API Documentation - WassimJabz/Purposeful GitHub Wiki
Our project has extensive java documentation that be found in the mmss/javadoc folder, that also describes the way each controller method is RESTfully exposed. The endpoint of every method is described here, with details available under each dropdown.
Endpoint Naming Convention: /api/classname. This lets you have one POST, PUT, GET, DELETE. If you need to make it unique, go for /api/classname/detail, where the detail is a noun, not a verb ( no actions :) ). Eg. we could have a post method at /api/appuser/moderator and api/appuser/regularuser for the appuser controller.
Notes For Controllers:
- Use @RequestBody for parameters that are Dtos
- Use @RequestParam for parameters that are passed through the url, with /api/classname?parameterName=value
POST: /api/login, /api/login/
Login an account to the Purposeful app
A custom header is required for authentication with the form: "Authorization": "Base ${base64(username:password)}"
A JWT token if the provided username and password matched, 401 Unauthorized otherwise.
GET: /api/login, /api/login/
Verify that a token is valid, i.e. an user is logged in.
None, only the custom header is required for authentication with the form: "Authorization": "Bearer token".
An appUserDTO:
{
"id": "String",
"email": "String",
"password": "",
"firstname": "String",
"lastname": "String"
}
or 401 Unauthorized otherwise.
GET: /login/employee (Example from 321)
Login an employee
String username
a request parameter, the username of the employee
String password
a request parameter, the password of the employee
An EmployeeDto
{
"phoneNumber" : "String",
"userName": "String",
"personId": "int",
"communicationId": "int"
}
GET: /api/appuser/users
Allows to get all users in the system
{
"id": "String",
"email": "String",
"password": "",
"firstname": "String",
"lastname": "String"
}
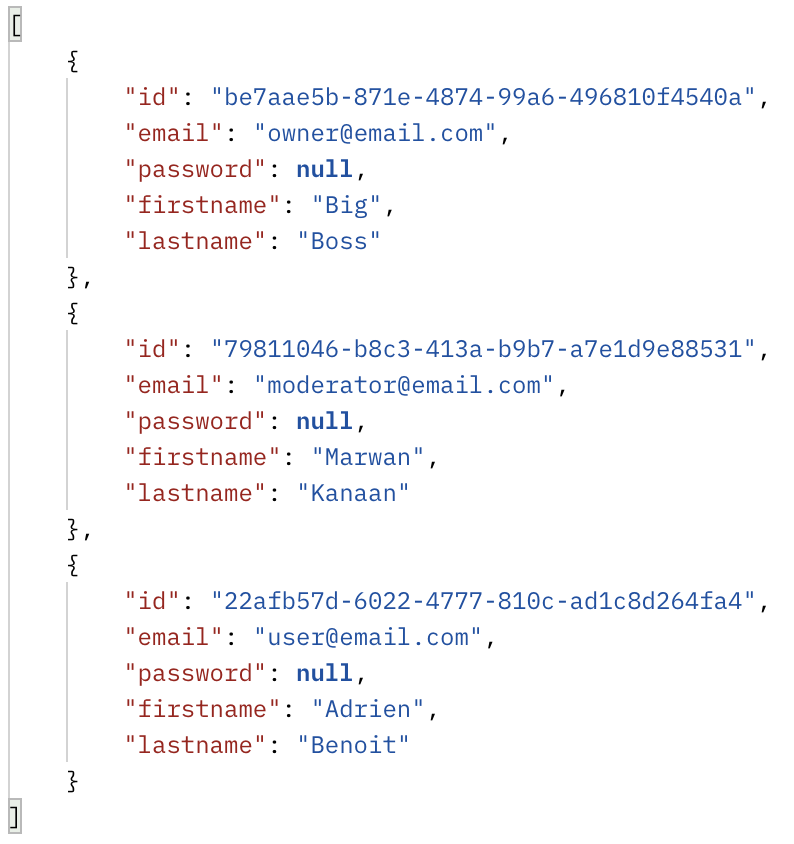
POST: /api/appuser/regular
Allows someone to register a new regular user account when they are not logged in
AppUserDto
appUserDto - the user to be registered
-
String
email -
String
password -
String
firstname -
String
lastname
{
"id": "String",
"email": "String",
"password": "",
"firstname": "String",
"lastname": "String"
}
POST: /api/appuser/moderator
Allows the owner to register a new moderator account
AppUserDto
appUserDto - the user to be registered
-
String
email -
String
password -
String
firstname -
String
lastname
{
"id": "String",
"email": "String",
"password": "",
"firstname": "String",
"lastname": "String"
}
PUT: /api/appuser/regular
Allows the Owner, Admin and Regular users to modify the names of the given account
AppUserDto
appUserDto - the user to be modified
-
String
email -
String
firstname -
String
lastname
{
"id": "String",
"email": "String",
"password": "",
"firstname": "String",
"lastname": "String"
}
Successful modification:
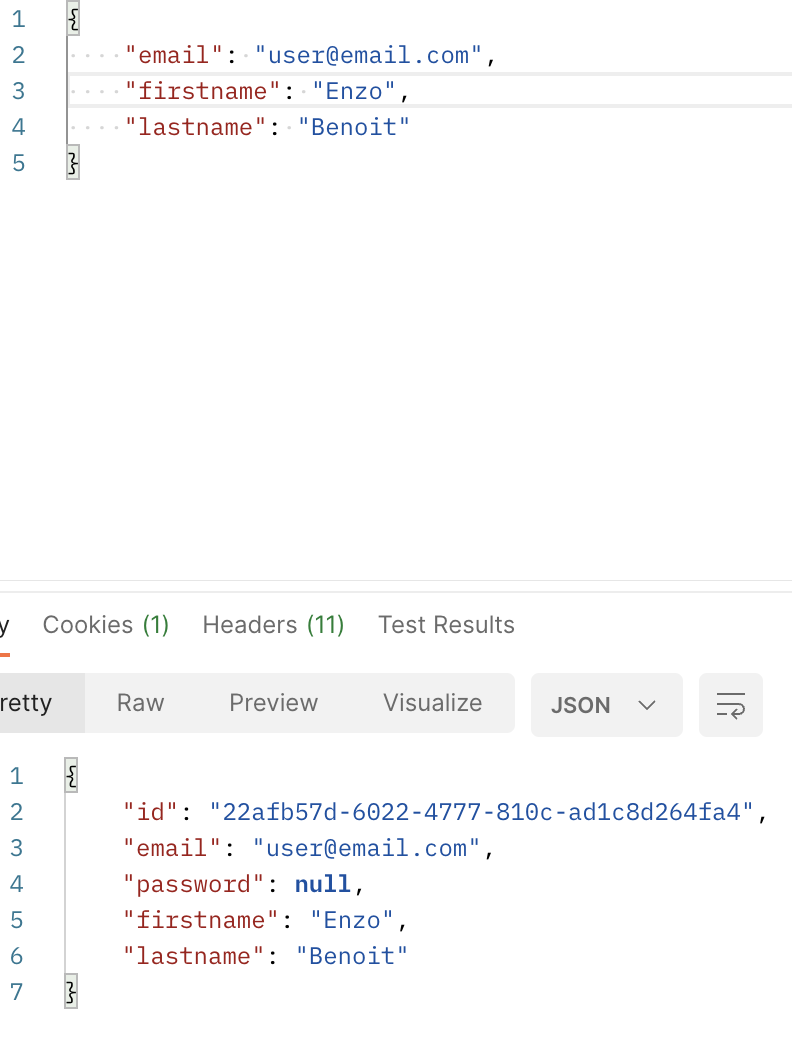
Test that errors are thrown correctly:
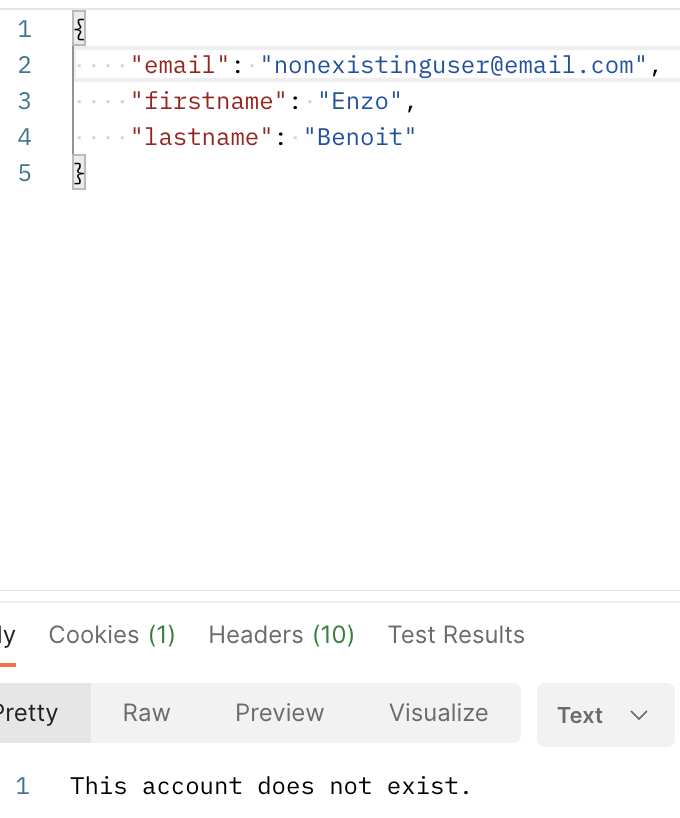
PUT: /api/appuser/regular/password
Allows the Owner, Admin and Regular users to modify the password of a given account
AppUserDto
appUserDto - the user to be modified
-
String
email -
String
password
{
"id": "String",
"email": "String",
"password": "",
"firstname": "String",
"lastname": "String"
}
Successful modification:
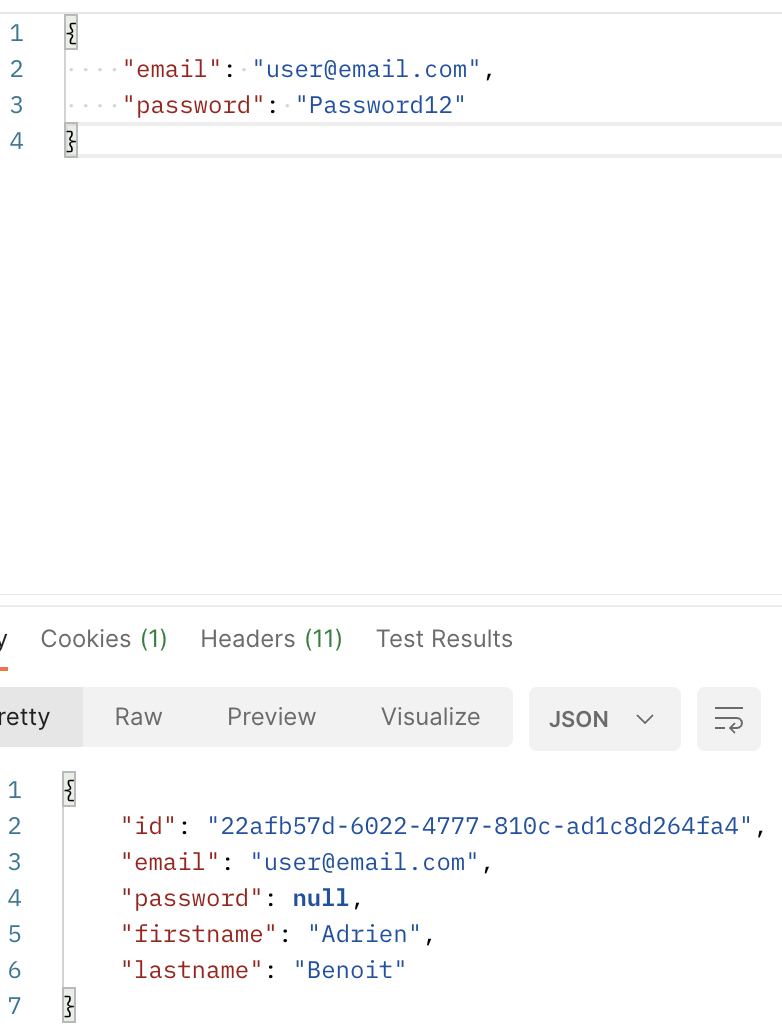
Test that errors are thrown correctly:
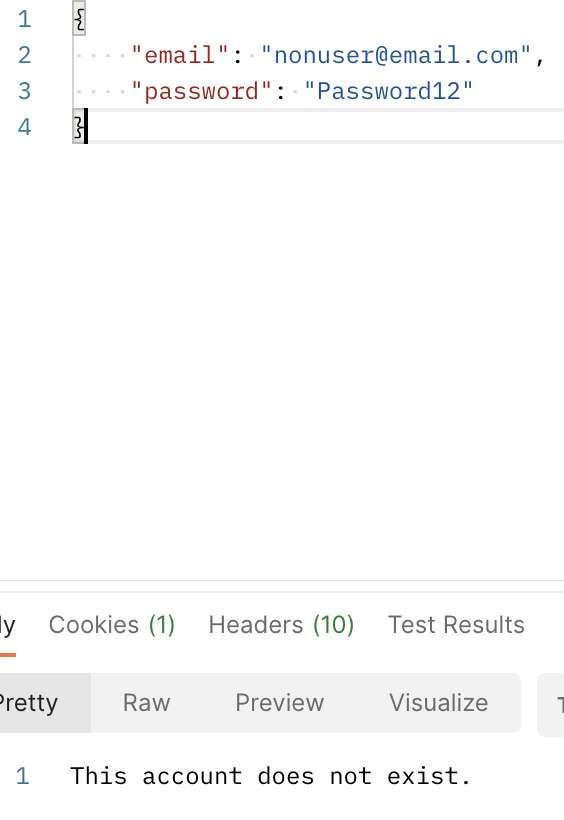
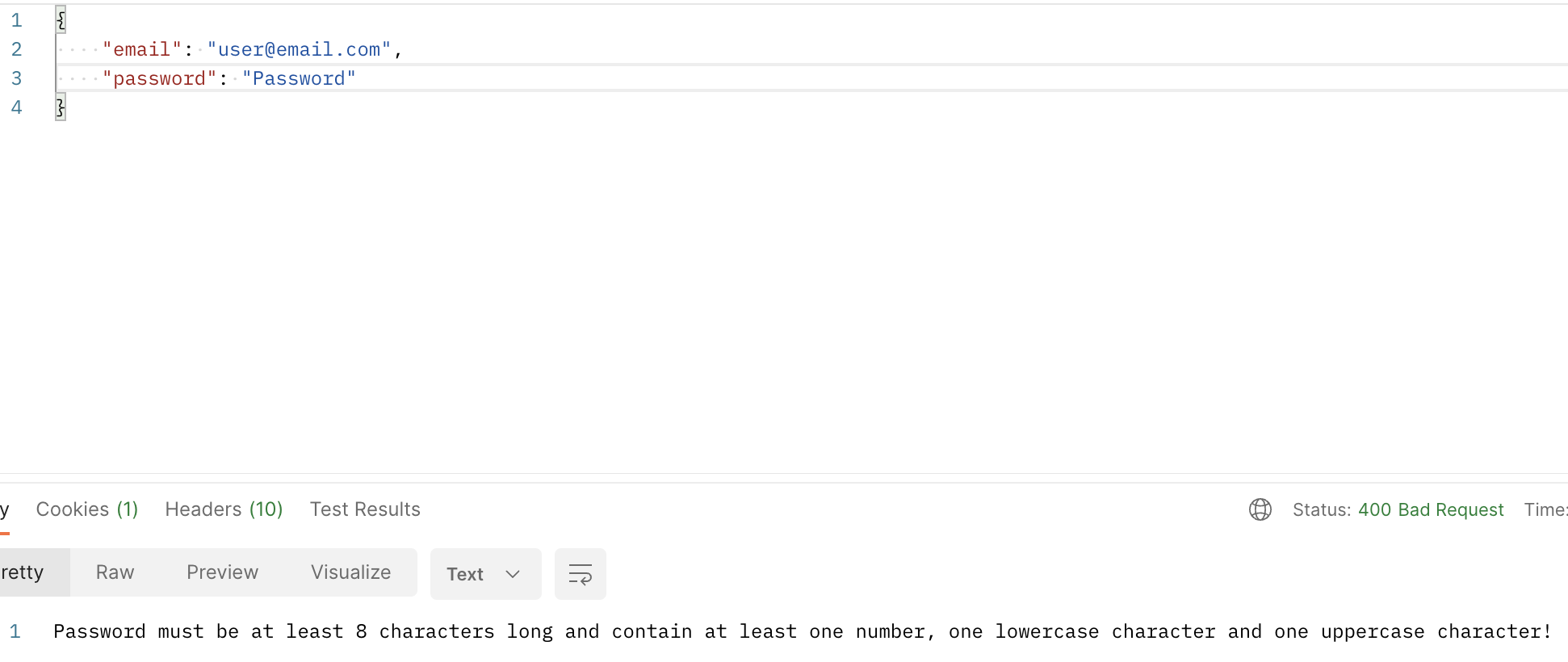
PUT: /api/appuser/moderator
Allows the Owner and Admin accounts to modify the names of the given moderator account
AppUserDto
appUserDto - the user to be modified
-
String
email -
String
firstname -
String
lastname
{
"id": "String",
"email": "String",
"password": "",
"firstname": "String",
"lastname": "String"
}
Successful modification:
Test that errors are thrown correctly:
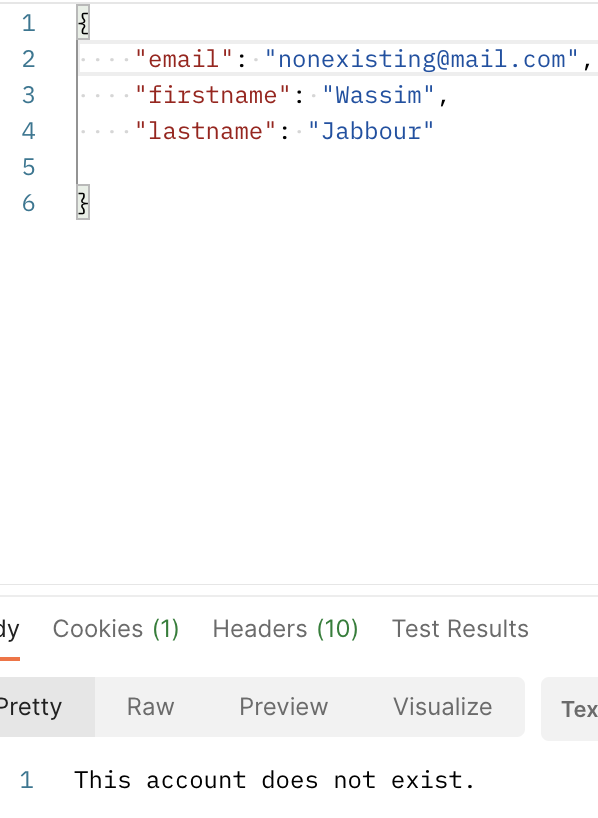
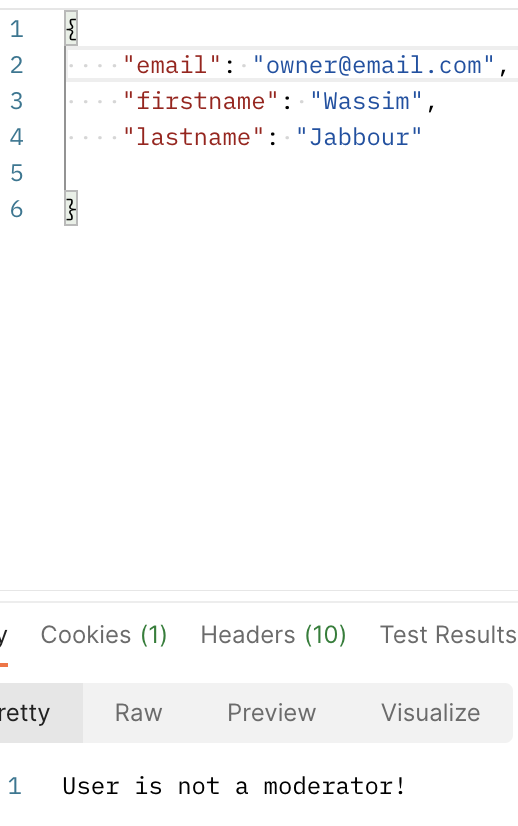
PUT: /api/appuser/moderator/password
Allows the Owner and Admin to modify the password of a moderator account
AppUserDto
appUserDto - the moderator to be modified
-
String
email -
String
password
{
"id": "String",
"email": "String",
"password": "",
"firstname": "String",
"lastname": "String"
}
Successful modification:
Test that errors are thrown correctly:
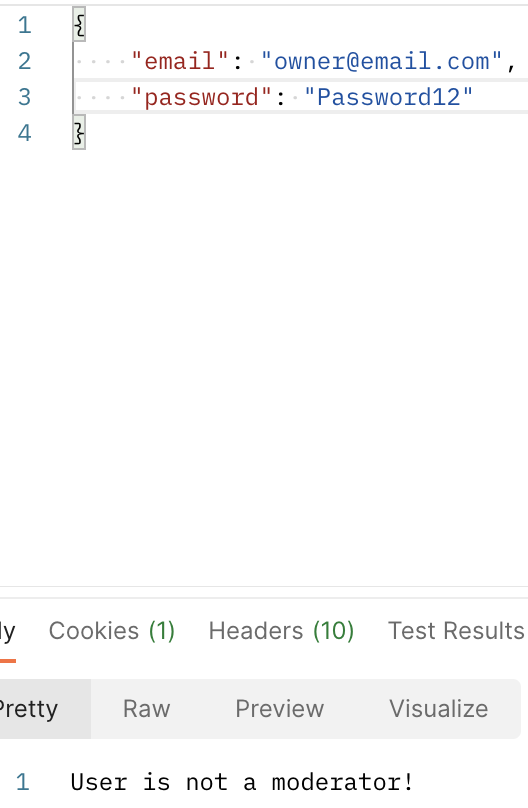
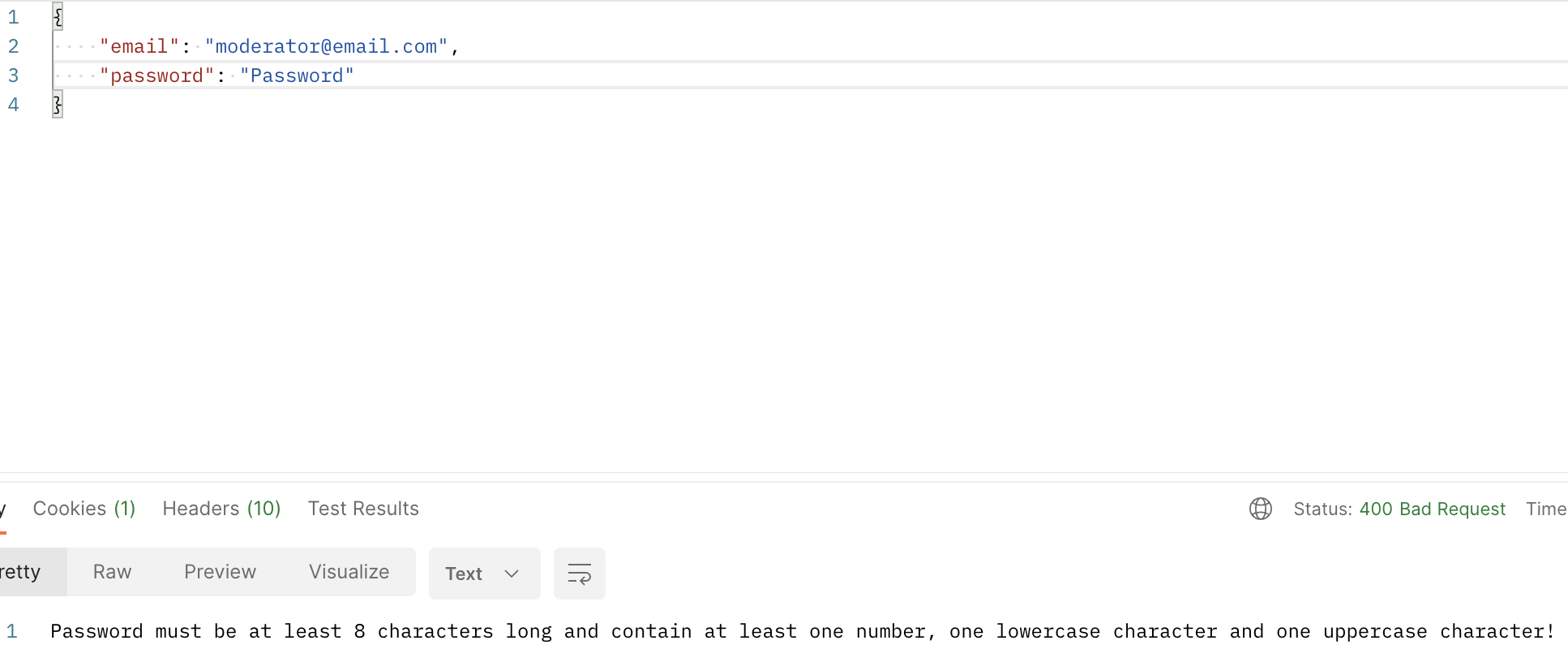
GET: /api/idea/{id}
Get an idea from the Purposeful App using its id
String id
: the idea's id
An IdeaDTO
{
"id": "string",
"isPaid": "boolean",
"isPrivate": "boolean",
"inProgress": "boolean",
"title": "string",
"purpose": "string",
"description": "string",
"date": "YYYY-MM-DD",
"domains": ["domainDTOs"],
"techs": ["techDTOs"],
"topics": ["topicDTOs"],
"imgUrls": ["urlDTOs"],
"iconUrl": {"urlDTO"}
}
GET: /api/idea/user
Get all created ideas of logged in user from the Purposeful App
N/A
An ArrayList of IdeaDTOs
[{
"id": "string",
"isPaid": "boolean",
"isPrivate": "boolean",
"inProgress": "boolean",
"title": "string",
"purpose": "string",
"description": "string",
"date": "YYYY-MM-DD",
"domains": ["domainDTOs"],
"techs": ["techDTOs"],
"topics": ["topicDTOs"],
"imgUrls": ["urlDTOs"],
"iconUrl": {"urlDTO"}]
}
POST: /api/idea
Returns all public criteria with the given search filters.
A SearchFilterDTO
{
"domains": "List<String>",
"topics": "List<String>",
"technologies": "List<String>"
}
An IdeaDTO
list
{
"id": "string",
"isPaid": "boolean",
"isPrivate": "boolean",
"inProgress": "boolean",
"title": "string",
"purpose": "string",
"description": "string",
"date": "YYYY-MM-DD",
"domains": ["domainDTOs"],
"techs": ["techDTOs"],
"topics": ["topicDTOs"],
"imgUrls": ["urlDTOs"],
"iconUrl": {"urlDTO"}
}
POST: /api/idea/create
Creates an idea in the Purposeful App
An IdeaRequestDTO
{
"id": null,
"isPaid": "boolean",
"isPrivate": "boolean",
"inProgress": "boolean",
"date": null,
"title": "string",
"purpose": "string",
"description": "string",
"domainIds": ["domainId1","domainId2"],
"techIds": ["techIds"],
"topicIds": ["topicIds"],
"imgUrls": ["urls"],
"iconUrl": {"url"}
}
Here is an example of how to create an idea. Replace the Ids of the Domain Topic and Tech with the updated ones you get from the database.
{
"id": null,
"isPaid":true,
"isPrivate":true,
"inProgress":true,
"title":"test_post",
"purpose":"testing",
"description":"just_a_test",
"date":null,
"domainIds":["bc672312-198c-47b8-839a-ba8a5ce3f745"],
"techIds":["40b47982-a40c-4364-8915-e408bcb6b571"],
"topicIds":["981e08dc-89b6-490f-b0e1-99bee9e9b188"],
"imgUrls":["https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcRjAo08bvUmiFOqrYCYh6VeCUQKPzkORdENovFsBGWG7A&s"],
"iconUrl":"https://cdn.thecoolist.com/wp-content/uploads/2016/05/Japanese-Cherry-beautiful-tree.jpg"
}
An IdeaRequestDTO
{
"id": "a323bdw2-sad8-mte9-n2k3-3nr920mds9d8",
"isPaid":true,
"isPrivate":true,
"inProgress":true,
"title":"test_post",
"purpose":"testing",
"description":"just_a_test",
"date":null,
"domainIds":["bc672312-198c-47b8-839a-ba8a5ce3f745"],
"techIds":["40b47982-a40c-4364-8915-e408bcb6b571"],
"topicIds":["981e08dc-89b6-490f-b0e1-99bee9e9b188"],
"imgUrls":["https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcRjAo08bvUmiFOqrYCYh6VeCUQKPzkORdENovFsBGWG7A&s"],
"iconUrl":"https://cdn.thecoolist.com/wp-content/uploads/2016/05/Japanese-Cherry-beautiful-tree.jpg"
}
PUT: /api/idea/edit
Modify an idea from the Purposeful App
An IdeaRequestDTO
{
"id": "string",
"isPaid": "boolean",
"isPrivate": "boolean",
"inProgress": "boolean",
"title": "string",
"purpose": "string",
"description": "string",
"date": "YYYY-MM-DD",
"domains": ["domainIds"],
"techs": ["techIds"],
"topics": ["topicIds"],
"imgUrls": ["urls"],
"iconUrl": {"url"}
}
An IdeaRequestDTO
{
"id": "string",
"isPaid": "boolean",
"isPrivate": "boolean",
"inProgress": "boolean",
"title": "string",
"purpose": "string",
"description": "string",
"date": "YYYY-MM-DD",
"domains": ["domainIds"],
"techs": ["techIds"],
"topics": ["topicIds"],
"imgUrls": ["urls"],
"iconUrl": {"url"}
}
DELETE: /api/idea/{id}
Remove an idea from the Purposeful App using its id
String
id: the idea's id
A response entity with a message instance and the HttpStatus
GET: /api/idea/user/requests
Get all ideas that the user provided has requested to collaborate on.
List of IdeaDTO
{
"id": "string",
"isPaid": "boolean",
"isPrivate": "boolean",
"inProgress": "boolean",
"title": "string",
"purpose": "string",
"description": "string",
"date": "YYYY-MM-DD",
"domains": ["domainDTOs"],
"techs": ["techDTOs"],
"topics": ["topicDTOs"],
"imgUrls": ["urlDTOs"],
"iconUrl": {"urlDTO"}
}
POST: /api/reaction, /api/reaction/
React to an idea on the Purposeful App
reactionRequestDTO
DTO of the request for a reaction to be added or removed
-
ReactionType
reactionType -
String
idea_id -
String
user_id
The newly created reaction DTO
{
"id" : null
"date" : "Date",
"reactionType": "ReactionType" ,
"idea_id": "String" ,
"user_id": "String"
}
GET: /api/collaborationResponse/{ideaId}
Get a CollaborationResponse from the Purposeful App using the ideaId and associated user (from login)
String ideaId
: the id of the idea associated with the response
A CollaborationResponseDTO
{
"id": "string",
"message": "string",
"additionalContact": "string"
}
POST: /api/collaborationResponse/approve
Approves a collaboration request
A collaborationResponseInformationDTO
.
{
"collaborationRequestId": "string",
"message": "string",
"additionalContact": "string"
}
A CollaborationResponseDTO
{
"id": "string",
"message": "string",
"additionalContact": "string"
}
POST: /api/collaborationResponse/decline
Declines a collaboration request
A CollaborationResponseDTO
{
"id": "string",
"message": "string",
}
A CollaborationResponseDTO
{
"id": "string",
"message": "string",
"additionalContact": "string"
}
POST: /api/collaborationRequest
Sends a collaboration request
collaborationRequestDTO
The DTO containing the idea, the message, and the additional contact information
{
"ideaId" : "String",
"message": "String" ,
"additionalContact": "String"
}
A CollaborationRequestDTO
{
"id": "String",
"ideaId": "String",
"message": "String",
"additionalContact": "String",
"hasResponse": "boolean"
}