Custom Effect Settings
Choose the color for the custom effect.
This guide will help you to create your own effect. We will create an effect called "effect_custom". Replace the name with your effect name. You can find all modified files here: [Custom Effect Demo Files](https://gist.github.com/TobKra96/2e3fb76395aeb67c77afffc77e07b8f5) **Do not copy and paste the whole file. These files may be outdated! Use the files from the master branch and add the required code lines.** ## Create the effect Create a new file inside the directory `music_led_strip_control/server/libs/effects/`: ```shell sudo touch /share/music_led_strip_control/server/libs/effects/effect_custom.py ``` Add the following content: ```python from libs.effects.effect import Effect # pylint: disable=E0611, E0401 import numpy as np from scipy.ndimage.filters import gaussian_filter1d class EffectCustom(Effect): def __init__(self, device): # Call the constructor of the base class. super(EffectCustom, self).__init__(device) # Init your custom variables here. def run(self): effect_config = self._device.device_config["effects"]["effect_custom"] led_count = self._device.device_config["LED_Count"] led_mid = self._device.device_config["LED_Mid"] audio_data = self.get_audio_data() y = self.get_mel(audio_data) if y is None: return # Build an empty array. output_array = np.zeros((3, self._device.device_config["LED_Count"])) # Use this output method for music responsive effects. # It will put the output array into a queue. # After this it continues directly with the next music signal. self.queue_output_array_noneblocking(output_array) # Use this output method for non-music responsive effects. # It will block the effect until the array was output. self.queue_output_array_blocking(output_array) ``` Above is the file where you will manipulate the output array. Code description: Create a new class EffectCustom that inherits from Effect. ```python class EffectCustom(Effect): ``` This will be called during the start of the effect. It will be executed once. Add all variables that should be stored between the output cycle. E.g. the previous output array. ```python def __init__(self, device): ``` This method will be called each time the program wants to update the LED strip. E.g. 60 times per second. Add the array manipulation here. ```python def run(self): ``` Configs of your effect, that are stored inside the config.json. ```python effect_config = self._device.device_config["effects"]["effect_custom"] ``` The count of the LEDs. ```python led_count = self._device.device_config["LED_Count"] ``` The mid that you set for this LED strip. ```python led_mid = self._device.device_config["LED_Mid"] ``` This variable is the audio signal that was captured between the last call of the run and now. It is an array of 24 elements and represents the level of a specific frequency range. It goes from lows to highs. See the other effects to understand it better. ```python y ``` This is the array you will output. It is three-dimensional with the length of the LED strip. E.g. `output[0][:]` the `[0]` represents the red color channel. `[:]` selects all LEDs. See the other effects for inspiration. ```python output_array ``` This will put the `output_array` into a queue which will output it. Use the blocking method for non-music effects and the non-blocking one for music reactive effects. ```python self.queue_output_array_noneblocking(output_array) self.queue_output_array_blocking(output_array) ``` ## Set all dependencies ### Configuration Add the configuration of your effect inside the config 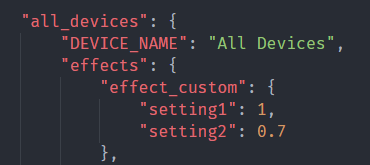 Remember the following line: ```python effect_config = self._device.device_config["effects"]["effect_custom"] ``` Open `music_led_strip_control/server/libs/config_template.json` (this is the template config, which will be used if you reset your settings) and add your effect. Find `all_devices` and `default_device`, then `effects`. Now you should see some other effect settings like `effect_bars`. Add your own settings: ```json "effect_custom": { "setting1": 1, "setting2": 0.7 }, ``` Do the same steps in `/share/.mlsc/config.json`. You have to add it inside all of your existing devices and both the `all_devices` and `default_device` blocks. ### EffectsEnum Add a new entry in `music_led_strip_control/server/libs/effects_enum.py`: ```python effect_custom = 26 ``` 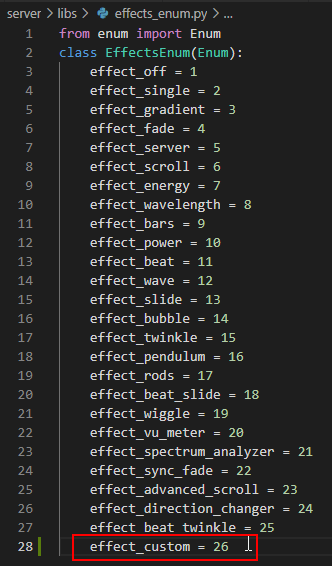 ### Effect Service Add your effect to the imports in `music_led_strip_control/server/libs/effect_service.py`: ```python from libs.effects.effect_custom import EffectCustom # pylint: disable=E0611, E0401` ``` 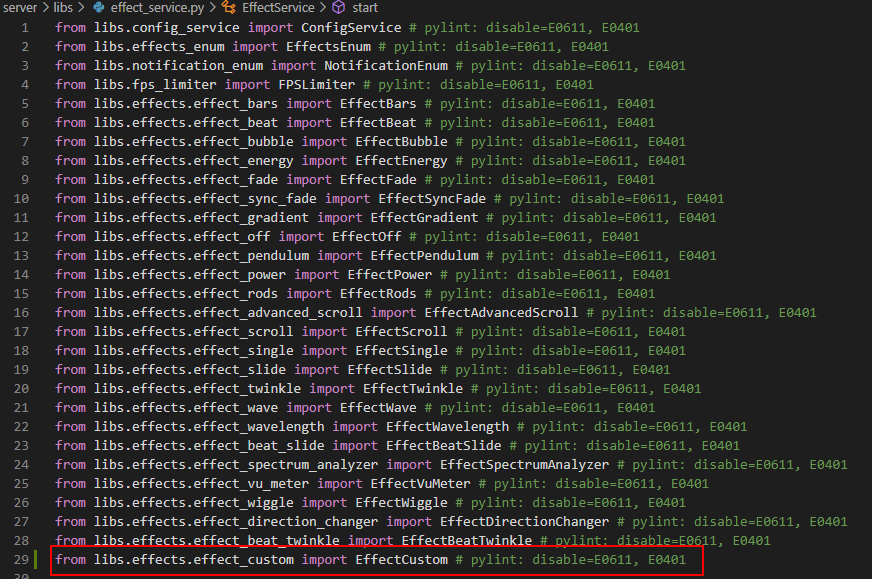 Add your effect to the available effects in `self._available_effects`: ```python EffectsEnum.effect_custom: EffectCustom ``` This will link your effect class with the enum. 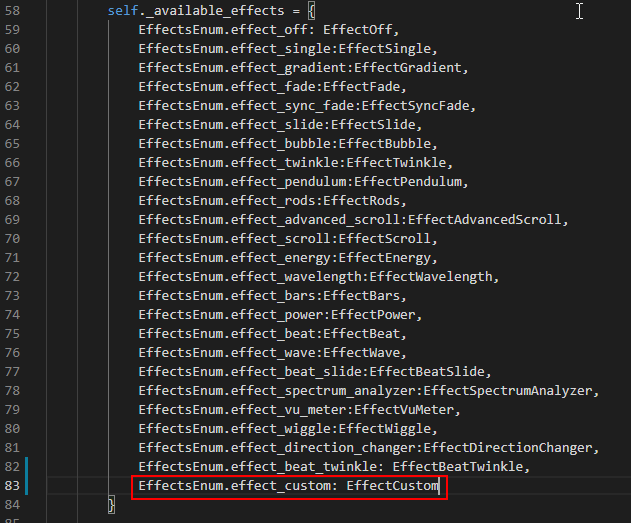 ## Web interface integration ### Dashboard Add a new tile to the Dashboard. 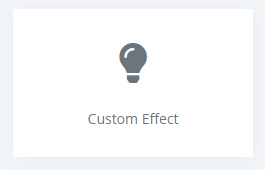 Open `/share/music_led_strip_control/server/libs/app/home/templates/dashboard.html` and create a new `div` element: ```html
Custom Effect
Choose the color for the custom effect.