Redux Saga Middleware - htalexnguyen/htapp GitHub Wiki
-
Saga is like a separate thread in your application that's solely responsible for side effects (i.e. asynchronous things like data fetching and impure things like accessing the browser cache or device storage).
-
It uses generator function to handle side effects instead of async/await
function* example() {
const response = yield fetch(url);
console.log(response);
}
-
Redux-saga is a redux middleware, which means this thread can be started, paused and cancelled from the main application with normal redux actions, it has access to the full redux application state and it can dispatch redux actions as well.
-
Its core function is to intercept the action sent to the reducer, perform any asynchronous operation that may be present in the action, and present an object to the reducer.
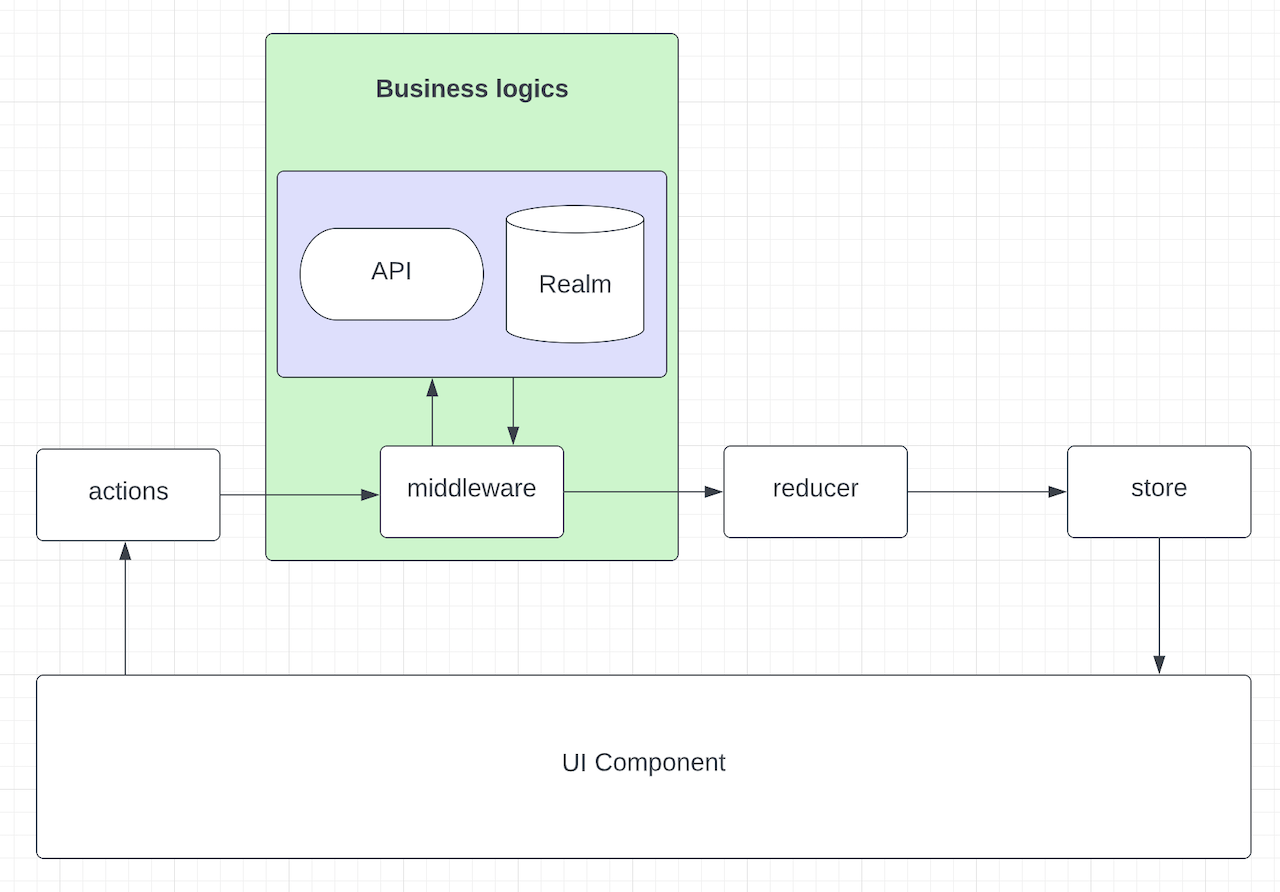
- It looks similar to async/await but there are somethings it can do but async/await can't.
-
split logics and UI.
-
reduce number of re-render times
-
give ability to cancel promise (async/await can't do)
-
many other built-in effects.
-
avoid the use of
async componentDidMount()
Component can be unmounted before the async task get completed and we will get thisWarning: Can't call setState (or forceUpdate) on an unmounted component. This is a no-op, but it indicates a memory leak in your application. To fix, cancel all subscriptions and asynchronous tasks in the componentWillUnmount method.
=> It could make our components more readable by taking the fetching data, business logics and only return the necessary data to render.
=> We also improve the app performance by reducing the number of re-render times.