Guide: A Simple Project - Alteruna/au-multiplayer-guide GitHub Wiki
- In this part, we will go through how to set up a project with Alteruna, how spawning works, and how to sync transforms between players.
Create a new 2D project in Unity (Unity 2020.3 or later) and follow the guides 00 Get Alteruna Multiplayer and 01 Identify Your Application.
More information and additional resources are available on the Alteruna Discord server.
- Create an empty GameObject in the scene.
- Add the
Multiplayer Component
to the object.


- Create an object to represent the player. In this example we will make a
2D Object > Sprites > Square
. - Add an
Avatar
component. - Add a
TransformSynchronizable2D
component.
To control the Player, we need to create a controller component and then add it to our Player object. In this example we will make a simple version of a player controller which looks like this:
public class PlayerController : CommunicationBridge
{
public float Speed = 10.0f;
public float RotationSpeed = 180.0f;
private SpriteRenderer _renderer;
public override void Possessed(bool isPossessor, User user)
{
enabled = isPossessor;
if (isPossessor)
{
_renderer = GetComponent<SpriteRenderer>();
// Set the color sprite representing me to be green
_renderer.color = Color.green;
}
}
// Only runs when possessed by me.
void Update()
{
// Get the horizontal and vertical axis.
float _translation = Input.GetAxis("Vertical") * Speed;
float _rotation = -Input.GetAxis("Horizontal") * RotationSpeed;
_translation *= Time.deltaTime;
_rotation *= Time.deltaTime;
transform.Translate(0, _translation, 0, Space.Self);
transform.Rotate(0, 0, _rotation);
}
}
To use the newly created Player object in our game, we must first save it as a prefab. Once we have a player prefab, we can hook it up to the Multiplayer Component to be automatically spawned when we or someone else joins the Room.
First, in the Multiplayer Component, change the Spawn Avatar
dropdown value to Spawn On Join
. This will open up more options.
Put the Player prefab that we made earlier in the Avatar Prefab to spawn
property.
Finally, create a new empty GameObject and place it somewhere in front of the camera. Select this GameObject in the AvatarSpawnLocation
property and new players will spawn at the same location.
Now, when we or someone else joins a Room, an instance of the Player prefab will be spawned and only accept input from the User it represents!
To test that our game works, add the RoomMenu
prefab into the scene so that we can join a room easily. The prefab is located in Alteruna > Prefabs > RoomMenu
Then, build and run the game! Now, if we start the game and join the same Room on two instances at the same time, we should see two of our Player objects but only be able to control the green one!
It should look something like this:
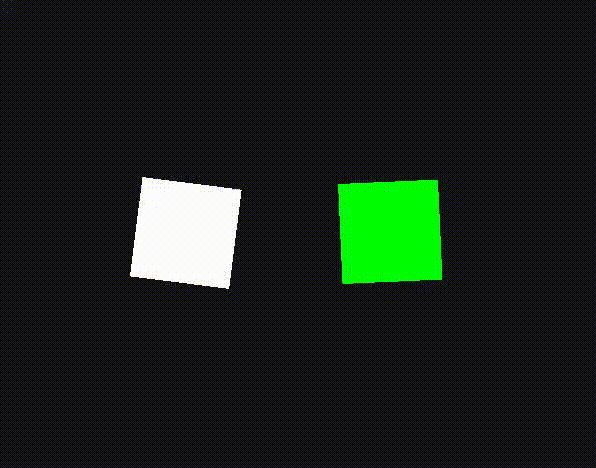