Player sdk - Straas/Straas-iOS-sdk GitHub Wiki
StraaS Player SDK is a player library for iOS platform. You may use this SDK to play an CMS resource and get something resource you need from CMS.
Requires Swift 4.0/Xcode 9.3 or later.
If you need Swift 3.x/Xcode 9.2(or eariler) use the v0.13.0.
Add following lines to your Podfile:
pod 'StraaS-iOS-SDK/Player'
Then run pod install
in the command line.
(You might need to do pod repo update
before pod install
.)
Be sure to fill up SDK Authentication.
After successfully configured application, it means you can retrieve media via STSCMSManager
and play media via STSSDKPlayerView
as guest. To configure member, refer to here.
To Initialize a STSSDKPlayerView
, you can use either code or nib to do this just like any other UIView class.
- Create from code:
//Initialize a playerView which has 320 width, 180 height
STSSDKPlayerView * playerView = [[STSSDKPlayerView alloc] initWithFrame:CGRectMake(0,0,320,180)];
//Add playerView to the view you want to add. Assume add to current ViewController.
[self.view addSubview:playerView];
- Create from nib:
Create a nib view and link to STSSDKPlayerView
To identify member, you have to set member JWT to STSCMSManager
sharedManager
[[STSCMSManager sharedManager] setJWT:@"your_member_JWT" completion:^(BOOL success){
//If success, means you can retrieve and play media with the member's authority.
}];
iOS StraaSPlay is simple to be used. We have implement the basic view as
STSSDKPlayerView
, which include the basic function a player should have,
like play, puase, seek, and replay. You should conform the STSPlayerPlaybackEventDelegate and STSSDKPlayerViewDelegate protocols
in the ViewController you want to contain STSSDKPlayerView. This two protocols will be needed in whatever mode you want to play.
You can call the method loadVideoWithId:
in STSSDKPlayerView
to play a video. For example:
STSSDKPlayerView * playerView = [STSSDKPlayerView new];
playerView.delegate = self;
[playerView loadVideoWithId:@"<#the_video_id_you_want_to_play#>"];
The delegate
property in STSSDKPlayerView
will send basic playback event which defined in STSPlayerPlaybackEventDelegate
. STSSDKPlayerViewDelegate
is also needed.
You can call the method loadLiveWithId:
in STSSDKPlayerView
to play a live. For example:
STSSDKPlayerView * playerView = [STSSDKPlayerView new];
playerView.liveEventDelegate = self;
playerView.delegate = self;
[playerView loadLiveWithId:@"<#the_Live_id_you_want_to_play#>"];
The delegate
property in STSSDKPlayerView will send basic playback event which defined in STSPlayerPlaybackEventDelegate
. STSSDKPlayerViewDelegate
also needed.
The liveEventDelegate
property in STSSDKPlayerView
will send several callback event let you easily catch the live related event and do something needed when callback receive.
You can call the method loadPlaylistWithId:
in STSSDKPlayerView
to play a playlist. For example:
STSSDKPlayerView * playerView = [STSSDKPlayerView new];
playerView.playlistEventDelegate = self;
playerView.delegate = self;
[playerView loadLiveWithId:@"<#the_playlist_id_you_want_to_play#>"];
The delegate
property in STSSDKPlayerView
will send basic playback event which defined in STSPlayerPlaybackEventDelegate
. STSSDKPlayerViewDelegate
is also needed.
The playlistEventDelegate
property in STSSDKPlayerView
will send several callback event let you easily catch the playlist related event and do something needed when callback receive.
STSCMSManager
provides several methods to get the CMS resources you want. You don't have to worry about the tedious works like Json mapping or http connection, since we've done all the things in STSCMSManager
.
You should no need to get one video, playlist, live by STSCMSManager, since STSSDKPlayerView
will help you get the resources. You may go to the last Chapter to see the details.
To get video list, you may call
[[STSCMSManager sharedManager] getVideoListWithPage:<#(NSUInteger)#>
success:<#^(NSArray<STSLive *> * _Nonnull, STSPagination * _Nonnull)success#>
failure:<#^(NSError * _Nonnull)failure#>];
To get live list, you may call
[[STSCMSManager sharedManager] getLiveListWithPage:<#(NSUInteger)#>
success:<#^(NSArray<STSLive *> * _Nonnull, STSPagination * _Nonnull)success#>
failure:<#^(NSError * _Nonnull)failure#>];
To get playlist list, you may call
[[STSCMSManager sharedManager] getPlaylistsWithPage:<#(NSUInteger)#>
success:<#^(NSArray<STSPlaylist *> * _Nonnull, STSPagination * _Nonnull)success#>
failure:<#^(NSError * _Nonnull)failure#>];
Although we have implement the basic playerView UI for the general use case, you may still want to make some customizations. StraaSPlayerSDK provides ways to fulfill your wishes too!
Here is the ways you can do.
- change the
subviewLayoutConstraints
In every UIView subclass from StraaSPlayerSDK provides the subviewLayoutConstraints
to let you change the default subview's layout constraint. You may change the layout position logic by replacing the origin constraints.
2. set canShowSomething
to NO
This is a convenient way to make the UIView you don't need to be hide. For example, if you don't want to see playbackTimelineView
on the STSSDKPlayerControlView
, you may set
STSSDKPlayerView * playerView = [STSSDKPlayerView new];
playerView.playerControlView.canShowPlaybackTimelineView = NO;
Then the playbackTimelineView
will be hide and you will not see playbackTimelineView
on the playerView
unless you set canShowPlaybackTimelineView back to YES.
playerView.playerControlView.canShowPlaybackTimelineView = YES;
Then the playbackTimelineView
would be showed again!
You can add custom video quality naming by using property mappingForDisplayingAvailableQualityNames
in STSSDKPlayerView.h. There is sample code to demo how to use mappingForDisplayingAvailableQualityNames in STSPlayerViewController.m as followed:
// You can uncomment the below part to see the demo of using `mappingForDisplayingAvailableQualityNames`
self.playerView.mappingForDisplayingAvailableQualityNames = ^NSArray<NSString *> *(NSArray<NSString *> * _Nonnull qualityNames) {
NSMutableArray *newQualityNames = [[NSMutableArray alloc] init];
for (NSString *qualityName in qualityNames) {
if ([qualityName isEqualToString:@"auto"]) {
[newQualityNames addObject:NSLocalizedString(@"Auto", @"Auto")];
} else if ([qualityName isEqualToString:@"240p"]) {
[newQualityNames addObject:NSLocalizedString(@"Normal Quality", @"Normal Quality")];
} else if ([qualityName isEqualToString:@"360p"]) {
[newQualityNames addObject:NSLocalizedString(@"High Quality", @"High Quality")];
}
}
return [newQualityNames copy];
};
By default, when the app goes to the background, or the device is locked, iOS will pause any media that is playing. If you want to change this behavior, you should take the following steps:
- Declaring the app plays audible content in the background.
In Xcode 8, select a target, then under Capabilities > Background Modes, enable "Audio, Airplay, and Picture in Picture".
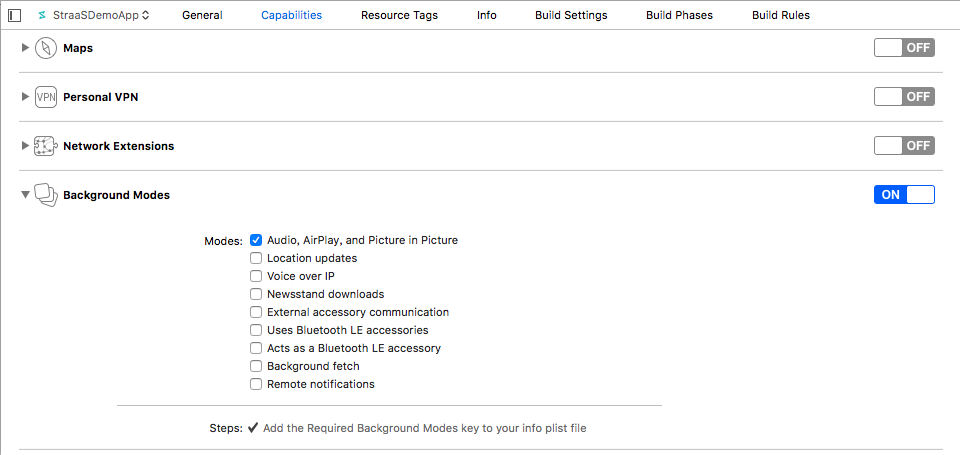
-
Set
allowsPlayingInBackground
property of theSTSSDKPlayerView
toYES
(The default value isNO
). -
If you want to let the app users to control the playback on the lock screen and in the control center when the player is playing a media, set
remoteControlEnabled
property of theSTSSDKPlayerView
toYES
(The default value isNO
).
DVR feature allows you to pause, rewind, and play DVR-enabled live events. Once you resume playing, the event will start from where you hit pause so that you won't miss a thing,
DVR playback will only be available for a limited amount of time after the live event ends. Currently, the recording will remain open for 10 minutes.
-
With StraaS iOS player SDK default UI
- Merely call
loadLiveWithId:
then the player will handle everything. - Seeking: Miss something? Seek back and forth in the event by dragging the scrubber backward and forwards. You'll see the timestamp change as you move the scrubber around, so you'll know where you are.
- Live: Ready to get back to the real-time coverage of the event? Just click the Live button, and we'll take you there.
- Merely call
-
With customize UI
-
Set
delegate
of the player view and implementplayerView:seekableTimeRangesChanged:
andplayerView:mediaCurrentTimeChanged:
methods to update your progress bar (or something else).UISlider * yourProgressBar; Float64 startTime = -1; - (void)playerView:(STSSDKPlayerView *)playerView seekableTimeRangesChanged:(NSArray<NSValue *> *)seekableTimeRanges { // Update the progress bar of the player according to `seekableTimeRanges`. CMTimeRange timeRange = kCMTimeRangeInvalid; if ([seekableTimeRanges count] > 0) { NSValue * value = [seekableTimeRanges firstObject]; [value getValue:&timeRange]; } if (CMTIMERANGE_IS_VALID(timeRange) && CMTimeGetSeconds(timeRange.duration) > 0) { Float64 durationInSeconds = CMTimeGetSeconds(timeRange.duration); yourProgressBar.maximumValue = durationInSeconds; startTime = CMTimeGetSeconds(timeRange.start); } } - (void)playerView:(STSSDKPlayerView *)playerView mediaCurrentTimeChanged:(Float64)currentTime { // Update the progress bar of the player according to `currentTime`. if (startTime >= 0) { return; } Float64 timeInterval = playbackTime - startTime; yourProgressBar.value = timeInterval; }
-
Set
liveEventDelegate
of the player view and implementplayerView:liveId:playbackModeChanged:
so that you can update your UI with whether the playback is in live DVR mode.- (void)playerView:(STSSDKPlayerView *)playerView liveId:(NSString *)liveId playbackModeChanged:(BOOL)isInDvrMode { // Update player UI according to `isInDvrMode`. }
-
Call
STSSDKPlayerView
'sseekToTime:
method to implement seek feature. -
Call
STSSDKPlayerView
'splayAtLiveEdge
method when users want to seek the current playback position back to the real-time coverage of live(Live).
-
You can log the location information for usage analysis by using the location property in STSSDKPlayerView.h
.
You can follow the example to update location:
- (void)locationManager:(CLLocationManager *)manager didUpdateLocations:(NSArray<CLLocation *> *)locations {
self.playerView.location = locations.lastObject;
}
You can get the errors from -(void)playerView:(STSSDKPlayerView *)playerView error:(NSError *)error;
and reference this Player Errors
document: Player Errors