fork - hochan222/Everything-in-JavaScript GitHub Wiki
๋ฆฌ๋ ์ค ์์คํ ์์๋ ํ๋ก์ธ์ค๋ฅผ ์์ฑํ๋ system call์ธ fork, vfork ํจ์๋ฅผ ์ ๊ณตํ๊ณ ์์ต๋๋ค. fork์ vfork๋ ํ๋ก์ธ์ค ์์ ์ ํ๋ก๊ทธ๋จ ์ด๋ฏธ์ง๋ฅผ ๋น๋กฏํ์ฌ ๋ง์ ์ ๋ณด๋ฅผ ๋ณต์ ํ ์์ ํ๋ก์ธ์ค๋ฅผ ์์ฑํฉ๋๋ค.
//fork() ํจ์.
//Parent Process, Child Process์ ๊ดํ ์์ .
#include <stdio.h>
#include <unistd.h> // pid_t ํ์
, fork() ํจ์๊ฐ ํฌํจ๋ ํค๋
int main()
{
pid_t pid; // pid_t ํ์
์ ํ๋ก์ธ์ค์์ด๋(PID)๋ฅผ ์ ์ฅํ๋ ํ์
.
/* fork another process */ pid = fork(); // fork() ํจ์๋ ํ์ฌ ์คํ์ค์ธ ํ๋ก์ธ์ค๋ฅผ ๋ณต์ฌํ์ฌ ์คํํ๋ค.
if (pid < 0) { /* error occurred */
// pid๊ฐ ์์์ผ ๊ฒฝ์ฐ๋ fork() ์คํจ.
fprintf(stderr, "Fork Failed");
exit(-1);
} else if (pid == 0) {
/* child process */
// Child Process๋ pid๋ก 0์ ๋ฆฌํด.
printf("Child Process is running\n");
sleep (10);
execlp("/bin/ls", "ls", NULL); // ์๋์์ ์ค๋ช
.
} else {
/* parent process */
/* parent will wait for the child to complete */
wait (NULL);
/* wait()์ ํ๋กํ ํ์
์ pid_t wait(int* status)์ด๋ค.
status๋ Child Process ์ข
๋ฃ ์์ ์ํ์ ๋ณด๋ฅผ ์ ์ฅํ๊ณ ,
๋ฆฌํด๊ฐ์ธ pid_t๋ ์ข
๋ฃ๋ Child Process์ PID๋ฅผ ๋ฆฌํดํ๋ค.
Child Process๊ฐ ์ข
๋ฃ๋ ํ์ Parent Process๊ฐ ์คํ๋๋ค. */
printf ("Child Complete\n");
exit(0); // ์คํ๋ Parent Process์ ๋๋จธ์ง ๋ถ๋ถ.
}
}
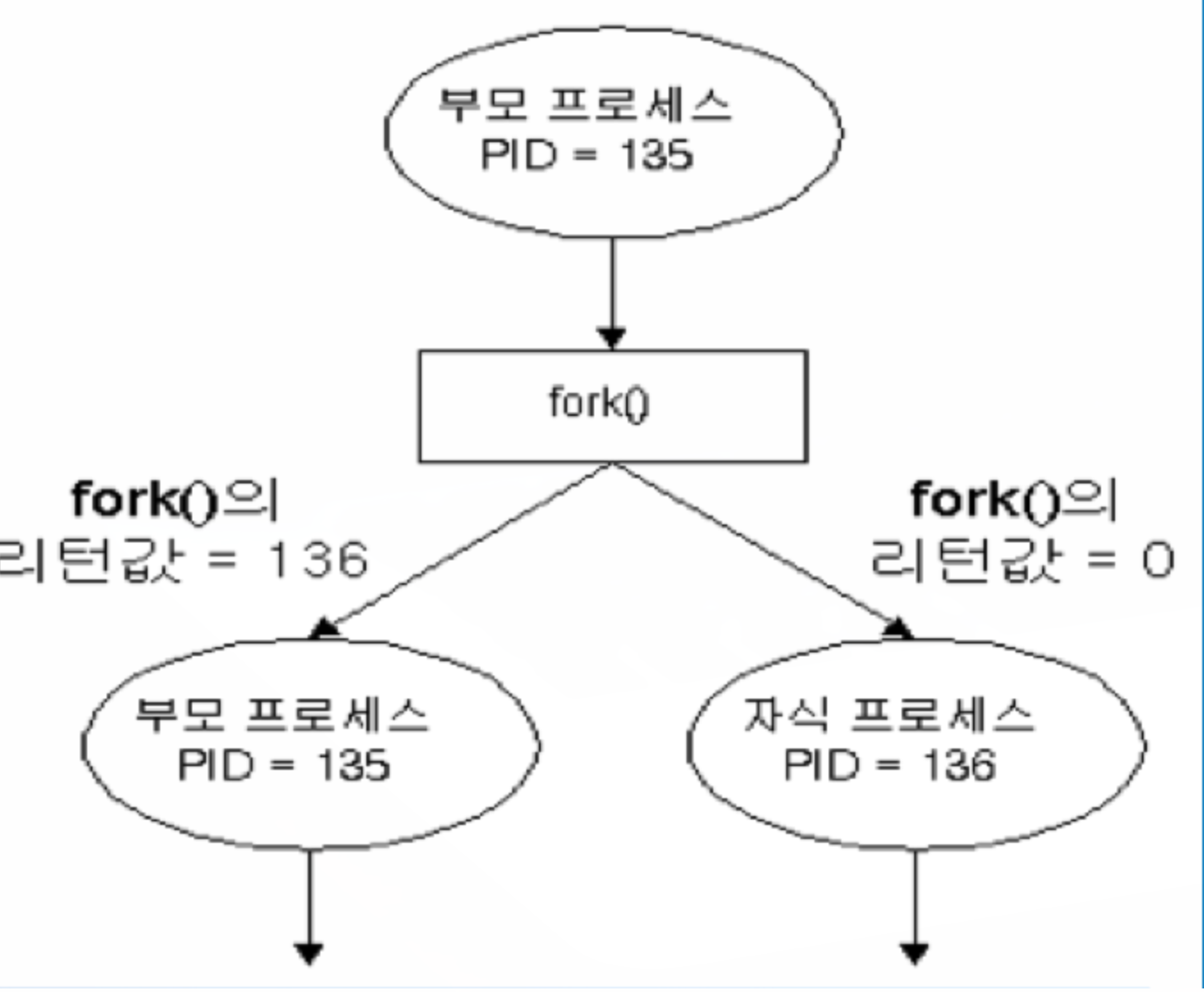
์์ ํ๋ก์ธ์ค์๊ฒ pid๋ฅผ 0์ ์ฃผ๋ ์ด์ ๋ ์์ ํ๋ก์ธ์ค์์๋ ํ์ํ๋ฉด getppid ์์คํ ํธ์ถ๋ก ๋ถ๋ชจ ํ๋ก์ธ์ค์ pid๋ฅผ ์์๋ผ ์ ์๊ธฐ ๋๋ฌธ์ ๋๋ค. ํ์ง๋ง ๋ถ๋ชจ ํ๋ก์ธ์ค์์๋ ์์ ํ๋ก์ธ์ค์ pid๋ฅผ ์ ์ ์๋ ๋ฐฉ๋ฒ์ fork์ vfork์ ์ํด ๋ฐํํ๋ pid๋ฅผ ๊ธฐ์ตํ๋ ๋ฐฉ๋ฒ์ ์ฌ์ฉํด์ผ ํฉ๋๋ค.
WIFEXITED, WEXITSTATUS
WIFEXITED : ์์ ํ๋ก์ธ์ค๊ฐ ์ ์ ์ข
๋ฃํ ๊ฒฝ์ฐ TRUE๋ฅผ ๋ฐํํ๋ค.
WEXITSTATUS : ์์ ํ๋ก์ธ์ค์ ์ ๋ฌ ๊ฐ์ ๋ฐํํ๋ค.
capturing-exit-status-code-of-child-process
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
int main(void)
{
pid_t p = fork();
if ( p == -1 ) {
perror("fork failed");
return EXIT_FAILURE;
}
else if ( p == 0 ) {
execl("/bin/sh", "bin/sh", "-c", "./failprog", "NULL");
return EXIT_FAILURE;
}
int status;
if ( waitpid(p, &status, 0) == -1 ) {
perror("waitpid failed");
return EXIT_FAILURE;
}
if ( WIFEXITED(status) ) {
const int es = WEXITSTATUS(status);
printf("exit status was %d\n", es);
}
return EXIT_SUCCESS;
}