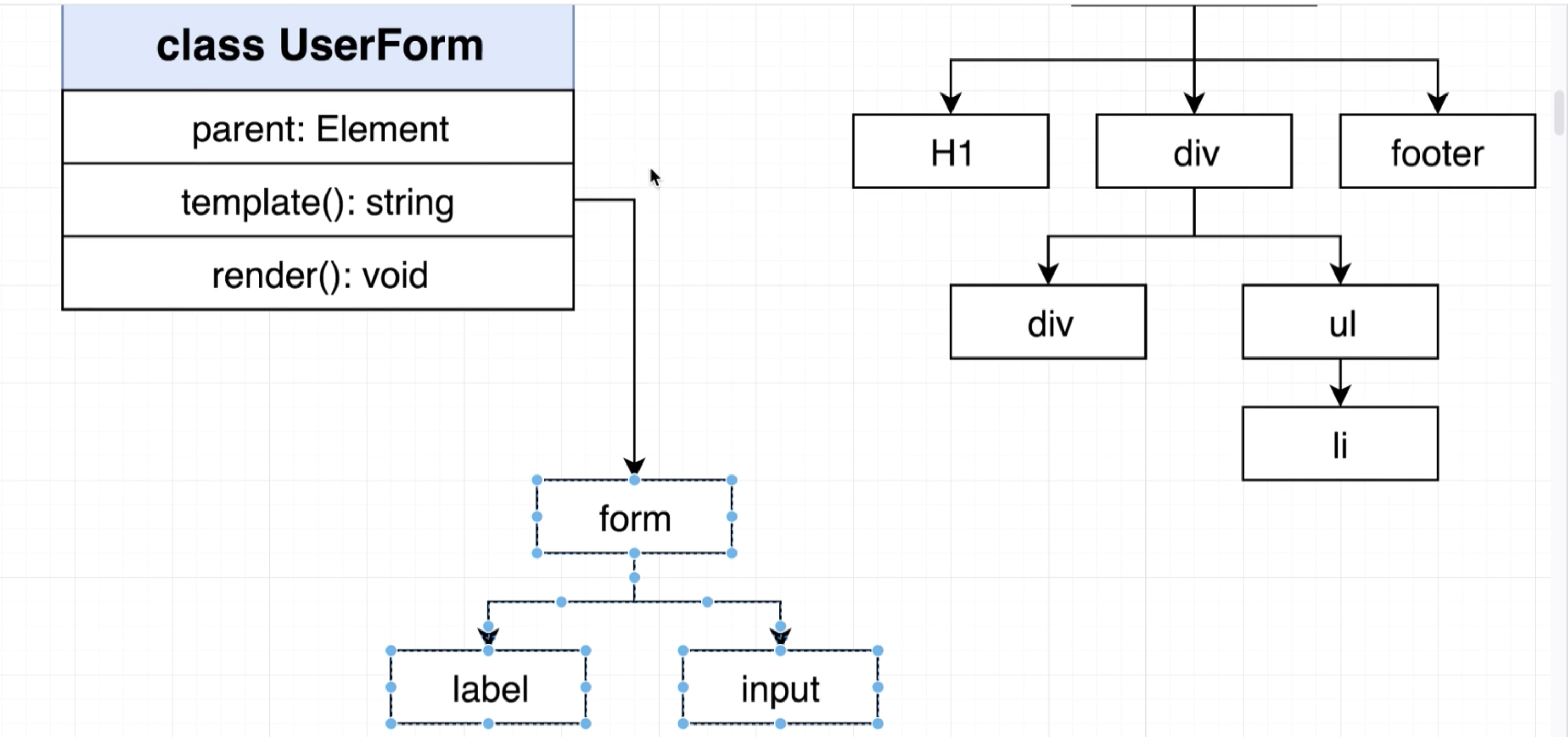
The render()
method will then take this HTML, append it as a child to the parent
property. This parent
property is going to be a reference to some HTML element that already exists inside the DOM. So if we study the DOM inside the diagram, maybe the parent
element is going to point at the div. So when render()
calls template()
and gets the HTML snippet, render()
will take that HTML and append it to the parent: Element
.
I am not going to do fancy React style DOM diffing, I am going to be inserting and removing snippets of HTML. So this is pretty primitive, but it is easy for me to implement, certainly easier than taking a React style approach.
So I created a src/views/UserForm.ts
file and inside there I added:
export class UserForm {}
This will have a parent property that is a reference to an HTML element like so:
export class UserForm {
parent: Element;
}
Element is the most general base class, basically a reference to any HTML element. Next up, I will add my template()
method like so:
export class UserForm {
parent: Element;
template(): string {
return `<div>
<h1>User Form</h1>
<input />
</div>`;
}
}
I need to also ensure this thing has a render()
method and I can use that to actually call the template()
and append it to the parent
.