06.Caching Techniques - JohnyzHub/jax-rs GitHub Wiki
In case of HTTP, the caching is little different as it doesn't have any intermediary storage component. It has several ways of notifying the client about the availability of updated data and thereby reduces the network traffic by avoid sending the duplicate data. But not between restful web service and the database.
JAX-RS API embraces the HTTP caching features for managing the cache of the results returned by restful web services.
The key to making caching work effectively is to use HTTP caching headers.-
Strong caching headers: These headers specify how long a cached resource is valid.
- Expires
- Cache-Control max-age
- Weak caching headers: These headers help the browser decide if it needs to fetch an item from cache by issuing a conditional GET request.
- Last-Modified
- ETag
This is a general header field. This is used to specify directives for caching mechanisms in both requests and responses. Cache-control gives more control over web caching. Refer this link to know more about cache-control Cache-control supports several directives. The popular one is max-age. This directive suggests (in seconds denominations) how long the cached resource is valid.
For working examples, refer
Server: MovieCachedService.listMovies()
Client: MovieCacheClient.findMovies_maxage()
This is useful in reading(ie GET) the data only if modified. The precondition validation will be passed if set with post-modified date.
This is useful in PUT/POST calls to perform the insert/update operation only if the object is not modified to avoid duplicate operation to address concurrency issues.
For working examples, refer
Server:
MovieCachedService.getMovie()
MovieCachedService.updateMovie_unmodified()
Client:
MovieCacheClient.findMovie_ifModified()
MovieCacheClient.findMovie_ifUnmodified()
MovieCacheClient.updateMovie_ifUnmodified()
EntityTag is an unique opaque identifier, that represents the state of the entity returned in the response object. Typically this is hashcode of the object. This etag is useful in the scenarios where the service consumer(ie. client) is interested in the latest object not just because the object is updated(ie. last modified date is changed) but a set(one or more) of the object attributes are changed.
The precondition validation will be passed if set with matching entity tag.This is useful in PUT/POST calls to perform the insert/update operation only if the object is not modified to avoid duplicate operation to address concurrency issues. The precondition validation will be passed if set with non matching entity tag.
This is useful in reading the latest object, only if modified.
For working examples, refer
Server:
MovieCachedService.getActorInfo()
Client:
MovieCacheClient.findActor_etag_match()
MovieCacheClient.findActor_etag_unmatch()
When last modified date and entity tag are used together, the results seems to be like below:
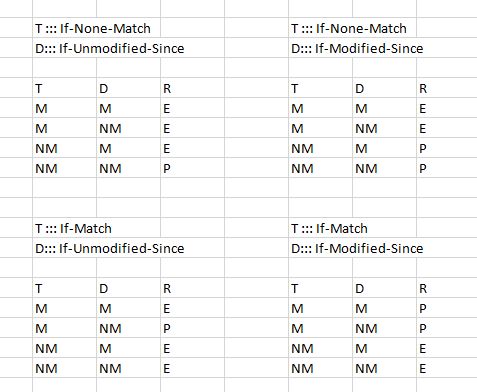
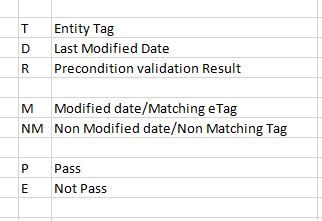
For working examples, refer
Server:
MovieCachedService.updateMovie()
Client:
MovieCacheClient.updateMovie_etag_NM_lastModified_NM()
MovieCacheClient.updateMovie_etag_M_lastModified_NM()
MovieCacheClient.updateMovie_etag_NM_lastModified_M()
MovieCacheClient.updateMovie_etag_M_lastModified_M()