2. Meesterproef week 2 - TheKevSter35/meesterproef-1920 GitHub Wiki
After a lot of brainstorming and help for our couch we decide to make a platform that improve the digital skills from a Computer illiterate. The platform has multiple components. From a Phishing Quiz to an informative component for example (What do you think what data is sent to the company). The user will get points to let the see there digital skills improve.
The target audience are from Amsterdam the client want a map from Amsterdam to show what the city do with the data that is collected. But we don't have access to that data set. Luckily we are allowed to create are own for example the library and public transport.
- A use of a map of Amsterdam to navigate through all the components.
- a toggle for map and list
- point system
- Option to save your points
- teach the user the use of data in an approachable way (a game or an education )
Because we are working in a team. We decided that have make a guideline for how we going to code.
What we use
- Express
- NodeJS
- EJS
- HTML
- CSS
- JS
Gulp
For CSS we used gulp-clean-css te make all our css files in to 1 minified file. Because we are working in different branches and every page has it own css file is this the perfect way to get less merge errors.
const gulp = require('gulp')
const cleanCSS = require('gulp-clean-css')
const concat = require('gulp-concat-css')
gulp.task('buildCSS', () => {
return gulp.src('public/css/*.css')
.pipe(cleanCSS({compatibility: 'ie8'}))
.pipe(concat('style.css'))
.pipe(gulp.dest('public/css/minified'))
})
gulp.task('watch', function(){
gulp.watch('public/css/*.css', gulp.series('buildCSS'))
})
ESlint For JS we used ESlint so that all our JS is write in the same guideline.
{
"env": {
"browser": true,
"commonjs": true,
"es6": true
},
"globals": {
"Atomics": "readonly",
"SharedArrayBuffer": "readonly"
},
"parserOptions": {
"ecmaVersion": 11
},
"rules": {
"no-multi-spaces": "error",
"indent": [
"error",
"tab"
],
"linebreak-style": [
"error",
"windows"
],
"quotes": [
"error",
"single"
],
"semi": [
"error",
"never"
]
}
}
corporate identity (Wijzer.amsterdam)
We are working together with Ontwerplab that developed (Wijzer.amsterdam) We decide to make link it with our platform. In the website it already gives tips for the user for example: how to make a mail. But it doesn't teach the user how to use the internet how it works.
We used figma to design the platform. There was no style guide for (Wijzer.amsterdam). We used the sidebar to show the process and the score for the user. We added an introduction page to explain what Digi-weet is and what for subject's the user will come across.
Randy was working on the map and Kevin.k working on the score system. I was working on the Quiz. Like a typical quiz the user choose an answer and see if it is correct (green) or incorrect (red) but the user will also get an explanation about the question. After 10 questions the user will see the total score.
For HTML i created the quiz in a <form>
tag so it can be submitted to a different page to show what the users score is. Kevin.k used data-score
to validated the right answer.
<form action="/quiz-result" method="POST">
<div id="question1">
<section>
<h2>Phishing Quiz: Vraag 1/10</h2>
<img src="../source/pictures/quiz/bank-mail.png" alt="">
</section>
<section>
<h2 id="text-question1"> Je opent jou e-mail en ziet een bericht van de bank. Daarin staat dat je bankrekening is
gedeactiveerd ( tijdelijk op stop is gezet). Er wordt in het bericht gevraagd om in te loggen, zodat jou bankrekening
weer geactiveerd wordt.
</h2>
<h2 class="info-text-question1 hidden"> <strong>Uitleg: </strong>De bank vraagt nooit om in te loggen op Mijn Bank
(Internetbankieren) via een sms of e-mail.
</h2>
<label for="question1-answer1" class="question1-answer1">
<input type="radio" id="question1-answer1" name="question1" data-score="0" value="0">
Dit is wel belangrijk de bank stuurt dit niet zomaar.
</label>
<label for="question1-answer2" class="question1-answer2">
<input type="radio" id="question1-answer2" name="question1" data-score="1" value="1">
Misschien moet ik toch nog contact opnemen met mijn bank en vragen waarom mijn pas is gedeactiveerd.</label>
<p><a href="#question2" class="next-question1 hidden">Volgende vraag </a></p>
</section>
</div>
For CSS I used ''' DISPLAY: flex;''' to make the questions horizontal and with ''' overflow: hidden;''' to hide the scroll bar. Then for the smooth transition is used '''scroll-behavior: smooth;''' to go to the next question smoothly if the user click on the next button.
#quiz #question {
DISPLAY: flex;
flex-direction: row;
}
#quiz form {
overflow: hidden;
scroll-behavior: smooth;
width: 90%;
margin: 0 auto;
}
#quiz form div {
display: flex;
justify-content: center;
align-items: center;
background-color: #f6f6f6;
color: #000;
border-radius: 2em;
flex-shrink: 0;
height: auto;
transform: scale(1);
min-width: auto;
transform-origin: center center;
scroll-snap-align: start;
width: 100%;
height: auto;
margin-bottom: 2em;
}
#quiz label {
border-radius: 1em;
background-color: #002b37;
margin-bottom: 1em;
padding: 1em;
display: flex;
flex-direction: column;
cursor: pointer;
position: relative;
position: relative;
margin: 0 0 3em;
font-size: 1em;
color: #fff;
min-height: 3em;
max-width: 100%;
text-align: center;
}
#quiz label input {
position: absolute;
opacity: 0;
z-index: -1;
}
.hidden {
display: none;
}
.label-hidden{
z-index: -999;
}
And for JS first I made a fallback for the form. So if JS is turned off the user can still use the quiz (only '''scroll-behavior: smooth;''' will not work).
//Fallback___________________________________
document.querySelector('form').style.display = 'flex'
When js is turned on. When the user click on a answer the first '''setTimeout''' will change the color of the button. And by the second '''setTimeout''' it add a hidden class to the question and remove the hidden class from the info text to give the user information for the user. Finally, it shows the next question button.
//Question 1___________________________________
const correctQuestion1 = document.getElementById('question1-answer2')
correctQuestion1.addEventListener('click', scrollToForm)
function scrollToForm() {
document.querySelector('.question1-answer2').style.backgroundColor = '#00607b'
setTimeout(function () {
document.querySelector('.question1-answer2').style.backgroundColor = '#6abb40'
}, 1500)
setTimeout(function () {
const textQuestion1 = document.getElementById('text-question1')
textQuestion1.classList.add('hidden')
const CorrectTextQuestion1 = document.querySelector('.info-text-question1')
CorrectTextQuestion1.classList.remove('hidden')
const next = document.querySelector('.next-question1')
next.classList.remove('hidden')
}, 2000)
}
We showed our demo for our first prototype. It was not much but it showed what we were working on and how the basic functions work. for the map we used openstreet map because it was easy to use and you can custom the markers.
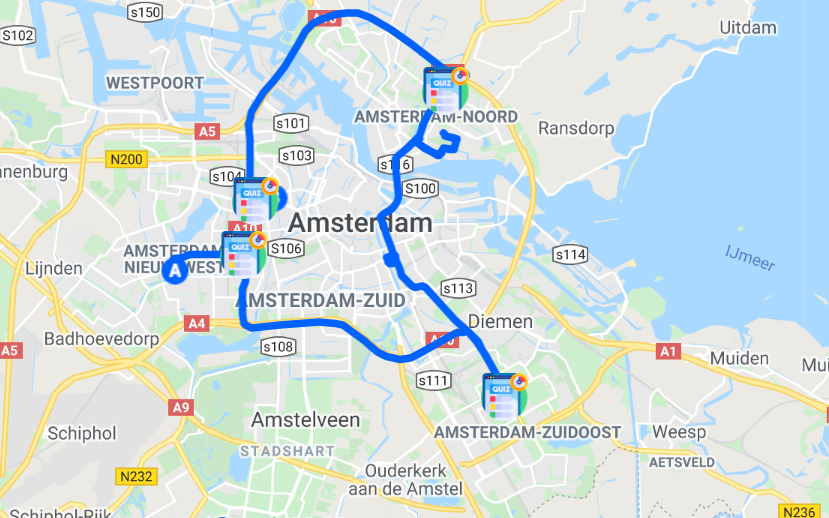
We showed our demo for our first prototype. It was not much but it showed what we were working on and how the basic functions work. For the map we used openstreet map because it was easy to use and you can custom the markers.
The quiz was for the most part positive. It was easy to use and it contains real examples (with different names for company's). But there were some spellings errors.
Feedback
- Try to make a story to follow for the user like: (Use the bus, or the store).
- Tell the user why they are using this platform. (A introduction).
- The client use already a map for the location form all the OBA's in Amsterdam We can use that for our platform.
- Add a chat function (provided from the client) so the user can ask for help.
For the quiz
- Show the user which question they are (question: 2/10 )
- Add a background for the info text.
- some images are not readable (The second one)
- add the chat function for the user if they can't figure it out.
Based on the feedback we added a page that explains what Digi-weet is. On the sidebar you can see your progress with the platform. And I hide the normal nav on a Hamburger Menu so the user can focus on the core function of the platform.
We were glad that we start over with our project. We have now a clear idea what we wanna make. The clients like the idea and the map is an interesting idea but we were not sure if the target audience can use it or just use the list view. That's something we have to test. The core function works the user get points for the quiz. We wanna add a second tool/game to the platform that is totally different from a quiz. I think we are in the right direction.