Dashboard - NeoSOFT-Technologies/workflow-plugins GitHub Wiki
Create a new, empty ASP.NET Core project and add the following NuGet packages.
We need to install some packages from NuGet Package Manager to use ELSA workflow.
- Elsa
- Elsa.Activities.Http
- Elsa.Server.Api
- Elsa.Designer.Components.Web
- Elsa.Activities.Temporal.Quartz
- Elsa.Persistence.EntityFramework.SqlServer
We need to modify Startup.cs file as shown below,
public void ConfigureServices(IServiceCollection services)
{
var elsaSection = Configuration.GetSection("Elsa");
// Elsa services.
services
.AddElsa(elsa => elsa
.AddConsoleActivities()
.AddHttpActivities(elsaSection.GetSection("Server").Bind)
.AddQuartzTemporalActivities()
.AddWorkflowsFrom<Startup>()
);
// Elsa API endpoints.
services.AddElsaApiEndpoints();
// For Dashboard.
services.AddRazorPages();
}
Now, create a Razor Page named _Host. Add the following content to _Host.cshtml;
@page "/"
@{
var serverUrl = $"{Request.Scheme}://{Request.Host}";
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Elsa Workflows</title>
<link rel="icon" type="image/png" sizes="32x32" href="/_content/Elsa.Designer.Components.Web/elsa-workflows-studio/assets/images/favicon-32x32.png">
<link rel="icon" type="image/png" sizes="16x16" href="/_content/Elsa.Designer.Components.Web/elsa-workflows-studio/assets/images/favicon-16x16.png">
<link rel="stylesheet" href="/_content/Elsa.Designer.Components.Web/elsa-workflows-studio/assets/fonts/inter/inter.css">
<link rel="stylesheet" href="/_content/Elsa.Designer.Components.Web/elsa-workflows-studio/elsa-workflows-studio.css">
<script src="/_content/Elsa.Designer.Components.Web/monaco-editor/min/vs/loader.js"></script>
<script type="module" src="/_content/Elsa.Designer.Components.Web/elsa-workflows-studio/elsa-workflows-studio.esm.js"></script>
</head>
<body>
<elsa-studio-root server-url="@serverUrl" monaco-lib-path="_content/Elsa.Designer.Components.Web/monaco-editor/min">
<elsa-studio-dashboard></elsa-studio-dashboard>
</elsa-studio-root>
</body>
</html>
NOTE : The application will always serve the _Host.cshtml page.
Add following line of code in Configure method of Startup.cs file.
app.UseEndpoints(endpoints =>
{
// Elsa API Endpoints are implemented as regular ASP.NET Core API controllers.
endpoints.MapControllers();
// For Dashboard.
endpoints.MapFallbackToPage("/_Host");
});
We need to also specify the BaseUrl on which ELSA workflow will be running.
"Elsa": {
"Server": {
"BaseUrl": "https://localhost:5001"
},
}
Now run the program and open a web browser to the home page.
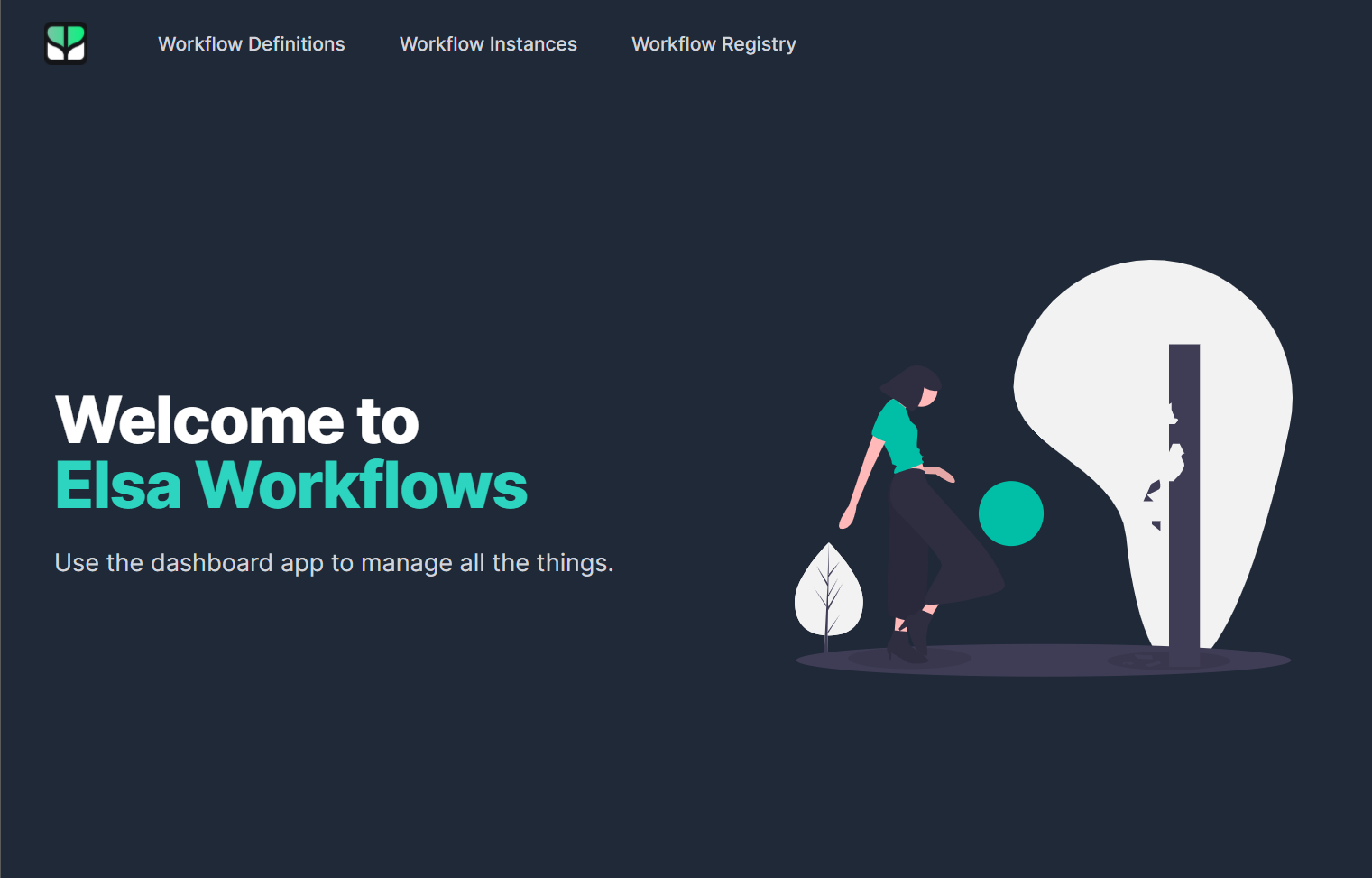
Click on Workflow Definitions on dashboard and then click on Create Workflow,
Then on Design page, we have to click on Start button to start a new workflow,
After clicking Start button, it will show the lists of activities,