Chapter 3: Digging to the Roots of JS - hochan222/Everything-in-JavaScript GitHub Wiki
ES6๋ JS์ ๋ฐ์ดํฐ ๊ตฌ์กฐ/ ์ปฌ๋ ์ ํ์ ๋ค์ iterable๋ค๋ก ์ ์ํ๋ค.
Closure๋ ํจ์๊ฐ ๊ธฐ์ต์ ํด์ ๋ฒ์ ์ธ๋ถ์์ ๋ณ์์ ์ก์ธ์คํ๋ ๊ฒ์ ๋งํ๋ค.
function greeting(msg) {
return function who(name) {
console.log(`${ msg }, ${ name }!`);
};
}
var hello = greeting("Hello");
var howdy = greeting("Howdy");
hello("Kyle");
// Hello, Kyle!
hello("Sarah");
// Hello, Sarah!
howdy("Grant");
// Howdy, Grant!
์ผ๋ฐ์ ์ผ๋ก ํจ์๊ฐ ์ข
๋ฃ๋๋ฉด garbage collected ๋ ๊ฒ์ด๋ผ๊ณ ์๊ฐํ๋ค. (๋ฉ๋ชจ๋ฆฌ์์ ์ ๊ฑฐ ์๋ฏธ)
๊ทธ๋ฌ๋ ๋ด๋ถ ํจ์ ์ธ์คํด์ค๋ ์ฌ์ ํ ์ด์์๊ธฐ ๋๋ฌธ์ ํด๋ก์ ๋ msg ๋ณ์๋ฅผ ์ ์งํ๋ค.
ํด๋ก์ ๋ msg ๋ณ์ ๊ทธ ์์ฒด๋ค. ๋ฐ๋ก ๋ณธ๋จ๊ฑฐ๋ ๋ง๋ ๋ณ์๊ฐ ์๋๋ค. ์ด ๋ณ์๋ค์ ์ ์ง๋๊ณ ์
๋ฐ์ดํธ๋ ๋๋ค.
function counter(step = 1) {
var count = 0;
return function increaseCount(){
count = count + step;
return count;
};
}
var incBy1 = counter(1);
var incBy3 = counter(3);
incBy1(); //1
incBy1(); //2
incBy3(); //3
incBy3(); //6
incBy3(); //9
ํด๋ก์ ๋ ๋น๋๊ธฐ ์ฝ๋์์๋ callback์ผ๋ก ๋์ฒด๋ก ์ฌ์ฉ๋๋ค.
function getSomeData(url) {
ajax(url,function onResponse(resp){
console.log(
`Response (from ${ url }): ${ resp }`
);
});
}
getSomeData("https://some.url/wherever");
// Response (from https://some.url/wherever): ...
ํด๋ก์ ๋ ๊ผญ ์ธ๋ถ ๋ฒ์๊ฐ ํจ์์ผ ํ์๋ ์๋ค. ์ ์ด๋ ํ๋์ ๋ณ์๊ฐ ์ธ๋ถ ๋ฒ์์ ์์ด๋ ํด๋ก์ ๋ฅผ ๋ง๋ค ์ ์๋ค.
ํจ์๊ฐ ์ ์๋๋ฉด ํด๋ก์ ๋ฅผ ํตํด ๋๋ฌ์ธ๋ Scope๊ฐ ๋ง๋ค์ด์ง๋ค. Scope๋ ๋ณ์์ ๋ํ ์ฐธ์กฐ๋ฅผ ํ์ธํ๋ ๋ฐฉ๋ฒ์ ์ ์ดํ๋ โโ๊ท์น ์งํฉ์ด๋ค.
ํจ์๋ ๊ทธ๋ค์ Scope์ ์ํฅ์ ์ฃผ๋ ๋๋ค๋ฅธ ํน์ฑ์ ๊ฐ์ง๊ณ ์๋ค. ์ด ํน์ฑ์ ์คํ ์ปจํ
์คํธ(execution context)๋ก ๊ฐ์ฅ ์ ์ค๋ช
๋๋ฉฐ this ํค์๋๋ฅผ ํตํด ํจ์์ ๋
ธ์ถ๋๋ค.
์ค...! execution context ๋๋์ด.. ๋ค์ด๋ณธ ๋จ์ด๊ฐ ์ด๋ก ์ ๋ฑ์ฅํ๋ฐ...
Scope๋ ํจ์๋ฅผ ์ ์ํ๋ ์๊ฐ ์ ์ ์ด๊ณ ๊ณ ์ ๋ ๋ณ์๋ค์ ํฌํจํ๋๋ฐ ํจ์์ execution context๋ ๋์ ์ด๋ฉฐ ๊ทธ๊ฒ์ด ์ด๋ป๊ฒ ๋ถ๋ฆฌ๋๋์ ๋ฐ๋ผ์ ๋ฌ๋ผ์ง๋ค. (ํจ์๊ฐ ํธ์ถ ๋ ๋๋ง๋ค ๋ฌ๋ผ์ง๋ค.)
execution context๋ ํจ์๊ฐ ์คํ๋๋ ๋์ ์ฌ์ฉ ํ ์ ์๋ ๊ฐ์ฒด์ ์์ฑ์ด๋ค.
function classroom(teacher) {
return function study() {
console.log(
`${ teacher } says to study ${ this.topic }`
);
};
}
var assignment = classroom("Kyle");
๋๋๊ฒ๋ ์ ์ฝ๋๋ ์ค๋ฅ๊ฐ ์๋๋ค. this๊ฐ execution context์ ์์กดํ๊ธฐ ๋๋ฌธ์ด๋ค.
ํํธ assignment();
๋ undefined๊ฐ ๋์ค๋๋ฐ ์ด๋ ์ฐ์ , "strict" ๋ชจ๋๋ก ์ค์ ํ์ง ์์๊ณ , ๊ทธ๋ด ๊ฒฝ์ฐ ์ ์ญ ๊ฐ์ฒด์(window)context๋ก ๊ฐ๋ฅดํค๊ฒ ๋ผ์ ์ ์ญ ์ปจํ
์คํธ์๋ topic ๋ณ์๊ฐ ์๊ธฐ ๋๋ฌธ์ undefined๊ฐ ๋์จ๋ค.
var otherHomework = {
topic: "Math"
};
assignment.call(otherHomework);
์ฐ๋ฆฌ๋ call ๋ฉ์๋๋ก this์ ์ฐธ์กฐ๋ฅผ ํจ์ ํธ์ถ์ ๋ํด ์ค์ ํ ์ ์๋ค. (์ค์ค,, call์ด ์ด๋ฐ ์๋ฏธ์๋ค๋...!)
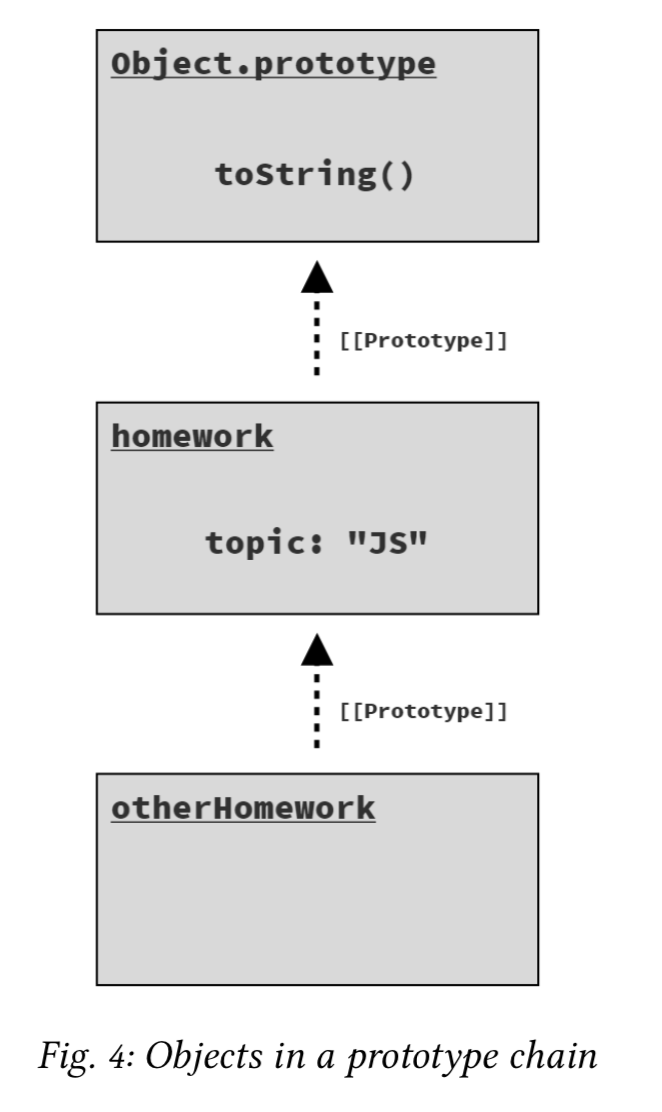
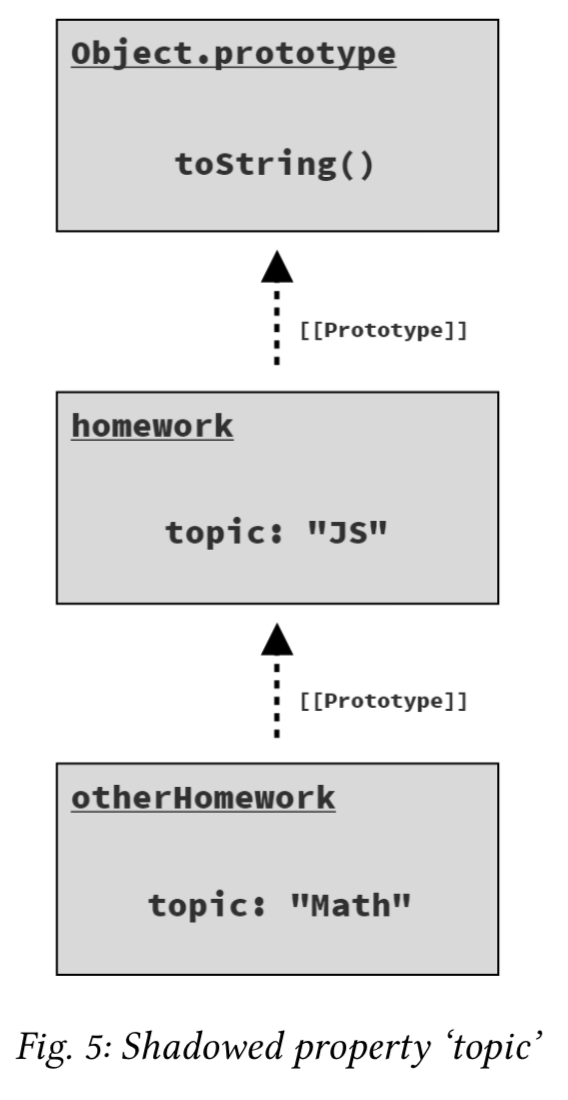
